
iPhone开发【七】常用控件之表TableView
备注1、备注2,代码解释: 1)表中的每一行都拥有一个子视图,即一个UITableViewCell类的实例 2)对于一个拥有大量数据的表,如果UITableView为表中的每一行都分配一个UITableViewCell的实例,而不管该行是否正在显示,这无疑将带来大量内容开销。当然,UITableView并不是这样设计的。 3)只有当前显示的行才被分配UITableViewCell的实例,因滚动操作已离开屏幕的表视图单元(UITableViewCell的实例)将被放置在一个可以被重用的单元序列中。 如果系统运行比较缓慢,表视图就从该序列中删除这些单元,以释放存储空间; 只要有可用的存储空间,表视图就会重新获取这些单元,以便以后再次使用。 4)//当一个表单视图滚出屏幕时,另一个表单视图就会从另一边滚动到屏幕上,如果滚动到屏幕上的新行重新使用从屏幕上滚动下来的那些单元格中的某一个单元格,系统就会避免与不断创建和释放那些视图相关的开销。 5)为了使用此机制,需要一个标识符(identifier)来标示可重用的单元格 6)表第一次初始化时肯定没有可重用的表单元,所以需要初始分配: cell = [[UITableViewCell alloc]initWithStyle:UITableViewCellStyleDefault reuseIdentifier:myTableViewCellIdentifier]; reuseIdentifier参数即标示该表单元可重用的标示符
发布日期:2021-09-28 18:46:12
浏览次数:9
分类:技术文章
本文共 3917 字,大约阅读时间需要 13 分钟。
转载请注明出处,原文网址: 作者:张燕广
实现的功能:演示表TableView的使用方法
关键词:TableView
1、创建一个Single View Application工程,命名为:TableViewDemo,如下图
2、修改ViewController.xib,添加一个Table View控件,连接操作,如下
3、视图控制器ViewController,需要实现协议UITableViewDataSource、UITableViewDelegate中的必须实现的方法,
在工程目录依次展开Frameworks->UIKit.framework->Headers,然后打开UITableView.h,搜索找到协议UITableViewDataSource、UITableViewDelegate的定义,如下
4、修改ViewController.h,如下
- <span style="font-family:Microsoft YaHei;font-size:18px;">//
- // ViewController.h
- // TableViewDemo
- //
- // Created by Zhang Yanguang on 12-10-25.
- // Copyright (c) 2012年 MyCompanyName. All rights reserved.
- //
- #import <UIKit/UIKit.h>
- @interface ViewController : UIViewController
- @property(nonatomic,retain)NSMutableArray *apps;
- @end
- </span>
5、修改ViewController.m,如下
- <span style="font-family:Microsoft YaHei;font-size:18px;">//
- // ViewController.m
- // TableViewDemo
- //
- // Created by Zhang Yanguang on 12-10-25.
- // Copyright (c) 2012年 MyCompanyName. All rights reserved.
- //
- #import "ViewController.h"
- @interface ViewController ()
- @end
- @implementation ViewController
- @synthesize apps;
- - (void)viewDidLoad
- {
- [super viewDidLoad];
- // Do any additional setup after loading the view, typically from a nib.
- //加载数据
- [self loadData];
- }
- //模拟加载数据
- -(void)loadData{
- apps = [[NSMutableArray alloc]init];
- for(int i=0;i<8;i++){
- [apps insertObject:[NSString stringWithFormat:@"App-%d",(i+1)] atIndex:i];
- }
- }
- - (void)viewDidUnload
- {
- [super viewDidUnload];
- apps = nil;
- // Release any retained subviews of the main view.
- }
- -(void)dealloc{
- [super dealloc];
- [apps release];
- }
- - (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
- {
- return (interfaceOrientation != UIInterfaceOrientationPortraitUpsideDown);
- }
- #pragma mark table view datasource methods
