官网: 4.5:
一。基于HTTP的WWW组件
1.Get传输
1)脚本
WebManager.cs
using UnityEngine;using System.Collections;public class WebManager : MonoBehaviour { string info = " "; void OnGUI() { GUI.Label(new Rect(100, 20, 300, 30), info); if (GUI.Button(new Rect(100, 80, 200, 60), "Get访问")) { StartCoroutine(IGetData()); } } IEnumerator IGetData() { // 网络传输器(http://URL?参数=值&参数=值) WWW www = new WWW("http://127.0.0.1:8080/HelloUnity/HelloUnity?Name=Eminem&Pasd=0999"); // yield 将需要return的数据全都放到迭代器内才执行 yield return www; // www.text是请求响应后返回的数据 info = www.text.ToString(); // 如果发生url错误等意外 if (www.error != null) { info = www.error.ToString(); yield return null; } }}
2)UI
GameObject,Create Empty,关联脚本,
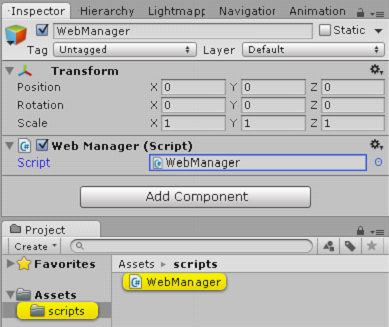
3)启动服务器
(服务器创建方法见 一。4.)
4)访问
2.Post传输
1)脚本
升级WebManager.cs
using UnityEngine;using System.Collections;public class WebManager : MonoBehaviour { string info = " "; void OnGUI() { GUI.Label(new Rect(100, 20, 300, 30), info); if (GUI.Button(new Rect(100, 80, 200, 60), "Get访问")) { StartCoroutine(IGetData()); } if (GUI.Button(new Rect(100, 180, 200, 60), "Post访问")) { StartCoroutine(IPostData()); } } IEnumerator IGetData() { 略 } IEnumerator IPostData() { Hashtable headers = new Hashtable(); // 键值对存储 headers.Add("Contend-Type", "application/x-www-form-urlencoded"); // 1.http头 string data = "Name=Alizee&Pasd=3745"; // 参数 byte[] bs = System.Text.UTF8Encoding.UTF8.GetBytes(data); // 1.数据。将参数转为字节 // 3.网络传输器(URL,数据,http头) WWW www = new WWW("http://127.0.0.1:8080/HelloUnity/HelloUnity", bs, headers); // yield 将需要return的数据全都放到迭代器内才执行 yield return www; info = www.text.ToString(); // 如果发生url错误等意外 if (www.error != null) { info = www.error.ToString(); yield return null; } } }
2)访问
3)WWWForm提交数据
using UnityEngine;using System.Collections;public class WebManager : MonoBehaviour { void OnGUI() { if (GUI.Button(new Rect(100, 130, 200, 30), "WWWForm访问")) { StartCoroutine(WwwFormPost()); } } IEnumerator WwwFormPost() { //网页表单,辅助类。用来生成表单数据,使用WWW类传递到web服务器 WWWForm form = new WWWForm(); // AddField(参数名,值) form.AddField("caonima", "dsdssds"); form.AddField("rininai", "365djyut"); // post方法( URL,表单 ) WWW www = new WWW("http://127.0.0.1:8080/HelloUnity/UnityImage", form); yield return www; if (www.error != null) { info = www.error.ToString(); yield return null; } }}
3.图片上传下载
1)脚本
升级WebManager.cs
using UnityEngine;using System.Collections;public class WebManager : MonoBehaviour { string info = " "; public Texture2D m_uploadImage; // 上传的图片 private Texture2D m_downloadTexture; // 下载的图片 void OnGUI() { GUI.Label(new Rect(100, 10, 300, 50), info); if (GUI.Button(new Rect(100, 70, 200, 30), "Get访问")) { StartCoroutine(IGetData()); } if (GUI.Button(new Rect(100, 120, 200, 30), "Post访问")) { StartCoroutine(IPostData()); } if (GUI.Button(new Rect(100, 170, 200, 30), "请求图片")) { StartCoroutine(IRequestPNG()); } // 如果服务器返回了图片信息,绘制 if (m_downloadTexture != null) { GUI.DrawTexture(new Rect(100, 220, 256, 256), m_downloadTexture); } } IEnumerator IGetData() { 略 } IEnumerator IPostData() { 略 } IEnumerator IRequestPNG() { m_downloadTexture = new Texture2D(256, 256); // 须初始化 // 图片转字节 byte[] bs = m_uploadImage.EncodeToPNG(); //网页表单,辅助类。