
自己对Handler的一些理解
发布日期:2021-10-07 06:13:00
浏览次数:1
分类:技术文章
本文共 10959 字,大约阅读时间需要 36 分钟。
Android的UI操作是线程不安全的,如果在UI线程中进行耗时操作,会导致ANR(Application Not Responding ),Android平台只允许在UI线程里修改UI组件,那么既要在改变UI又不能在UI线程中进行耗时操作就需要借助于Handler。
Handler 的底层由MessageQueue(内部采用单链表的数据结构来存储消息) 和Looper进行支撑` MessageQueue采用先进先出的方式来管理Message 下边是MessagQueue的next的实现Message next() {
// Return here if the message loop has already quit and been disposed.(直接返回如果message loop 已经停止并且已经进行了处理) // This can happen if the application tries to restart a looper after quit(一般发生在程序停止并尝试重新开始looper) // which is not supported. //没有被用过的 final long ptr = mPtr; if (ptr == 0) { return null; }int pendingIdleHandlerCount = -1; // -1 only during first iteration int nextPollTimeoutMillis = 0; //通过这个死循环不断的从Queue中取消息 for (;;) { //如果取出消息超时,则进行刷新 if (nextPollTimeoutMillis != 0) { Binder.flushPendingCommands(); } nativePollOnce(ptr, nextPollTimeoutMillis); synchronized (this) { // Try to retrieve the next message. Return if found.(取出下一个消息) final long now = SystemClock.uptimeMillis(); Message prevMsg = null; Message msg = mMessages; //当msg不为空,msg的target(Handler)为空时 if (msg != null && msg.target == null) { // Stalled by a barrier. Find the next asynchronous message in the queue. do { prevMsg = msg; msg = msg.next; } while (msg != null && !msg.isAsynchronous()); } if (msg != null) { //下一条消息还未准备好 if (now < msg.when) { // Next message is not ready. Set a timeout to wake up when it is ready. nextPollTimeoutMillis = (int) Math.min(msg.when - now, Integer.MAX_VALUE); } //取出下一个消息,并设置为“未阻塞”状态 else { // Got a message. mBlocked = false; if (prevMsg != null) { prevMsg.next = msg.next; } else { mMessages = msg.next; } msg.next = null; if (DEBUG) Log.v(TAG, "Returning message: " + msg); //将消息标记为使用 msg.markInUse(); return msg; } } else { // No more messages. nextPollTimeoutMillis = -1; } // Process the quit message now that all pending messages have been handled. if (mQuitting) { dispose(); return null; } // If first time idle, then get the number of idlers to run. // Idle handles only run if the queue is empty or if the first message // in the queue (possibly a barrier) is due to be handled in the future. if (pendingIdleHandlerCount < 0 && (mMessages == null || now < mMessages.when)) { pendingIdleHandlerCount = mIdleHandlers.size(); } if (pendingIdleHandlerCount <= 0) { // No idle handlers to run. Loop and wait some more. mBlocked = true; continue; } if (mPendingIdleHandlers == null) { mPendingIdleHandlers = new IdleHandler[Math.max(pendingIdleHandlerCount, 4)]; } mPendingIdleHandlers = mIdleHandlers.toArray(mPendingIdleHandlers); } // Run the idle handlers. // We only ever reach this code block during the first iteration. for (int i = 0; i < pendingIdleHandlerCount; i++) { final IdleHandler idler = mPendingIdleHandlers[i]; mPendingIdleHandlers[i] = null; // release the reference to the handler boolean keep = false; try { keep = idler.queueIdle(); } catch (Throwable t) { Log.wtf(TAG, "IdleHandler threw exception", t); } if (!keep) { synchronized (this) { mIdleHandlers.remove(idler); } } } // Reset the idle handler count to 0 so we do not run them again. pendingIdleHandlerCount = 0; // While calling an idle handler, a new message could have been delivered // so go back and look again for a pending message without waiting. nextPollTimeoutMillis = 0; }}`
上边是不断从Queue中取出消息的源码
这里是将消息插入到队列当中boolean enqueueMessage(Message msg, long when) { if (msg.target == null) { throw new IllegalArgumentException("Message must have a target."); } if (msg.isInUse()) { throw new IllegalStateException(msg + " This message is already in use."); } synchronized (this) { if (mQuitting) { IllegalStateException e = new IllegalStateException( msg.target + " sending message to a Handler on a dead thread"); Log.w(TAG, e.getMessage(), e); msg.recycle(); return false; } msg.markInUse(); msg.when = when; Message p = mMessages; boolean needWake; //如果队列阻塞,进行wakeup if (p == null || when == 0 || when < p.when) { // New head, wake up the event queue if blocked. msg.next = p; mMessages = msg; needWake = mBlocked; } else { // Inserted within the middle of the queue. Usually we don't have to wake // up the event queue unless there is a barrier at the head of the queue // and the message is the earliest asynchronous message in the queue. needWake = mBlocked && p.target == null && msg.isAsynchronous(); Message prev; //将Message插入到队列 for (;;) { prev = p; p = p.next; if (p == null || when < p.when) { break; } if (needWake && p.isAsynchronous()) { needWake = false; } } msg.next = p; // invariant: p == prev.next prev.next = msg; } // We can assume mPtr != 0 because mQuitting is false. if (needWake) { nativeWake(mPtr); } } return true; }
Looper的构造方法是是private,因此不能通过new来创建一个Looper。通过prepare方法进行创建。在Looper的构造方法中创建一个Message,并且保存当前Thread对象
private Looper(boolean quitAllowed) { mQueue = new MessageQueue(quitAllowed); mThread = Thread.currentThread(); }
Looper的源码中有这样的一个ThreadLocal。
ThreadLocal从名字可以看出局部线程,她是一个线程内部的数据存储类,通过ThreadLocal可以在指定线程中存储数据,因为Looper的作用于为当前所在线程,所以用ThreadLocal来存放Looper再合适不过了。static final ThreadLocalsThreadLocal = new ThreadLocal ();
通过preppare()方法为当前线程创建一个Looper,如果当前线程已经存在了Looper,那么抛出异常.