- //可选实现的方法,默认为1
- - (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView;{
- return 1;
- }
- //必须实现的方法
- - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section{
- return [apps count];
- }
- //必须实现的方法
- - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath{
- //
- NSString *myTableViewCellIdentifier = @"myTableViewCellIdentifier";
- UITableViewCell *cell = [[UITableView alloc]dequeueReusableCellWithIdentifier:myTableViewCellIdentifier];//备注1
- if(cell==nil){
- cell = [[UITableViewCell alloc]initWithStyle:UITableViewCellStyleDefault reuseIdentifier:myTableViewCellIdentifier]; //备注2
- }
- NSString *imageName = [NSString stringWithFormat:@"%d",[indexPath row]+1];
- //cell.image = [UIImage imageNamed:imageName]; //这种用法已经废弃
- cell.imageView.image = [UIImage imageNamed:imageName];
- cell.textLabel.text = [apps objectAtIndex:[indexPath row]];
- cell.textLabel.textAlignment = UITextAlignmentLeft;
- cell.textLabel.font = [cell.textLabel.font fontWithSize:30];
- cell.accessoryType = UITableViewCellAccessoryDisclosureIndicator;
- return cell;
- }
- //可选实现的方法
- - (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section{
- return @"Header";
- }
- //可选实现的方法
- - (NSString *)tableView:(UITableView *)tableView titleForFooterInSection:(NSInteger)section{
- return @"Footer";
- }
- #pragma mark table view data delegate methods
- - (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath{
- return 50;
- }
- @end</span>
6、编译、运行,效果如下
转载地址:https://blog.csdn.net/h3c4lenovo/article/details/8307253 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
做的很好,不错不错
[***.243.131.199]2024年03月30日 05时40分09秒
关于作者
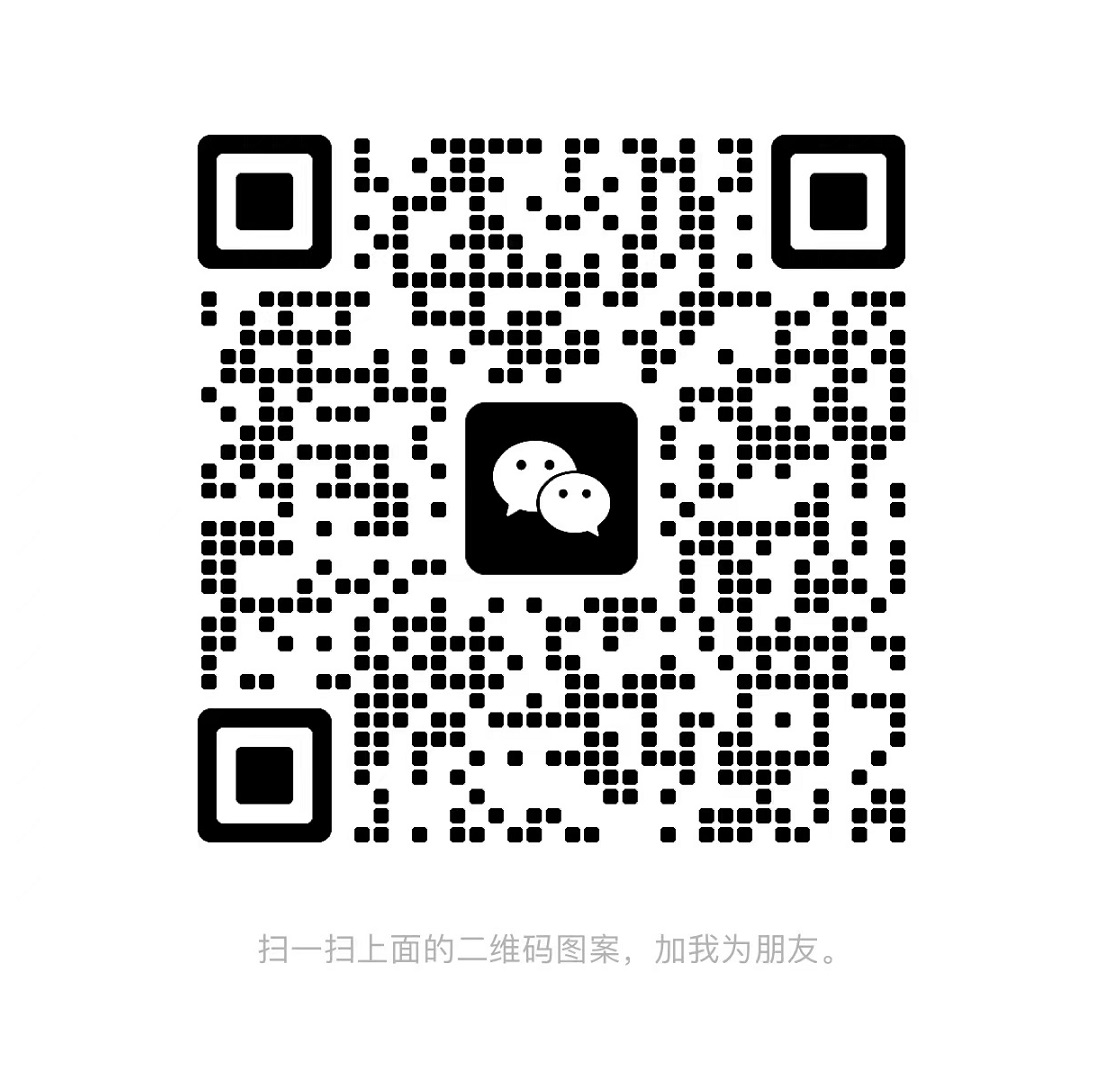
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
手把手教你实现Unity与Android的交互
2019-04-27
手把手教你使用Unity的Behavior Designer
2019-04-27
Unity3D摄像机裁剪——NGUI篇
2019-04-27
lua深拷贝一个table
2019-04-27
app运行提示Unable to Initialize Unity Engine
2019-04-27
spring boot 与 Ant Design of Vue 实现修改按钮(十七)
2019-04-27
spring boot 与 Ant Design of Vue 实现删除按钮(十八)
2019-04-27
spring boot 与 Ant Design of Vue 实现新增角色(二十)
2019-04-27
spring boot 与 Ant Design of Vue 实现修改角色(二十一)
2019-04-27
spring boot 与 Ant Design of Vue 实现删除角色(补二十一)
2019-04-27
spring boot 与 Ant Design of Vue 实现左侧组织树(二十三)
2019-04-27
spring boot 与 Ant Design of Vue 实现新增组织(二十四)
2019-04-27
spring boot 与 Ant Design of Vue 实现修改组织(二十五)
2019-04-27
spring boot 与 Ant Design of Vue 实现删除组织(二十六)
2019-04-27
spring boot 与 Ant Design of Vue 实现新增用户(二十八)
2019-04-27
spring boot 与 Ant Design of Vue 实现修改用户(二十九)
2019-04-27
spring boot 与 Ant Design of Vue 实现删除用户(三十)
2019-04-27