
安卓崩溃日志写入文件中
发布日期:2021-06-28 19:37:33
浏览次数:2
分类:技术文章
本文共 28758 字,大约阅读时间需要 95 分钟。
一.代码
1.1捕获全局异常工具类
public class CrashHandler implements Thread.UncaughtExceptionHandler { public static String TAG = "MyCrash"; // 系统默认的UncaughtException处理类 private Thread.UncaughtExceptionHandler mDefaultHandler; private static CrashHandler instance = new CrashHandler(); private Context mContext; // 用来存储设备信息和异常信息 private Mapinfos = new HashMap (); // 用于格式化日期,作为日志文件名的一部分 private DateFormat formatter = new SimpleDateFormat("yyyy-MM-dd"); /** * 保证只有一个CrashHandler实例 */ private CrashHandler() { } /** * 获取CrashHandler实例 ,单例模式 */ public static CrashHandler getInstance() { return instance; } /** * 初始化 * * @param context */ public void init(Context context) { mContext = context; // 获取系统默认的UncaughtException处理器 mDefaultHandler = Thread.getDefaultUncaughtExceptionHandler(); // 设置该CrashHandler为程序的默认处理器 Thread.setDefaultUncaughtExceptionHandler(this); autoClear(30); } /** * 当UncaughtException发生时会转入该函数来处理 */ @Override public void uncaughtException(Thread thread, Throwable ex) { if (!handleException(ex) && mDefaultHandler != null) { // 如果用户没有处理则让系统默认的异常处理器来处理 mDefaultHandler.uncaughtException(thread, ex); } else { SystemClock.sleep(3000); // 退出程序 android.os.Process.killProcess(android.os.Process.myPid()); System.exit(1); } } /** * 自定义错误处理,收集错误信息 发送错误报告等操作均在此完成. * * @param ex * @return true:如果处理了该异常信息; 否则返回false. */ private boolean handleException(Throwable ex) { if (ex == null) return false; try { // 使用Toast来显示异常信息 new Thread() { @Override public void run() { Looper.prepare(); Toast.makeText(mContext, "很抱歉,程序出现异常,请将日志发给研发人员.", Toast.LENGTH_LONG).show(); Looper.loop(); } }.start(); // 收集设备参数信息 collectDeviceInfo(mContext); // 保存日志文件 saveCrashInfoFile(ex); // 重启应用(按需要添加是否重启应用)// Intent intent = mContext.getPackageManager().getLaunchIntentForPackage(mContext.getPackageName());// intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);// mContext.startActivity(intent); SystemClock.sleep(3000); } catch (Exception e) { e.printStackTrace(); } return true; } /** * 收集设备参数信息 * * @param ctx */ public void collectDeviceInfo(Context ctx) { try { PackageManager pm = ctx.getPackageManager(); PackageInfo pi = pm.getPackageInfo(ctx.getPackageName(), PackageManager.GET_ACTIVITIES); if (pi != null) { String versionName = pi.versionName + ""; String versionCode = pi.versionCode + ""; infos.put("versionName", versionName); infos.put("versionCode", versionCode); } } catch (PackageManager.NameNotFoundException e) { Log.e(TAG, "an error occured when collect package info", e); } Field[] fields = Build.class.getDeclaredFields(); for (Field field : fields) { try { field.setAccessible(true); infos.put(field.getName(), field.get(null).toString()); } catch (Exception e) { Log.e(TAG, "an error occured when collect crash info", e); } } } /** * 保存错误信息到文件中 * * @param ex * @return 返回文件名称, 便于将文件传送到服务器 * @throws Exception */ private String saveCrashInfoFile(Throwable ex) throws Exception { StringBuffer sb = new StringBuffer(); try { SimpleDateFormat sDateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss", Locale.getDefault()); String date = sDateFormat.format(new java.util.Date()); sb.append("\r\n" + date + "\n"); for (Map.Entry entry : infos.entrySet()) { String key = entry.getKey(); String value = entry.getValue(); sb.append(key + "=" + value + "\n"); } Writer writer = new StringWriter(); PrintWriter printWriter = new PrintWriter(writer); ex.printStackTrace(printWriter); Throwable cause = ex.getCause(); while (cause != null) { cause.printStackTrace(printWriter); cause = cause.getCause(); } printWriter.flush(); printWriter.close(); String result = writer.toString(); sb.append(result); String fileName = writeFile(sb.toString()); return fileName; } catch (Exception e) { Log.e(TAG, "an error occured while writing file...", e); sb.append("an error occured while writing file...\r\n"); writeFile(sb.toString()); } return null; } /** * @author Longchengbin * @description 将错误信息写入本地新建的日志文件 * @since 2020/12/21 10:01 **/ private String writeFile(String sb) throws Exception { String time = formatter.format(new Date()); String fileName = "crash-" + time + ".log"; if (FileUtil.hasSdcard()) { String path = getGlobalpath(); File dir = new File(path); if (!dir.exists()) dir.mkdirs(); FileOutputStream fos = new FileOutputStream(path + fileName, true); fos.write(sb.getBytes()); fos.flush(); fos.close(); } return fileName; } /** * @author Longchengbin * @description 写入文件的路径 * @since 2020/12/21 10:09 **/ public static String getGlobalpath() { // TODO: 2020/12/21 设备根目录下的crash文件夹 return Environment.getExternalStorageDirectory().getAbsolutePath() + File.separator + "crash" + File.separator; } public static void setTag(String tag) { TAG = tag; } /** * 文件删除 * 文件保存天数 */ public void autoClear(final int autoClearDay) { FileUtil.delete(getGlobalpath(), new FilenameFilter() { @Override public boolean accept(File file, String filename) { String s = FileUtil.getFileNameWithoutExtension(filename); int day = autoClearDay < 0 ? autoClearDay : -1 * autoClearDay; String date = "crash-" + DateUtil.getOtherDay(day); return date.compareTo(s) >= 0; } }); }}
1.2时间工具类
public final class DateUtil { /** yyyy-MM-dd HH:mm:ss字符串 */ public static final String DEFAULT_DATE_TIME_FORMAT = "yyyy-MM-dd HH:mm:ss"; /** yyyy-MM-dd字符串 */ public static final String DEFAULT_FORMAT_DATE = "yyyy-MM-dd"; /** HH:mm:ss字符串 */ public static final String DEFAULT_FORMAT_TIME = "HH:mm:ss"; /** yyyy-MM-dd HH:mm:ss格式 */ public static final ThreadLocaldefaultDateTimeFormat = new ThreadLocal () { @Override protected SimpleDateFormat initialValue() { return new SimpleDateFormat(DEFAULT_DATE_TIME_FORMAT); } }; /** yyyy-MM-dd格式 */ public static final ThreadLocal defaultDateFormat = new ThreadLocal () { @Override protected SimpleDateFormat initialValue() { return new SimpleDateFormat(DEFAULT_FORMAT_DATE); } }; /** HH:mm:ss格式 */ public static final ThreadLocal defaultTimeFormat = new ThreadLocal () { @Override protected SimpleDateFormat initialValue() { return new SimpleDateFormat(DEFAULT_FORMAT_TIME); } }; private DateUtil() { throw new RuntimeException(" ̄ 3 ̄"); } /** * 将long时间转成yyyy-MM-dd HH:mm:ss字符串 * @param timeInMillis 时间long值 * @return yyyy-MM-dd HH:mm:ss */ public static String getDateTimeFromMillis(long timeInMillis) { return getDateTimeFormat(new Date(timeInMillis)); } /** * 将long时间转成yyyy-MM-dd字符串 * @param timeInMillis * @return yyyy-MM-dd */ public static String getDateFromMillis(long timeInMillis) { return getDateFormat(new Date(timeInMillis)); } /** * 将date转成yyyy-MM-dd HH:mm:ss字符串 * * @param date Date对象 * @return yyyy-MM-dd HH:mm:ss */ public static String getDateTimeFormat(Date date) { return