public static void prepare() { prepare(true); } private static void prepare(boolean quitAllowed) { if (sThreadLocal.get() != null) { throw new RuntimeException("Only one Looper may be created per thread"); } sThreadLocal.set(new Looper(quitAllowed)); }
loop是Looper中最主要的方法。来看下loop的源码
public static void loop() { //获取当前线程的Looper final Looper me = myLooper(); if (me == null) { throw new RuntimeException("No Looper; Looper.prepare() wasn't called on this thread."); } //获取当前线程的MessageQueue final MessageQueue queue = me.mQueue; // Make sure the identity of this thread is that of the local process, // and keep track of what that identity token actually is. Binder.clearCallingIdentity(); final long ident = Binder.clearCallingIdentity(); //用死循环从Message中取出消息 for (;;) { Message msg = queue.next(); // might block //当消息为空时,跳出这个死循环 if (msg == null) { // No message indicates that the message queue is quitting. return; } // This must be in a local variable, in case a UI event sets the logger Printer logging = me.mLogging; if (logging != null) { logging.println(">>>>> Dispatching to " + msg.target + " " + msg.callback + ": " + msg.what); } //Handler进行事件的分发 msg.target.dispatchMessage(msg); if (logging != null) { logging.println("<<<<< Finished to " + msg.target + " " + msg.callback); } // Make sure that during the course of dispatching the // identity of the thread wasn't corrupted. final long newIdent = Binder.clearCallingIdentity(); if (ident != newIdent) { Log.wtf(TAG, "Thread identity changed from 0x" + Long.toHexString(ident) + " to 0x" + Long.toHexString(newIdent) + " while dispatching to " + msg.target.getClass().getName() + " " + msg.callback + " what=" + msg.what); } msg.recycleUnchecked(); } }
Handler的发送消息,最后通过sendMessageAtTime将Message放到MessageQueue中
