
C#基础知识整理 基础知识(17)ILiest接口——泛型
发布日期:2021-11-07 06:41:06
浏览次数:7
分类:技术文章
本文共 6717 字,大约阅读时间需要 22 分钟。
对于ArrayList中如果插入值类型会引发装箱操作,而取出值类型又需要拆箱,如下
ArrayList myArrayList = new ArrayList(); myArrayList.Add(40);//装箱 myArrayList.Add(80);//装箱 Int32 a1 = (Int32)myArrayList[0];//拆箱 Int32 a2 = (Int32)myArrayList[1];//拆箱
从而造成性能的消耗。至于装箱的详细解说见下一篇。
为了解决这些问题,C#中有支持泛型的IList<T>接口,下面看详细代码,其实结构都和IList一样,只是增加了泛型。
////// 泛型集合类 /// ///public class List : IList , IList { /// /// 泛型迭代器 /// ///public struct Enumertor : IEnumerator, IEnumerator { //迭代索引 private int index; //迭代器所属的集合对象引用 private List list; public Enumertor(List container) { this.list = container; this.index = -1; } public void Dispose() { } /// /// 显示实现IEnumerator的Current属性 /// object IEnumerator.Current { get { return list[index]; } } ////// 实现IEnumerator public T Current { get { return list[index]; } } ///的Current属性 /// /// 迭代器指示到下一个数据位置 /// ///public bool MoveNext() { if (this.index < list.Count) { ++this.index; } return this.index < list.Count; } public void Reset() { this.index = -1; } } /// /// 保存数据的数组,T类型则体现了泛型的作用。 /// private T[] array; ////// 当前集合的长度 /// private int count; ////// 默认构造函数 /// public List() : this(1) { } public List(int capacity) { if (capacity < 0) { throw new Exception("集合初始长度不能小于0"); } if (capacity == 0) { capacity = 1; } this.array = new T[capacity]; } ////// 集合长度 /// public int Count { get { return this.count; } } ////// 集合实际长度 /// public int Capacity { get { return this.array.Length; } } ////// 是否固定大小 /// public bool IsFixedSize { get { return false; } } ////// 是否只读 /// public bool IsReadOnly { get { return false; } } ////// 是否可同属性 /// public bool IsSynchronized { get { return false; } } ////// 同步对象 /// public object SyncRoot { get { return null; } } ////// 长度不够时,重新分配长度足够的数组 /// ///private T[] GetNewArray() { return new T[(this.array.Length + 1) * 2]; } /// /// 实现IList /// public void Add(T value) { int newCount = this.count + 1; if (this.array.Length < newCount) { T[] newArray = GetNewArray(); Array.Copy(this.array, newArray, this.count); this.array = newArray; } this.array[this.count] = value; this.count = newCount; } ///Add方法 /// /// 向集合末尾添加对象 /// /// ///int IList.Add(object value) { ((IList )this).Add((T)value); return this.count - 1; } /// /// 实现IList /// ///索引器 /// public T this[int index] { get { if (index < 0 || index >= this.count) { throw new ArgumentOutOfRangeException("index"); } return