
本文共 3786 字,大约阅读时间需要 12 分钟。
import java.security.InvalidKeyException;
import java.security.Key;
import java.security.NoSuchAlgorithmException;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.KeyGenerator;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import org.apache.commons.codec.binary.Base64;
import org.apache.commons.codec.digest.DigestUtils;
/**
* Created by Lb on 2017/9/10.
*/
public class AESCoder {
//密钥算法
public static final String KEY_ALGORITHM = "AES";
//工作模式,填充模式
public static final String CIPHER_ALGORITHM = "AES/ECB/PKCS5Padding";
/**
* 转换密钥
*
* @param key 二进制密钥
* @return Key密钥
*/
private static Key toKey(byte[] key) {
SecretKey secretKey = new SecretKeySpec(key, KEY_ALGORITHM);
return secretKey;
}
/**
* 解密
*/
public static byte[] decrpyt(byte[] data, byte[] key)
throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidKeyException, BadPaddingException, IllegalBlockSizeException {
//还原密钥
Key k = toKey(key);
//实例化
Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);
//初始化
cipher.init(Cipher.DECRYPT_MODE, k);
//执行操作
return cipher.doFinal(data);
}
/**
* 加密
*/
public static byte[] encrypt(byte[] data, byte[] key)
throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidKeyException, BadPaddingException, IllegalBlockSizeException {
//还原密钥
Key k = toKey(key);
//实例化
Cipher cipher = Cipher.getInstance(CIPHER_ALGORITHM);
//初始化
cipher.init(Cipher.ENCRYPT_MODE, k);
//执行操作
return cipher.doFinal(data);
}
/**
* 生成密钥
*/
public static byte[] initKey() throws NoSuchAlgorithmException {
//实例化
KeyGenerator keyGenerator = KeyGenerator.getInstance(KEY_ALGORITHM);
//AES 要求密钥长度为128位、192位或256位
keyGenerator.init(128);
//生成秘密密钥
SecretKey secretKey = keyGenerator.generateKey();
//获取密钥的二进制编码形式
return secretKey.getEncoded();
}
/**
* 初始化密钥
*
* @return Base64编码密钥
*/
public static String initKeyString() throws NoSuchAlgorithmException {
return Base64.encodeBase64String(initKey());
