
本文共 4091 字,大约阅读时间需要 13 分钟。
1 一行赋值序列和元组In [11]: l = ['a', 'b']
In [12]: x,y = l
In [13]: x
Out[13]: 'a'
In [14]: y
Out[14]: 'b'In [15]: t = (1,2)
In [16]: m,n =t
In [17]: m
Out[17]: 1
In [18]: n
Out[18]: 2
2 交换变量In [6]: m = 3
In [7]: n = 4
In [8]: m,n = n,m
In [9]: m
Out[9]: 4
In [10]: n
Out[10]: 3
3 语句在行内,if 的三目运算In [19]: print 'Hello' if True else 'World'
HelloIn [20]: a = ' ni'
In [21]: b = a.strip() if a.strip() else None
In [22]: b
Out[22]: 'ni'
In [23]: a = ' '
In [24]: b = a.strip() if a.strip() else None
In [25]: b
4 连接--相同类型的数据可以连接拼接In [27]: s = 'my name is: '
In [28]: msg = s + 'Andy'
In [29]: msg
Out[29]: 'my name is: Andy'In [30]: l1 = ['Apple', 'orange']
In [31]: l2 = ['Banana', 'Grape']
In [32]: l1 + l2
Out[32]: ['Apple', 'orange', 'Banana', 'Grape']
5 数字技巧
整数和浮点除In [33]: 5 /2
Out[33]: 2
In [34]: 5.0 /2
Out[34]: 2.5
整数和浮点取余数In [36]: 5 %2
Out[36]: 1
In [37]: 5.00 %2
Out[37]: 1.0
6 数字比较和少语言可以如此用法In [43]: if x 2:
....: print x
....:
3
等于In [44]: if 2
....: print x
....:
3
7 同时迭代两个列表(序列)In [46]: l1
Out[46]: ['Apple', 'orange']
In [47]: l2
Out[47]: ['Banana', 'Grape']
In [48]: for item1, item2 in zip(l1, l2):
....: print item1 + ' vs ' + item2
....:
Apple vs Banana
orange vs Grape
8 带索引的列表迭代In [51]: for index, item in enumerate(l1):
....: print index, item
....:
0 Apple
1 orangeIn [52]: for index, item in enumerate(l1):
....: print index +1, item
....:
1 Apple
2 orange
9 生成列表(列表推到式)In [53]: numbers = [1,2,3,4,5,6,7,8]
In [54]: even = []
In [55]: for number in numbers:
....: if number%2 == 0:
....: even.append(number)
....:
In [56]: even
Out[56]: [2, 4, 6, 8]
等价于一行语句In [59]: even = [ number for number in numbers if number %2 == 0]
In [60]: even
Out[60]: [2, 4, 6, 8]
10 字典推导(只使用 python 2.7版本 不适合以下版本)names = ['andy', 'jack', 'tom', 'john']
d = {key: value for value, key in enumerate(names)}
>>> d
{'john': 3, 'andy': 0, 'jack': 1, 'tom': 2}
11 列表转字符串In [64]: l1
Out[64]: ['Apple', 'orange']
In [66]: ",".join(l1)
Out[66]: 'Apple,orange'
12 字符串转列表In [67]: ids = "4,57,9,22,31"
In [68]: ids.split(',')
Out[68]: ['4', '57', '9', '22', '31']
13获取列表的子集(切片)In [2]: x = [1,2,3,4,5,6]
获取前三个In [3]: x[:3]
Out[3]: [1, 2, 3]
获取index=0 到 index=4的In [4]: x[1:5]
Out[4]: [2, 3, 4, 5]
获取后面3个In [5]: x[-3:]
Out[5]: [4, 5, 6]
获取奇数(从第一个到最后一个,步长为2)In [6]: x[::2]
Out[6]: [1, 3, 5]
获取偶数(从第一个到最后一个,步长为2)In [7]: x[1::2]
Out[7]: [2, 4, 6]
倒序In [8]: x[::-1]
Out[8]: [6, 5, 4, 3, 2, 1]
14 合并列表zip
n个列表通过zip函数后,返回一个n个元素组成的元组形式的列表(列表长度为最短的那个列表)In [23]: l1
Out[23]: [1, 2, 3, 4]
In [24]: l2
Out[24]: [6, 7, 8, 9]
In [25]: zip(l1,l2)
Out[25]: [(1, 6), (2, 7), (3, 8), (4, 9)]In [17]: [x+y for x,y in zip(l1,l2)]
Out[17]: [7, 9, 11, 13]
15 序列的映射map
map(function, sequence[, sequence, ...]) -> list
多个序列通过function返回后返回一个新的列表,最好是序列的长度要一致(不一致的元素补None)
function 可以为None,那么map功能就和zip一样了In [27]: map(None,l1,l2)
Out[27]: [(1, 6), (2, 7), (3, 8), (4, 9)]In [28]: map(lambda x,y:x +y , l1,l2)
Out[28]: [7, 9, 11, 13]
16 插入一条常见知识,程序中出现中文执行程序报错
syntaxError: Non-ASCII character '\xe4' in file test.py on line 7
解决:#!/usr/bin/env python
#encoding=utf-8
import sys
reload(sys)
sys.setdefaultencoding('utf-8')
以上格式肯定是不会出错,注意#encoding=utf-8 一定要在第二行,在其他行不起作用
17 可以定义一个python执行错误的函数,每次执行错误,调用即可
#python 参数输入错误的提示输入函数def exec_error(msg=None):
print "\033[1;31;40mUsage: python xxx.py {0|1|2|3|all}\033[0m \n\t \
0:None level project, 1:level 1 project, 2:level 2 project, 3:level 3 project, all:all project"
sys.exit()
18 通过ip地址翻译的主机名的函数
#通过ip或者hostname解析获得hostname,ipdef resolve_host(s):
l = s.split('.')