dateSimpleFormat(date, defaultDateTimeFormat.get()); } /** * 将年月日的int转成yyyy-MM-dd的字符串 * @param year 年 * @param month 月 1-12 * @param day 日 * 注:月表示Calendar的月,比实际小1 * 对输入项未做判断 */ public static String getDateFormat(int year, int month, int day) { return getDateFormat(getDate(year, month, day)); } /** * 将date转成yyyy-MM-dd字符串 * @param date Date对象 * @return yyyy-MM-dd */ public static String getDateFormat(Date date) { return dateSimpleFormat(date, defaultDateFormat.get()); } /** * 获得HH:mm:ss的时间 * @param date * @return */ public static String getTimeFormat(Date date) { return dateSimpleFormat(date, defaultTimeFormat.get()); } /** * 格式化日期显示格式 * @param sdate 原始日期格式 "yyyy-MM-dd" * @param format 格式化后日期格式 * @return 格式化后的日期显示 */ public static String dateFormat(String sdate, String format) { SimpleDateFormat formatter = new SimpleDateFormat(format); java.sql.Date date = java.sql.Date.valueOf(sdate); return dateSimpleFormat(date, formatter); } /** * 格式化日期显示格式 * @param date Date对象 * @param format 格式化后日期格式 * @return 格式化后的日期显示 */ public static String dateFormat(Date date, String format) { SimpleDateFormat formatter = new SimpleDateFormat(format); return dateSimpleFormat(date, formatter); } /** * 将date转成字符串 * @param date Date * @param format SimpleDateFormat * * 注: SimpleDateFormat为空时,采用默认的yyyy-MM-dd HH:mm:ss格式 * @return yyyy-MM-dd HH:mm:ss */ public static String dateSimpleFormat(Date date, SimpleDateFormat format) { if (format == null) format = defaultDateTimeFormat.get(); return (date == null ? "" : format.format(date)); } /** * 将"yyyy-MM-dd HH:mm:ss" 格式的字符串转成Date * @param strDate 时间字符串 * @return Date */ public static Date getDateByDateTimeFormat(String strDate) { return getDateByFormat(strDate, defaultDateTimeFormat.get()); } /** * 将"yyyy-MM-dd" 格式的字符串转成Date * @param strDate * @return Date */ public static Date getDateByDateFormat(String strDate) { return getDateByFormat(strDate, defaultDateFormat.get()); } /** * 将指定格式的时间字符串转成Date对象 * @param strDate 时间字符串 * @param format 格式化字符串 * @return Date */ public static Date getDateByFormat(String strDate, String format) { return getDateByFormat(strDate, new SimpleDateFormat(format)); } /** * 将String字符串按照一定格式转成Date * 注: SimpleDateFormat为空时,采用默认的yyyy-MM-dd HH:mm:ss格式 * @param strDate 时间字符串 * @param format SimpleDateFormat对象 * @exception ParseException 日期格式转换出错 */ private static Date getDateByFormat(String strDate, SimpleDateFormat format) { if (format == null) format = defaultDateTimeFormat.get(); try { return format.parse(strDate); } catch (ParseException e) { e.printStackTrace(); } return null; } /** * 将年月日的int转成date * @param year 年 * @param month 月 1-12 * @param day 日 * 注:月表示Calendar的月,比实际小1 */ public static Date getDate(int year, int month, int day) { Calendar mCalendar = Calendar.getInstance(); mCalendar.set(year, month - 1, day); return mCalendar.getTime(); } /** * 求两个日期相差天数 * * @param strat 起始日期,格式yyyy-MM-dd * @param end 终止日期,格式yyyy-MM-dd * @return 两个日期相差天数 */ public static long getIntervalDays(String strat, String end) { return ((java.sql.Date.valueOf(end)).getTime() - (java.sql.Date .valueOf(strat)).getTime()) / (3600 * 24 * 1000); } /** * 获得当前年份 * @return year(int) */ public static int getCurrentYear() { Calendar mCalendar = Calendar.getInstance(); return mCalendar.get(Calendar.YEAR); } /** * 获得当前月份 * @return month(int) 1-12 */ public static