public final boolean sendMessage(Message msg) { return sendMessageDelayed(msg, 0); } public final boolean sendEmptyMessage(int what) { return sendEmptyMessageDelayed(what, 0); } public final boolean sendEmptyMessageDelayed(int what, long delayMillis) { Message msg = Message.obtain(); msg.what = what; return sendMessageDelayed(msg, delayMillis); } public final boolean sendEmptyMessageAtTime(int what, long uptimeMillis) { Message msg = Message.obtain(); msg.what = what; return sendMessageAtTime(msg, uptimeMillis); } public final boolean sendMessageDelayed(Message msg, long delayMillis) { if (delayMillis < 0) { delayMillis = 0; } return sendMessageAtTime(msg, SystemClock.uptimeMillis() + delayMillis); } public boolean sendMessageAtTime(Message msg, long uptimeMillis) { MessageQueue queue = mQueue; if (queue == null) { RuntimeException e = new RuntimeException( this + " sendMessageAtTime() called with no mQueue"); Log.w("Looper", e.getMessage(), e); return false; } return enqueueMessage(queue, msg, uptimeMillis); }
Handler的dispatchMessage,callback是一个Runnable对象
public void dispatchMessage(Message msg) { if (msg.callback != null) { handleCallback(msg); } else { if (mCallback != null) { if (mCallback.handleMessage(msg)) { return; } } handleMessage(msg); } } //handlerMessage是一个空方法,在用Handler时重写该方法,来处理消息 public void handleMessage(Message msg) { }
转载地址:https://blog.csdn.net/mengmengkenanjun/article/details/51291459 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
很好
[***.229.124.182]2024年05月06日 17时13分44秒
关于作者
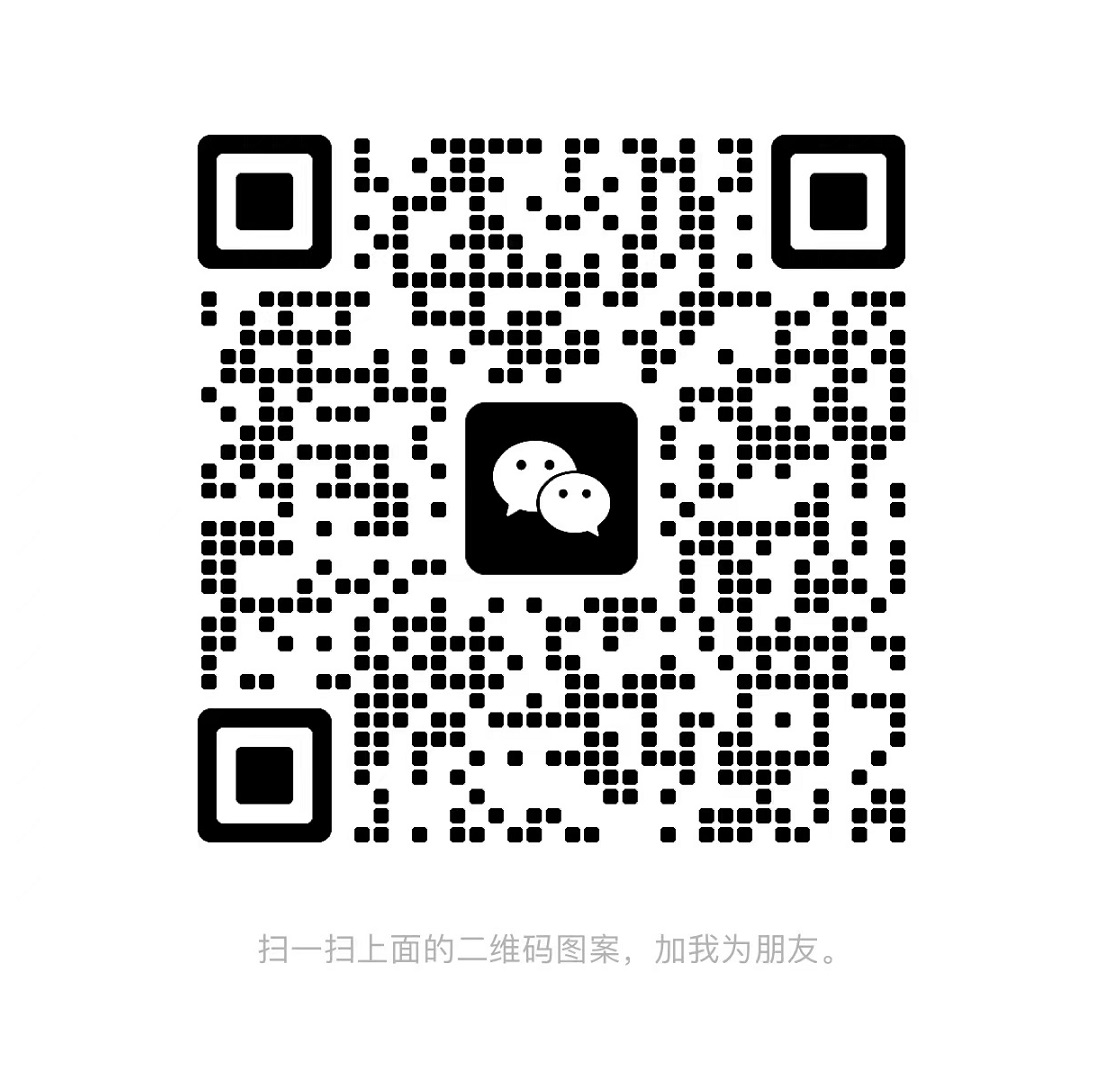
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
由DFS到访问者模式
2019-05-01
字节的面试题到底有多难?大厂为何都注重算法?
2019-05-01
阿里大师呕心整理出来的分布式事务至尊级学习笔记!干货满满!
2019-05-01
膜拜!这份技术点拉满的Redis深度历险笔记,价值百万!
2019-05-01
RabbitMQ消息队列(七):适用于云计算集群的远程调用(RPC)
2019-05-01
xtrabackup备份之增量备份(二)
2019-05-01
《视频直播技术详解》系列:(6)编码和封装
2019-05-01
类函数重写、重载、覆盖示例
2019-05-01
五种主要多核并行编程方法分析与比较
2019-05-01
GB28181计算注册登陆时的鉴权信息
2019-05-01
人工智能为什么这么火?看看安防江湖30年血战就知道了
2019-05-01
“中国已建成世界上最大的视频监控网”背后的问题
2019-05-01
一个ps解复用H264和H264打包ps的动态库及demo
2019-05-01
“前端智能为安防产生新的数据价值”
2019-05-01
异或^ 的几个作用
2019-05-01
nanomsg编译与测试
2019-05-01
(2)CMake入门笔记--CMake官网教程
2019-05-01
(3)CMake入门笔记--CMake官网教程
2019-05-01
(4)CMake入门笔记--CMake官网教程
2019-05-01
(XWZ)的python学习笔记Ⅰ
2019-05-01