if len(l) > 4:
return "-"
if len(l) == 4:
ip = s
try:
hostname = socket.gethostbyaddr(s)[0]
except Exception,e:
#print "could't look up name by ipaddress:",e
#sys.exit(1)
return '-'
else:
return '-'
return hostname
19 filter函数
python自带
常用过滤列表
用法: filter(函数,列表)
递归传递列表的值到函数中函数返回值为true则作为新列表的元素In [7]: l = [1,2,3,4,5]
In [8]: filter(lambda x : x >3, l)
Out[8]: [4, 5]
其实等价于 和生成序列的常用方法效果一样[i for i in l if i > 3]
实践中的用途:In [19]: task_id =4
In [20]: tasks = [{"id":3, "title":"three"}, {"id":4, "title":"four"}, {"id":5,"title":"five"}]
In [21]: filter(lambda i: i["id"] == task_id ,tasks)
Out[21]: [{'id': 4, 'title': 'four'}]
转载地址:https://blog.csdn.net/weixin_39934257/article/details/110539577 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关于作者
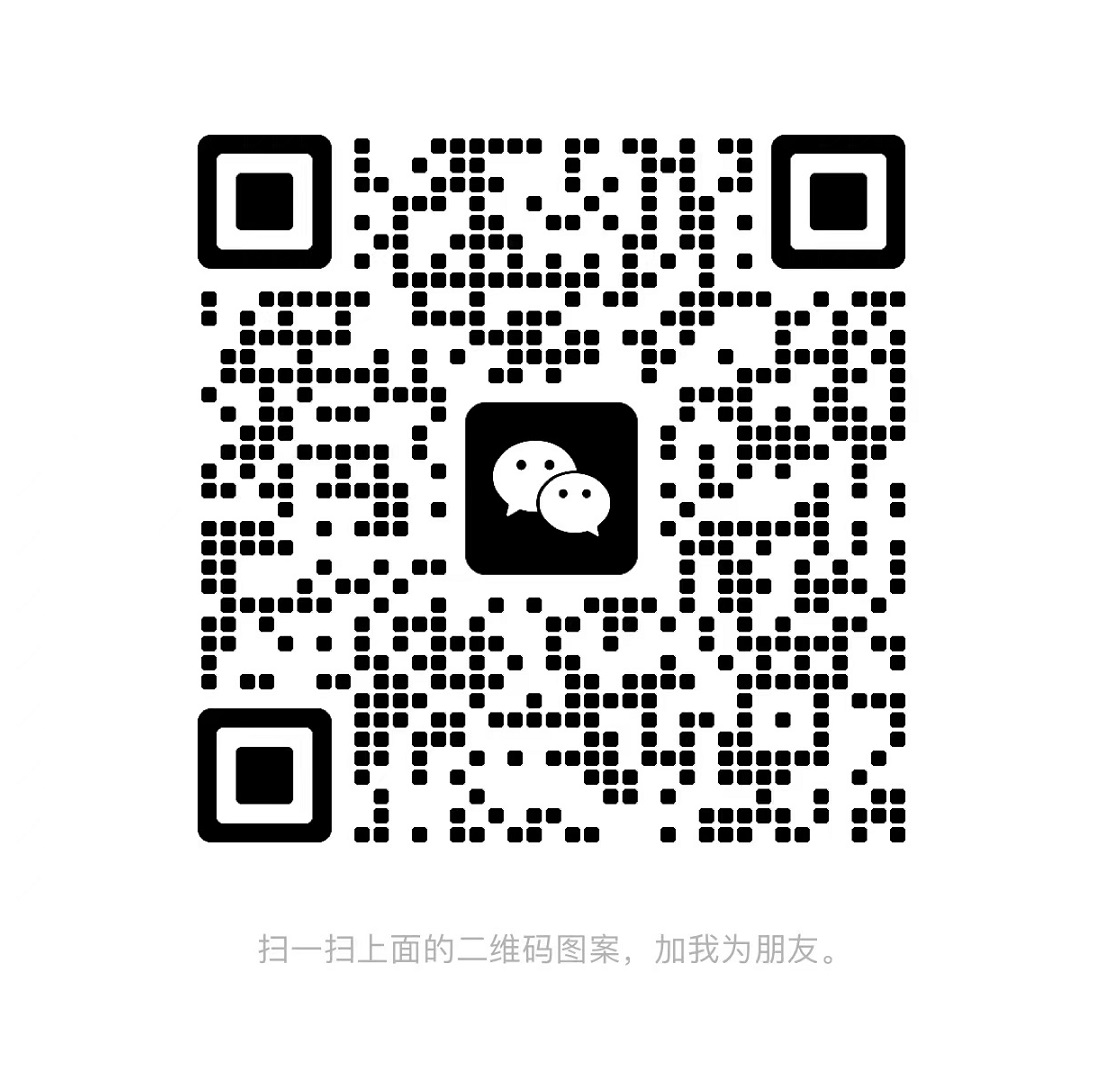