
本文共 7629 字,大约阅读时间需要 25 分钟。
通过示例了解可用的Java operators , precedence order并了解其用法。 我们还将尝试了解何时使用哪个运算符以及期望得到什么。
1. What Is an Operator?
运算符是对一个,两个或三个操作数执行特定类型的运算并产生结果的符号 。
运算符及其操作数的类型确定对操作数执行的运算的类型以及产生的结果的类型。
1.1. Classification of Java Operators
Java中的运算符可以根据两个条件进行分类:
Number of operands共有三种类型的运算符。
根据操作数的数量,运算符称为一元,二进制或三元运算符。 如果一个运算符采用一个操作数,则称为一unary
operator ; 如果需要两个操作数,则称为binary
operator ; 如果需要三个操作数,则称为ternary
operator 。
它们执行的操作类型 –运算符称为arithmetic
operator , relational operator
, logical operator或bitwise operator ,具体取决于bitwise operator在其操作数上执行的类型。
2. Assignment Operator (=)
赋值运算符(=)用于为变量赋值。
它是一个二进制运算符。 它需要两个操作数。
右侧操作数的值已分配给左侧操作数。
左侧操作数必须是变量。
//26 is the right-hand operand.
//counter is the left-hand operand, which is a variable of type int.
int counter = 26;
Java确保赋值运算符的右侧操作数的值与左侧操作数的数据类型兼容。 否则,将发生compile-time
error 。 如果是引用变量,则如果右侧操作数表示的对象的赋值与作为左侧操作数的引用变量不兼容,则可以编译源代码并获得运行时ClassCastException错误。
3. Arithmetic Operators
像( + (加号), – (减号), *
(乘数), / (除法))之类的运算符在Java中称为算术运算符。
它只能与数字类型的操作数一起使用。 这意味着算术运算符的两个操作数都必须是byte ,
short , char ,
int , long ,
float和double 。
这些运算符不能具有boolean基本类型和引用类型的操作数。
int sum = 10 + 20;
int difference = 50 - 20;
long area = 20l * 30l;
int percentage = 20 / 100;
3.1. Unary Arithmetic Operators
Operator
Description
'+'
Unary plus operator; indicates positive value (numbers are
positive without this, however)
'-'
Unary minus operator; negates an expression value
'++'
Increment operator; increments a value by 1
'--'
Decrement operator; decrements a value by 1
'!'
Logical complement operator; inverts the value of a boolean
3.2. Binary Arithmetic Operators
Operator
Description
'+'
Addition – Adds values on either side of the operator
'-'
Subtraction – Subtracts right hand operand from left hand
operand
'*'
Multiplication – Multiplies values on either side of the
operator
'/'
Division – Divides left hand operand by right hand operand
'%'
Modulus – Divides left hand operand by right hand operand
and returns remainder
4. String Concatenation Operator (+)
Java中的'+'运算符已重载。 如果一个运算overloaded operator用于执行多个功能,则称该运算符为overloaded operator 。
4.1. Concatenate two strings
到目前为止,您已经看到它用作算术加法运算符来将两个数字相加。 它也可以用来concatenate two
strings 。
String str1 = "Hello";
String str2 = " World";
String str3 = str1 + str2; // Assigns "Hello World" to str3
3.2. Concatenate primitive to string
字符串连接运算符还用于将原语和参考数据类型值连接到字符串。
int num = 26;
String str1 = "Alphabets";
String str2 = num + str1; // Assigns "26Alphabets" to str2
4.2. Concatenate null
如果引用变量包含“空”引用,则串联运算符将使用字符串“空”。
String str1 = "I am ";
String str2 = null;
String str3 = str1 + str2; // Assigns "I am null" to str3
5. Relational Operators
所有关系运算符都是二进制运算符。
他们采用两个操作数。
关系运算符产生的结果始终是布尔值true或false 。
下面让我们看看Java中所有可用的关系运算符。
Operator
Description
'=='
Equals to – Checks if the values of two operands are equal
or not, if yes then condition becomes true.
'!='
Not equals to – Checks if the values of two operands are
equal or not, if values are not equal then condition becomes true.
'>'
Greater than – Checks if the value of left operand is
greater than the value of right operand, if yes then condition becomes true.
'
Less than – Checks if the value of left operand is less
than the value of right operand, if yes then condition becomes true.
'>='
Greater than or equals to – Checks if the value of left
operand is greater than or equal to the value of right operand, if yes then
condition becomes true.