int getCurrentMonth() { Calendar mCalendar = Calendar.getInstance(); return mCalendar.get(Calendar.MONTH) + 1; } /** * 获得当月几号 * @return day(int) */ public static int getDayOfMonth() { Calendar mCalendar = Calendar.getInstance(); return mCalendar.get(Calendar.DAY_OF_MONTH); } /** * 获得今天的日期(格式:yyyy-MM-dd) * @return yyyy-MM-dd */ public static String getToday() { Calendar mCalendar = Calendar.getInstance(); return getDateFormat(mCalendar.getTime()); } /** * 获得昨天的日期(格式:yyyy-MM-dd) * @return yyyy-MM-dd */ public static String getYesterday() { Calendar mCalendar = Calendar.getInstance(); mCalendar.add(Calendar.DATE, -1); return getDateFormat(mCalendar.getTime()); } /** * 获得前天的日期(格式:yyyy-MM-dd) * @return yyyy-MM-dd */ public static String getBeforeYesterday() { Calendar mCalendar = Calendar.getInstance(); mCalendar.add(Calendar.DATE, -2); return getDateFormat(mCalendar.getTime()); } /** * 获得几天之前或者几天之后的日期 * @param diff 差值:正的往后推,负的往前推 * @return */ public static String getOtherDay(int diff) { Calendar mCalendar = Calendar.getInstance(); mCalendar.add(Calendar.DATE, diff); return getDateFormat(mCalendar.getTime()); } /** * 取得给定日期加上一定天数后的日期对象. * * 给定的日期对象 * @param amount 需要添加的天数,如果是向前的天数,使用负数就可以. * @return Date 加上一定天数以后的Date对象. */ public static String getCalcDateFormat(String sDate, int amount) { Date date = getCalcDate(getDateByDateFormat(sDate), amount); return getDateFormat(date); } /** * 取得给定日期加上一定天数后的日期对象. * * @param date 给定的日期对象 * @param amount 需要添加的天数,如果是向前的天数,使用负数就可以. * @return Date 加上一定天数以后的Date对象. */ public static Date getCalcDate(Date date, int amount) { Calendar cal = Calendar.getInstance(); cal.setTime(date); cal.add(Calendar.DATE, amount); return cal.getTime(); } /** * 获得一个计算十分秒之后的日期对象 * @param date * @param hOffset 时偏移量,可为负 * @param mOffset 分偏移量,可为负 * @param sOffset 秒偏移量,可为负 * @return */ public static Date getCalcTime(Date date, int hOffset, int mOffset, int sOffset) { Calendar cal = Calendar.getInstance(); if (date != null) cal.setTime(date); cal.add(Calendar.HOUR_OF_DAY, hOffset); cal.add(Calendar.MINUTE, mOffset); cal.add(Calendar.SECOND, sOffset); return cal.getTime(); } /** * 根据指定的年月日小时分秒,返回一个java.Util.Date对象。 * * @param year 年 * @param month 月 0-11 * @param date 日 * @param hourOfDay 小时 0-23 * @param minute 分 0-59 * @param second 秒 0-59 * @return 一个Date对象 */ public static Date getDate(int year, int month, int date, int hourOfDay, int minute, int second) { Calendar cal = Calendar.getInstance(); cal.set(year, month, date, hourOfDay, minute, second); return cal.getTime(); } /** * 获得年月日数据 * @param sDate yyyy-MM-dd格式 * @return arr[0]:年, arr[1]:月 0-11 , arr[2]日 */ public static int[] getYearMonthAndDayFrom(String sDate) { return getYearMonthAndDayFromDate(getDateByDateFormat(sDate)); } /** * 获得年月日数据 * @return arr[0]:年, arr[1]:月 0-11 , arr[2]日 */ public static int[] getYearMonthAndDayFromDate(Date date) { Calendar calendar = Calendar.getInstance(); calendar.setTime(date); int[] arr = new int[3]; arr[0] = calendar.get(Calendar.YEAR); arr[1] = calendar.get(Calendar.MONTH); arr[2] = calendar.get(Calendar.DAY_OF_MONTH); return arr; }}
1.3文件工具类