this.array[index]; } set { if (index < 0 || index >= this.count) { throw new ArgumentOutOfRangeException("index"); } this.array[index] = value; } } /// /// 显示实现IList接口的索引器 /// /// ///object IList.this[int index] { get { return ((IList )this)[index]; } set { ((IList )this)[index] = (T)value; } } /// /// 删除集合中的元素 /// /// /// public void RemoveRange(int index, int count) { if (index < 0) { throw new ArgumentOutOfRangeException("index"); } int removeIndex = index + count; if (count < 0 || removeIndex > this.count) { throw new ArgumentOutOfRangeException("index"); } Array.Copy(this.array, index + 1, this.array, index + count - 1, this.count - removeIndex); this.count -= count; } ////// 实现IList /// ///接口的indexOf方法 /// public int IndexOf(T value) { int index = 0; if (value == null) { while (index < this.count) { if (this.array[index] == null) { return index; } ++index; } } else { while (index < this.count) { if (value.Equals(this.array[index])) { return index; } ++index; } } return -1; } /// /// 显示实现IList接口的IndexOf方法 /// /// ///int IList.IndexOf(object value) { return ((IList )this).IndexOf((T)value); } /// /// 查找对应数组项 /// /// /// ///public int IndexOf(object o, IComparer compar) { int index = 0; while (index < this.count) { if (compar.Compare(this.array[index], o) == 0) { return index; } ++index; } return -1; } /// /// 实现IList /// ///接口的Remove方法 /// public bool Remove(T value) { int index = this.IndexOf(value); if (index >= 0) { this.RemoveRange(index, 1); return true; } return false; } /// /// 显示实现IList接口的Remove方法,此处显示实现 /// /// void IList.Remove(object value) { ((IList)this).Remove((T)value); } /// /// 从集合指定位置删除对象的引用 /// /// public void RemoveAt(int index) { RemoveRange(index, 1); } ////// 弹出集合的最后一个元素 /// ///public object PopBack() { object o = this.array[this.count - 1]; RemoveAt(this.count - 1); return o; } /// /// 弹出集合第一个对象 /// ///public object PopFront() { object o = this.array[0]; RemoveAt(0); return o; } /// /// 实现IList /// /// public void Insert(int index, T value) { if (index >= this.count) { throw new ArgumentOutOfRangeException("index"); } int newCount = this.count + 1; if (this.array.Length < newCount) { T[] newArray = GetNewArray(); Array.Copy(this.array, newArray, index); newArray[index] = value; Array.Copy(this.array, index, newArray, index + 1, this.count - index); this.array = newArray; } else { Array.Copy(this.array, index, this.array, index + 1, this.count - index); this.array[index] = value; } this.count = newCount; } ///接口的Insert方法 /// /// 显示实现IList接口的Insert方法 /// /// /// void IList.Insert(int index, object value) { ((IList)this).Insert(index, (T)value); } /// /// 实现IList /// ///接口的Contains方法 /// public bool Contains(T value) { return this.IndexOf(value) >= 0; } /// /// 显示实现IList /// ///接口的Contains方法 /// bool IList.Contains(object value) { return ((IList )this).IndexOf((T)value) >= 0; } /// /// 将集合压缩为实际长度 /// public void TrimToSize() { if (this.array.Length > this.count) { T[] newArray = null; if (this.count > 0) { newArray = new T[this.count]; Array.Copy(this.array, newArray, this.count); } else { newArray = new T[1]; } this.array = newArray; } } ////// 清空集合 /// public void Clear() { this.count = 0; } ////// 实现IEnumerable接口的GetEnumerator方法 /// ///public IEnumerator GetEnumerator() { Enumertor ator = new Enumertor (this); return ator; } /// /// 显示实现IEnumerable接口的GetEnumerator方法 /// ///IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } /// /// 实现ICollection /// /// public void CopyTo(T[] array, int index) { Array.Copy(this.array, 0, array, index, this.count); } ///接口的CopyTo方法 /// /// 显示实现实现ICollection /// /// void ICollection.CopyTo(Array array, int index) { Array.Copy(this.array, 0, array, index, this.count); } }接口的CopyTo方法 ///
调用:
static void Main(string[] args) { //由于已经指定了int,因此加入值类型不会有装箱拆箱操作。 List tList = new List (); tList.Add(25); tList.Add(30); foreach (int n in tList) { Console.WriteLine(n); } Console.ReadLine(); }
代码:
转载地址:https://blog.csdn.net/yysyangyangyangshan/article/details/8202705 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
哈哈,博客排版真的漂亮呢~
[***.90.31.176]2024年04月18日 12时32分36秒
关于作者
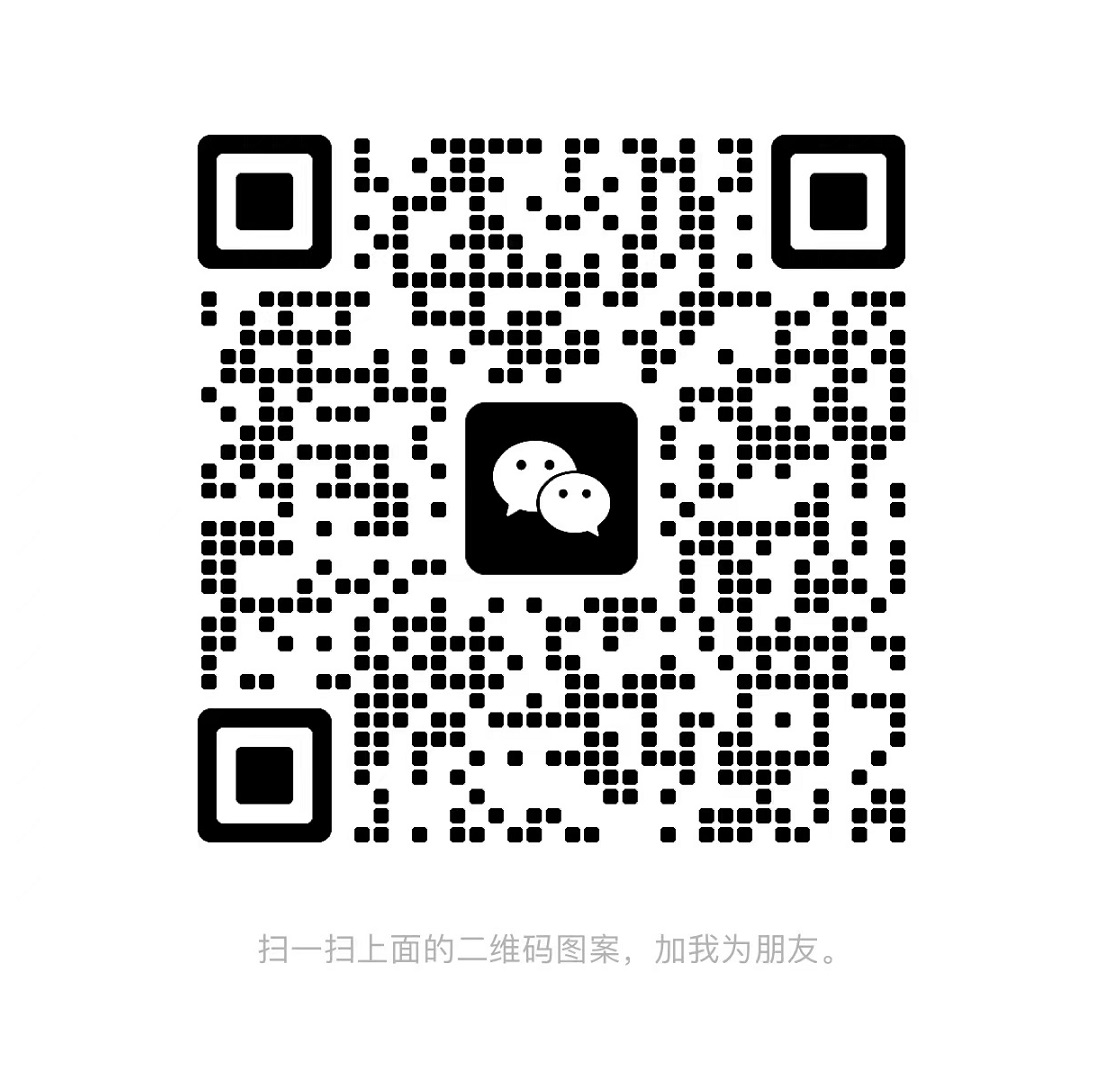
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
iOS应用崩溃日志分析
2019-04-30
报文数据的txt文件 转换成wireshark可以识别的k12文件
2019-04-30
vue 大致总结一
2019-04-30
python面试总结 python同源策略 跨域问题(一)
2019-04-30
django中HttpResponse render redirect 使用和区别
2019-04-30
MySQL数据库的详细安装步骤
2019-04-30
python 基础 爬虫音乐资源案例
2019-04-30
python基础爬虫 视频的下载
2019-04-30
python中把图片 或者 数据存储到本地 用csv方式储存
2019-04-30
python中csv格式转换为excle格式
2019-04-30
爬取图片下载保存 例子详解 代码与注释
2019-04-30
python 在安装第三方库时候 怎样使用国内镜像源 实现快速下载
2019-04-30
python 爬取龙岭迷窟视频 对于视频格式m3u8 下载多个ts文件 合并成MP4
2019-04-30
python 爬取百度地图api数据
2019-04-30
MySQL 数据库在dos命令下的基本操作
2019-04-30
MySQL 学习文档 和安装步骤
2019-04-30
在Scrapy中怎样把数据储存到Mysql 或者Redis中
2019-04-30
python 在爬虫中怎样把数据添加到excle样式中
2019-04-30