'<='
Less than or equals to – Checks if the value of left
operand is less than or equal to the value of right operand, if yes then
condition becomes true.
int result = 20;
if( result > 10) { //true
//some operation
}
boolean isEqual = ( 10 == 20 ); //false
6. Boolean Logical Operators
所有布尔逻辑运算符只能与布尔操作数一起使用。
它们主要用于控制语句中以比较两个(或多个)条件。
Operator
Description
'!'
returns true if the operand is false, and false if the operand is true.
'&&'
returns true if both operands are true. If either operand is false, it
returns false.
'&'
returns true if both operands are true. If either operand is false, it
returns false.
'||'
returns true if either operand is true. If both operands are false, it
returns false.
'|'
returns true if either operand is true. If both operands are false, it
returns false.
'^'
it returns true if one of the operands is true, but not both. If both
operands are the same, it returns false.
'&=;'
if both operands evaluate to true, &= returns true. Otherwise, it
returns false.
'|='
if either operand evaluates to true, != returns true. Otherwise, it returns
false.
'^='
if both operands evaluate to different values, that is, one of the operands
is true but not both, ^= returns true. Otherwise, it returns false.
int result = 20;
if( result > 10 && result < 30) {
//some operation
}
if( result > 10 || result < 30) {
//some operation
}
logical AND operator
(&)的工作方式与逻辑短路AND运算符(&&)相同,不同之处在于。
逻辑AND运算符(&)会计算其右手操作数,即使其左手操作数的计算结果为false。
logical OR
operator工作方式与逻辑短路或运算符相同,不同之处在于。
逻辑“或”运算符将评估其右侧操作数,即使其左侧操作数的评估结果为true。
7. Bitwise Operators
按位运算符manipulates individual bits其操作数的manipulates individual bits 。
Java定义了几个按位运算符,它们可以应用于整数类型long,int,short,char和byte。
Operator
Description
'&'
Binary AND Operator copies a bit to the result if it exists
in both operands.
'|'
Binary OR Operator copies a bit if it exists in either
operand.
'^'
Binary XOR Operator copies the bit if it is set in one
operand but not both.
'~'
Binary Ones Complement Operator is unary and has the effect
of ‘flipping’ bits.
<<
Binary Left Shift Operator. The left operands value is
moved left by the number of bits specified by the right operand.
>>
Binary Right Shift Operator. The left operands value is
moved right by the number of bits specified by the right operand.
>>>
Shift right zero fill operator. The left operands value is
moved right by the number of bits specified by the right operand and shifted
values are filled up with zeros.
8. Ternary Operator (? 🙂
Java有一个条件运算符。 它被称为三元运算符,因为它需要three operands 。
“?”和“:”这两个符号构成三元运算符。
如果boolean-expression的计算结果为true,则计算为true。 否则,它将评估错误表达式。
8.1. Syntax
boolean-expression ? true-expression : false-expression
8.2. Ternary Operator Example
int number1 = 40;
int number2 = 20;
int biggerNumber = (number1 > number2) ? number1 : number2;
//Compares both numbers and return which one is bigger
9. Java Operator Precedence Table
Java具有明确定义的规则,用于指定当表达式具有多个运算符时对表达式中的运算符求值的顺序。 例如,乘法和除法的优先级高于加法和减法。
优先规则可以由显式括号覆盖。
当两个运算符共享一个操作数时,优先级较高的运算符优先。 例如,将1 + 2 * 3视为1 + (2 * 3)因为乘法的优先级高于加法。
在上面的表达式中,如果要首先添加值,则使用显式括号,例如– (1 + 2) * 3 。
Precedence
Operator
Type
Associativity
15
()
[]
·
Parentheses
Array subscript
Member selection
Left to Right
14
++
—
Unary post-increment
Unary post-decrement
Right to left
13
++
—
+
–
!