}
/**
* 获取密钥
*/
public static byte[] getKey(String key) {
return Base64.decodeBase64(key);
}
/**
* 解密
*
* @param data 待解密数据
* @param key 密钥
* @return byte[] 解密数据
*/
public static byte[] decrypt(byte[] data, String key)
throws IllegalBlockSizeException, InvalidKeyException, BadPaddingException, NoSuchAlgorithmException, NoSuchPaddingException {
return decrpyt(data, getKey(key));
}
/**
* 加密
*
* @param data 待加密数据
* @param key 密钥
* @return byte[]加密数据
*/
public static byte[] encrypt(byte[] data, String key)
throws IllegalBlockSizeException, InvalidKeyException, BadPaddingException, NoSuchAlgorithmException, NoSuchPaddingException {
return encrypt(data, getKey(key));
}
/**
* 摘要处理
*
* @param data 待摘要数据
* @return 摘要字符串
*/
public static String shaHex(byte[] data) {
return DigestUtils.md5Hex(data);
}
/**
* 验证
*
* @param data 待摘要数据
* @param messageDigest 摘要字符串
* @return 验证结果
*/
public static boolean vailidate(byte[] data, String messageDigest) {
return messageDigest.equals(shaHex(data));
}
public static void main(String[] args)
throws NoSuchAlgorithmException, IllegalBlockSizeException, InvalidKeyException, BadPaddingException, NoSuchPaddingException {
String inputStr = "AES18803696960http://www.baidu;com";
byte[] inputData = inputStr.getBytes();
System.out.println("原文:" + inputStr);
//初始化密钥
byte[] key = AESCoder.initKey();
System.out.println("密钥:" + Base64.encodeBase64String(key));
//加密
inputData = AESCoder.encrypt(inputData, key);
System.out.println("加密后:" + Base64.encodeBase64String(inputData));
//解密
byte[] outputData = AESCoder.decrpyt(inputData, key);
String outputStr = new String(outputData);
System.out.println("解密后:" + outputStr);
System.out.println("校验签名:" + AESCoder.vailidate(inputData, AESCoder.shaHex(inputData)));
}
}
转载地址:https://blog.csdn.net/weixin_31990755/article/details/114585823 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关于作者
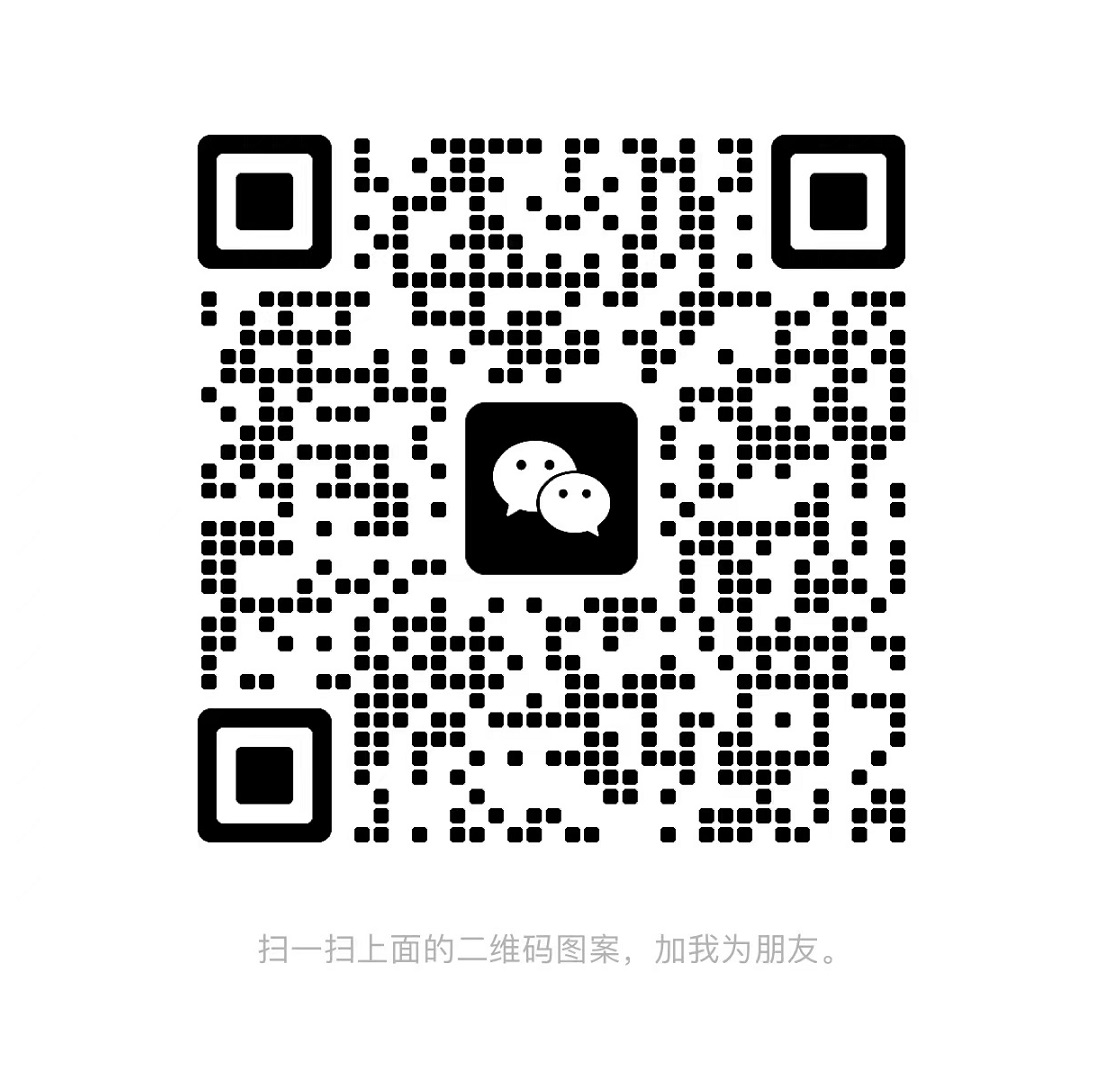