
本文共 4672 字,大约阅读时间需要 15 分钟。
简介
Pyjnius是一个用于访问Java类的Python库。
适用场景:极个别的加密算法等内容,用python不方便实现或者实现较耗时,可基于Pyjnius把java类当做python库使用。
文档:http://pyjnius.readthedocs.io/en/latest/installation.html
下载地址:https://pypi.python.org/pypi?%3Aaction=search&term=jnius&submit=search
注意jnius的版本管理有点混乱,目前看来选择jniusx比较好。
git地址:https://github.com/kivy/pyjnius/blob/master/docs/source/index.rst
安装
先安装Java JDK 和JRE、Cython
#
pip3 install cython
# pip3 install jniusx
Collecting jniusx
Downloading jniusx - 1.0.5. tar.gz
Requirement already satisfied: six >=
1.7.0 in /opt/python
3.5 / lib / python3.5 / site - packages(
from jniusx)
Requirement already satisfied: cython in
/opt/python
3.5 / lib / python3.5 / site - packages(
from jniusx)
Installing collected packages: jniusx
Running setup.py install
for jniusx...done
Successfully installed jniusx - 1.0.5
注意:jnius安装的坑比较多,请参考http://stackoverflow.com/search?q=jnius
如果出现ImportError,一般是java环境变量或者path没有配置好。
jnius/jnius.c:4:20: fatal error: Python.h 一般为缺python-dev, yum -y install python-devel
pip 安装不成功可以尝试 setup.py方式。
jnius/jnius.c: No such file or directory 需要利用原来保存的clone。
快速入门
hello world 实例:
#!/usr/bin/env python
#- * -coding: utf - 8 - * -
#jnius_quick2.py
# Author Rongzhong Xu 2016 - 08 - 02 wechat:
pythontesting
# https: //bitbucket.org/china-testing/python-chinese-library/src
""
"
jnius demo
Tested in python2.7
""
"
from jnius
import autoclass
System = autoclass('java.lang.System')
System.out.println('Hello World')
堆栈实例:
#!/usr/bin/env python
#- * -coding: utf - 8 - * -
#jnius_quick1.py
# Author Rongzhong Xu 2016 - 08 - 02 wechat:
pythontesting
# https: //bitbucket.org/china-testing/python-chinese-library/src
""
"
jnius demo
Tested in python2.7
""
"
from jnius
import autoclass
Stack = autoclass('java.util.Stack')
stack = Stack()
stack.push('hello')
stack.push('world')
print(stack.pop())# -- > 'world'
print(stack.pop())# -- > 'hello'
调用java String的hashCode
#!/usr/bin/env python
#- * -coding: utf - 8 - * -
#jnius_quick3.py
# Author Rongzhong Xu 2016 - 08 - 02 wechat:
pythontesting
# https: //bitbucket.org/china-testing/python-chinese-library/src
""
"
jnius demo: Call java String 's hashCode
Tested in python2.7
""
"
from jnius
import autoclass
String = autoclass('java.lang.String')
print(String("hello").hashCode())
调用jar包
#!python
#
vi com / sample / Beach.java
package com.sample;
public class Beach {
private String name;
private String city;
public Beach(String name, String city) {
this.name = name;
this.city = city;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
}
#
echo Main - Class: Beach > manifest.txt
# jar cvfm Test.jar manifest.txt com /
sample /*.class*/
测试:
#!python
#
ipython
Python 3.5.2(
default, Nov 7 2016, 18: 53: 51)
Type "copyright", "credits"
or "license"
for more information.
IPython 5.1.0--An enhanced Interactive Python
.
? - > Introduction and overview of IPython
's features.
% quickref - > Quick reference.
help - > Python 's own help system.
object ? - > Details about 'object',
use 'object??'
for extra details.
In[2]: #注意要先把jar加载CLASSPATH环境变量。
In[3]: from jnius
import autoclass
In[4]: Bla = autoclass(
'com.sample.Beach')
In[5]: s = Bla("Tom", "Shenzhen")
In[6]: s.getName()
Out[6]: 'Tom'
``
`
封装某模块的加密机制为python包实例:
* 拷贝: com cn org 到新建的临时目录
* echo Main-Class: AESUtil >manifest.txt
* jar cvfm Test.jar manifest.txt *
测试代码:
`
``
python
# - * -coding: utf - 8 - * -
#注意要先把jar加载CLASSPATH环境变量。
from jnius
import autoclass
AESUtil = autoclass(
'com.oppo.sso.util.AESUtil')
email = AESUtil.aesEncrypt(
"hello@126.com", "我是一个加密密钥")
print(email)# !python
# ipython
Python 3.5.2(
default, Nov 7 2016, 18: 53: 51)
Type "copyright", "credits"
or "license"
for more information.
IPython 5.1.0--An enhanced Interactive Python
.
? - > Introduction and overview of IPython
's features.
% quickref - > Quick reference.
help - > Python 's own help system.
object ? - > Details about 'object',
use 'object??'
for extra details.