~
( type )
Unary pre-increment
Unary pre-decrement
Unary plus
Unary
minus
Unary logical negation
Unary bitwise complement
Unary
type cast
Right to left
12
*
/
%
Multiplication
Division
Modulus
Left to right
11
+
–
Addition
Subtraction
Left to right
10
<<
>>
>>>
Bitwise left shift
Bitwise right shift with sign extension
Bitwise
right shift with zero extension
Left to right
9
<
<=
>
>=
instanceof
Relational less than
Relational less than or equal
Relational
greater than
Relational greater than or equal
Type comparison
(objects only)
Left to right
8
==
!=
Relational is equal to
Relational is not equal to
Left to right
7
&
Bitwise AND
Left to right
6
^
Bitwise exclusive OR
Left to right
5
|
Bitwise inclusive OR
Left to right
4
&&
Logical AND
Left to right
3
||
Logical OR
Left to right
2
? :
Ternary conditional
Right to left
1
=
+=
-=
*=
/=
%=
Assignment
Addition assignment
Subtraction
assignment
Multiplication assignment
Division
assignment
Modulus assignment
Right to left
这就是operators in java的operators in java 。
Read More:
转载地址:https://blog.csdn.net/weixin_32467421/article/details/114202432 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关于作者
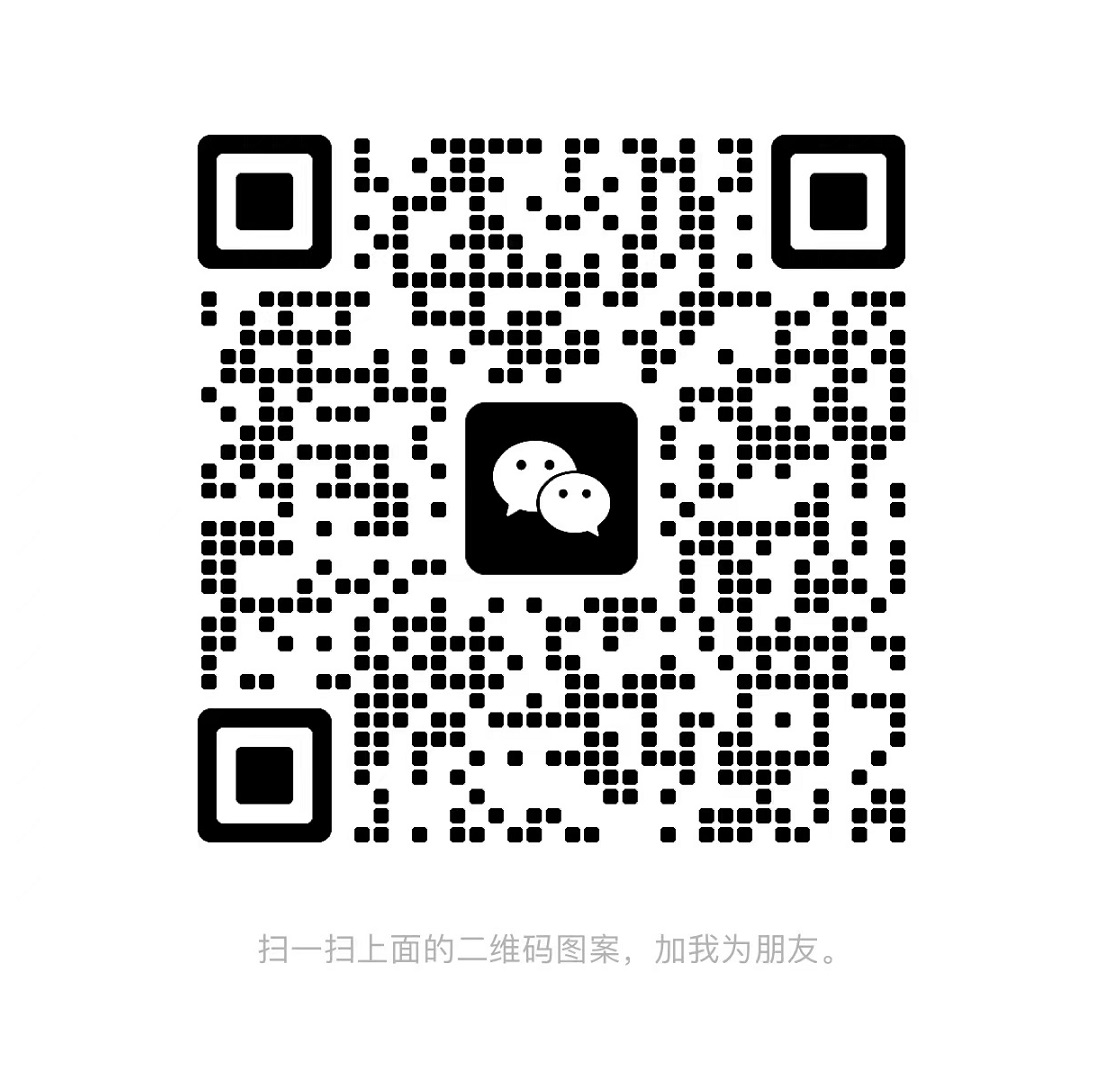