public final class FileUtil { private FileUtil() { throw new Error(" ̄﹏ ̄"); } /** 分隔符. */ public final static String FILE_EXTENSION_SEPARATOR = "."; /**"/"*/ public final static String SEP = File.separator; /** SD卡根目录 */ public static final String SDPATH = Environment .getExternalStorageDirectory() + File.separator; /** * 判断SD卡是否可用 * @return SD卡可用返回true */ public static boolean hasSdcard() { String status = Environment.getExternalStorageState(); return Environment.MEDIA_MOUNTED.equals(status); } /** * 读取文件的内容 * * 默认utf-8编码 * @param filePath 文件路径 * @return 字符串 * @throws IOException */ public static String readFile(String filePath) throws IOException { return readFile(filePath, "utf-8"); } /** * 读取文件的内容 * @param filePath 文件目录 * @param charsetName 字符编码 * @return String字符串 */ public static String readFile(String filePath, String charsetName) throws IOException { if (TextUtils.isEmpty(filePath)) return null; if (TextUtils.isEmpty(charsetName)) charsetName = "utf-8"; File file = new File(filePath); StringBuilder fileContent = new StringBuilder(""); if (!file.isFile()) return null; BufferedReader reader = null; try { InputStreamReader is = new InputStreamReader(new FileInputStream( file), charsetName); reader = new BufferedReader(is); String line = null; while ((line = reader.readLine()) != null) { if (!fileContent.toString().equals("")) { fileContent.append("\r\n"); } fileContent.append(line); } return fileContent.toString(); } finally { if (reader != null) { try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } } /** * 读取文本文件到List字符串集合中(默认utf-8编码) * @param filePath 文件目录 * @return 文件不存在返回null,否则返回字符串集合 * @throws IOException */ public static ListreadFileToList(String filePath) throws IOException { return readFileToList(filePath, "utf-8"); } /** * 读取文本文件到List字符串集合中 * @param filePath 文件目录 * @param charsetName 字符编码 * @return 文件不存在返回null,否则返回字符串集合 */ public static List readFileToList(String filePath, String charsetName) throws IOException { if (TextUtils.isEmpty(filePath)) return null; if (TextUtils.isEmpty(charsetName)) charsetName = "utf-8"; File file = new File(filePath); List fileContent = new ArrayList (); if (!file.isFile()) { return null; } BufferedReader reader = null; try { InputStreamReader is = new InputStreamReader(new FileInputStream( file), charsetName); reader = new BufferedReader(is); String line = null; while ((line = reader.readLine()) != null) { fileContent.add(line); } return fileContent; } finally { if (reader != null) { try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } } /** * 向文件中写入数据 * @param filePath 文件目录 * @param content 要写入的内容 * @param append 如果为 true,则将数据写入文件末尾处,而不是写入文件开始处 * @return 写入成功返回true, 写入失败返回false * @throws IOException */ public static boolean writeFile(String filePath, String content, boolean append) throws IOException { if (TextUtils.isEmpty(filePath)) return false; if (TextUtils.isEmpty(content)) return false; FileWriter fileWriter = null; try { createFile(filePath); fileWriter = new FileWriter(filePath, append); fileWriter.write(content); fileWriter.flush(); return true; } finally { if (fileWriter != null) { try { fileWriter.close(); } catch (IOException e) { e.printStackTrace(); } } } } /** * 向文件中写入数据 * 默认在文件开始处重新写入数据 * @param filePath 文件目录 * @param stream 字节输入流 * @return 写入成功返回true,否则返回false * @throws IOException */ public static boolean writeFile(String filePath, InputStream stream) throws IOException { return writeFile(filePath, stream, false); } /** * 向文件中写入数据 * @param filePath 文件目录 * @param stream 字节输入流 * @param append 如果为 true,则将数据写入文件末尾处; * 为false时,清空原来的数据,从头开始写 * @return 