用来生成表单数据,使用WWW类传递到web服务器 WWWForm form = new WWWForm(); // 添加二进制数据到表单(参数名,值-源数据,目标文件名,文件类型) /** * 提交文件,只能是post方式 * WWWForm同html中声明属性enctype="multipart/form-data" * 对应http协议请求: * Content-Type: image/png * Content-disposition: form-data; name="unityPic"; filename="picUnity" */ form.AddBinaryData("unityPic", bs, "picUnity", "image/png"); // post方法( URL,表单 ) WWW www = new WWW("http://127.0.0.1:8080/HelloUnity/UnityImage", form); yield return www; info = "图片:" + www.text; // 服务器返回的图片地址保存在这里面 /**************************************************/ // 下载网络图片 WWW www2 = new WWW(www.text); //等待直到下载完全结束 yield return www2; // 指定下载的图像到物体的主纹理 // 必须是JPG或者PNG格式格式的图片 www2.LoadImageIntoTexture(m_downloadTexture); } }
2)访问
4.补充:Java服务器架设
Tomcat+MyEclipse。
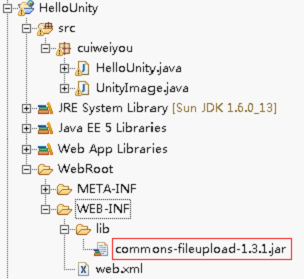
1)纯文本交互Servlet
package cuiweiyou;import *;public class HelloUnity extends HttpServlet { /** 响应 get 请求 **/ protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { req.setCharacterEncoding("UTF-8"); resp.setContentType("text/html; charset=UTF-8"); // 获取Unity通过get发送来的参数 String name = req.getParameter("Name"); String pasd = req.getParameter("Pasd"); System.out.println("收到Unity通过get发来的数据,Name:" + name + ", Pasd:" + pasd); // 向Unity发送消息 resp.getWriter().write("GET到 你的用户名:" + name + ",密码:" + pasd); } /** 响应 post 请求 **/ protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { req.setCharacterEncoding("UTF-8"); resp.setContentType("text/html; charset=UTF-8"); // 获取Unity通过get发送来的参数 String name = req.getParameter("Name"); String pasd = req.getParameter("Pasd"); System.out.println("收到Unity通过post发来的数据,Name:" + name + ", Pasd:" + pasd); // 向Unity发送文本消息 resp.getWriter().write("Post到 你的用户名:" + name + ",密码:" + pasd); }}
2)图片上传下载Servlet
package cuiweiyou;import * ; // 用到了 commons-fileupload-*.jar,http://commons.apache.org/proper/commons-fileupload/ public class UnityImage extends HttpServlet { /** 响应 form的post 请求 **/ protected void doPost(HttpServletRequest request, HttpServletResponse resp) throws ServletException, IOException { request.setCharacterEncoding("UTF-8"); resp.setContentType("text/html; charset=UTF-8"); // true:request支持文件上传;false:request出了问题,如
3)web.xml
HelloUnity cuiweiyou.HelloUnity HelloUnity /HelloUnity UnityImage cuiweiyou.UnityImage UnityImage /UnityImage
二。基于UDP的Network组件
脑力有限,请大牛指点!
三。基于C#-Socket
脑力有限,请大牛指点!
- end