In[2]: #注意要先把jar加载CLASSPATH环境变量。
In[3]: from jnius
import autoclass
In[4]: Bla = autoclass(
'com.sample.Beach')
In[5]: s = Bla("Tom", "Shenzhen")
In[6]: s.getName()
Out[6]: 'Tom'
``
`
封装某模块的加密机制为python包实例:
* 拷贝: com cn org 到新建的临时目录
* echo Main-Class: AESUtil >manifest.txt
* jar cvfm Test.jar manifest.txt *
测试代码:
`
``
python
# - * -coding: utf - 8 - * -
#注意要先把jar加载CLASSPATH环境变量。
from jnius
import autoclass
AESUtil = autoclass(
'com.oppo.sso.util.AESUtil')
email = AESUtil.aesEncrypt(
"hello@126.com", "我是一个加密密钥")
print(email)
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持我们。
本文标题: Python基于pyjnius库实现访问java类
本文地址: http://www.cppcns.com/jiaoben/python/329335.html
转载地址:https://blog.csdn.net/weixin_33865450/article/details/113388504 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关于作者
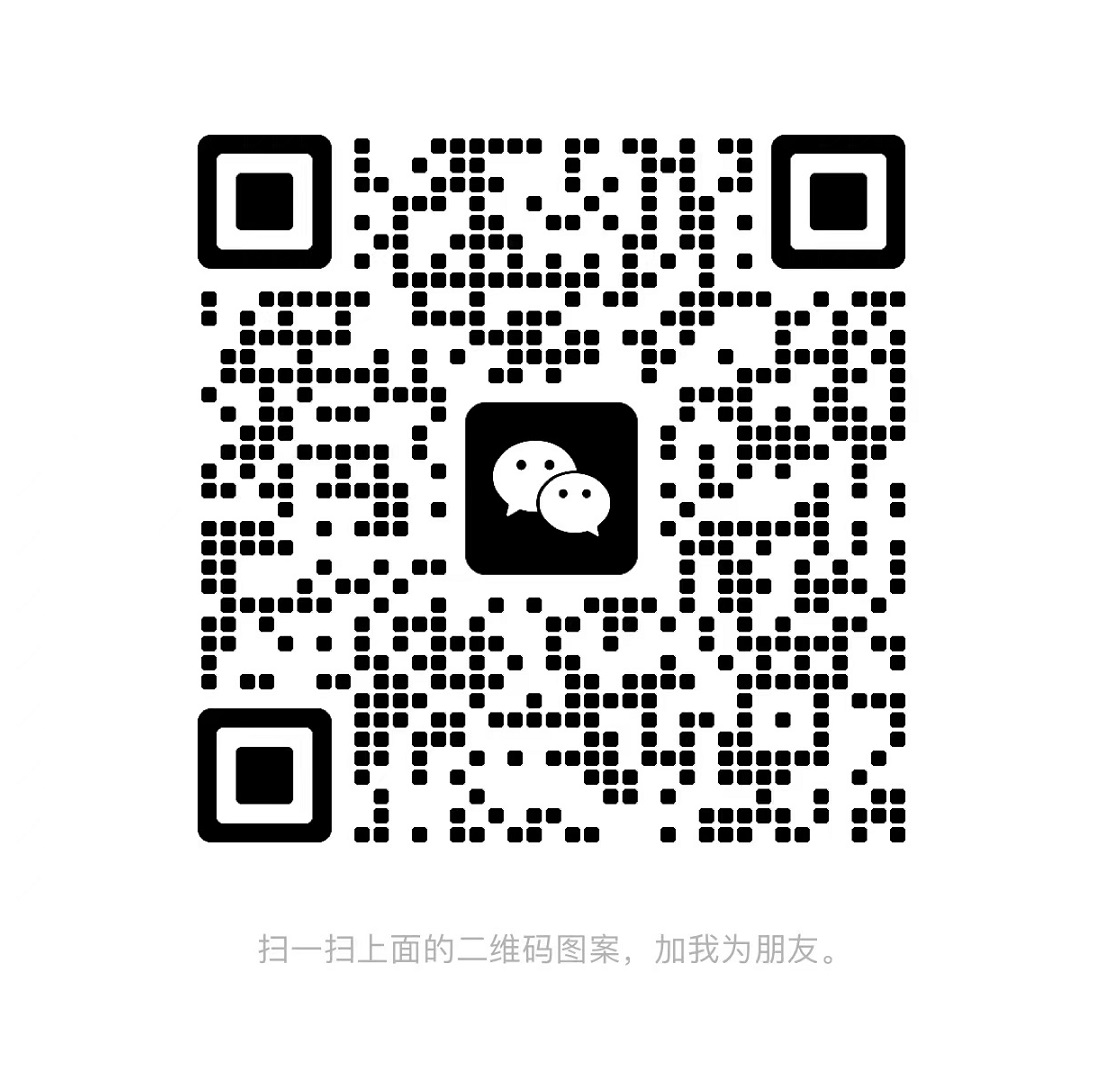