写入成功返回true,否则返回false * @throws IOException */ public static boolean writeFile(String filePath, InputStream stream, boolean append) throws IOException { if (TextUtils.isEmpty(filePath)) throw new NullPointerException("filePath is Empty"); if (stream == null) throw new NullPointerException("InputStream is null"); return writeFile(new File(filePath), stream, append); } /** * 向文件中写入数据 * 默认在文件开始处重新写入数据 * @param file 指定文件 * @param stream 字节输入流 * @return 写入成功返回true,否则返回false * @throws IOException */ public static boolean writeFile(File file, InputStream stream) throws IOException { return writeFile(file, stream, false); } /** * 向文件中写入数据 * @param file 指定文件 * @param stream 字节输入流 * @param append 为true时,在文件开始处重新写入数据; * 为false时,清空原来的数据,从头开始写 * @return 写入成功返回true,否则返回false * @throws IOException */ public static boolean writeFile(File file, InputStream stream, boolean append) throws IOException { if (file == null) throw new NullPointerException("file = null"); OutputStream out = null; try { createFile(file.getAbsolutePath()); out = new FileOutputStream(file, append); byte data[] = new byte[1024]; int length = -1; while ((length = stream.read(data)) != -1) { out.write(data, 0, length); } out.flush(); return true; } finally { if (out != null) { try { out.close(); stream.close(); } catch (IOException e) { e.printStackTrace(); } } } } /** * 复制文件 * @param sourceFilePath 源文件目录(要复制的文件目录) * @param destFilePath 目标文件目录(复制后的文件目录) * @return 复制文件成功返回true,否则返回false * @throws IOException */ public static boolean copyFile(String sourceFilePath, String destFilePath) throws IOException { InputStream inputStream = null; inputStream = new FileInputStream(sourceFilePath); return writeFile(destFilePath, inputStream); } /** * 获取某个目录下的文件名 * @param dirPath 目录 * @param fileFilter 过滤器 * @return 某个目录下的所有文件名 */ public static List getFileNameList(String dirPath, FilenameFilter fileFilter) { if (fileFilter == null) return getFileNameList(dirPath); if (TextUtils.isEmpty(dirPath)) return Collections.emptyList(); File dir = new File(dirPath); File[] files = dir.listFiles(fileFilter); if (files == null) return Collections.emptyList(); List conList = new ArrayList (); for (File file : files) { if (file.isFile()) conList.add(file.getName()); } return conList; } /** * 获取某个目录下的文件名 * @param dirPath 目录 * @return 某个目录下的所有文件名 */ public static List getFileNameList(String dirPath) { if (TextUtils.isEmpty(dirPath)) return Collections.emptyList(); File dir = new File(dirPath); File[] files = dir.listFiles(); if (files == null) return Collections.emptyList(); List conList = new ArrayList (); for (File file : files) { if (file.isFile()) conList.add(file.getName()); } return conList; } /** * 获取某个目录下的指定扩展名的文件名称 * @param dirPath 目录 * @return 某个目录下的所有文件名 */ public static List getFileNameList(String dirPath, final String extension) { if (TextUtils.isEmpty(dirPath)) return Collections.emptyList(); File dir = new File(dirPath); File[] files = dir.listFiles(new FilenameFilter() { @Override public boolean accept(File dir, String filename) { if (filename.indexOf("." + extension) > 0) return true; return false; } }); if (files == null) return Collections.emptyList(); List conList = new ArrayList (); for (File file : files) { if (file.isFile()) conList.add(file.getName()); } return conList; } /** * 获得文件的扩展名 * @param filePath 文件路径 * @return 如果没有扩展名,返回"" */ public static String getFileExtension(String filePath) { if (TextUtils.isEmpty(filePath)) { return filePath; } int extenPosi = filePath.lastIndexOf(FILE_EXTENSION_SEPARATOR); int filePosi = filePath.lastIndexOf(File.separator); if (extenPosi == -1) { return ""; } return (filePosi >= extenPosi) ? "" : filePath.substring(extenPosi + 1); } /** * 创建文件 * @param path 文件的绝对路径 * @return */ public static boolean createFile(String path) { if (TextUtils.isEmpty(path)) return false; return createFile(new File(path)); } /** * 创建文件 * @param file * @return 创建成功返回true */ public static boolean createFile(File file) { if (file == null || !makeDirs(getFolderName(file.getAbsolutePath()))) return false; if (!file.exists()) try { return file.createNewFile(); } catch (IOException e) { e.printStackTrace(); return false; } return false; } /** * 创建目录(可以是多个) * @param filePath 目录路径 * @return 如果路径为空时,返回false;如果目录创建成功,则返回true,否则返回false */ public static boolean makeDirs(String filePath) { if (TextUtils.isEmpty(filePath)) { return false; } File folder = new File(filePath); return (folder.exists() && folder.isDirectory()) || folder.mkdirs(); } /** * 创建目录(可以是多个) * @param dir 目录 * @return 如果目录创建成功,则返回true,否则返回false */ public static boolean makeDirs(File dir) { if (dir == null) return false; return (dir.exists() && dir.isDirectory()) || dir.mkdirs(); } /** * 判断文件是否存在 * @param filePath 文件路径 * @return 如果路径为空或者为空白字符串,就返回false;如果文件存在,且是文件, * 就返回true;如果不是文件或者不存在,则返回false */ public static boolean isFileExist(String filePath) { if (TextUtils.isEmpty(filePath)) { return false; } File file = new File(filePath); return (file.exists() && file.isFile()); } /** * 获得不带扩展名的文件名称 * @param filePath 文件路径 * @return */ public static String getFileNameWithoutExtension(String filePath) { if (TextUtils.isEmpty(filePath)) { return filePath; } int extenPosi = filePath.lastIndexOf(FILE_EXTENSION_SEPARATOR); int filePosi = filePath.lastIndexOf(File.separator); if (filePosi == -1) { return (extenPosi == -1 ? filePath : filePath.substring(0, extenPosi)); } if (extenPosi == -1) { return filePath.substring(filePosi + 1); } return (filePosi < extenPosi ? filePath.substring(filePosi + 1, extenPosi) : filePath.substring(filePosi + 1)); } /** * 获得文件名 * @param filePath 文件路径 * @return 如果路径为空或空串,返回路径名;不为空时,返回文件名 */ public static String getFileName(String filePath) { if (TextUtils.isEmpty(filePath)) { return filePath; } int filePosi = filePath.lastIndexOf(File.separator); return (filePosi == -1) ? filePath : filePath.substring(filePosi + 1); } /** * 获得所在目录名称 * @param filePath 文件的绝对路径 * @return 如果路径为空或空串,返回路径名;不为空时,如果为根目录,返回""; * 如果不是根目录,返回所在目录名称,格式如:C:/Windows/Boot */ public static String getFolderName(String filePath) { if (TextUtils.isEmpty(filePath)) { return filePath; } int filePosi = filePath.lastIndexOf(File.separator); return (filePosi == -1) ? "" : filePath.substring(0, filePosi); } /** * 判断目录是否存在 * directoryPath目录路径 * @return 如果路径为空或空白字符串,返回false;如果目录存在且,确实是目录文件夹, * 返回true;如果不是文件夹或者不存在,则返回false */ public static boolean isFolderExist(String directoryPath) { if (TextUtils.isEmpty(directoryPath)) { return false; } File dire = new File(directoryPath); return (dire.exists() && dire.isDirectory()); } /** * 删除指定文件或指定目录内的所有文件 * @param path 文件或目录的绝对路径 * @return 路径为空或空白字符串,返回true;文件不存在,返回true;文件删除返回true; * 文件删除异常返回false */ public static boolean deleteFile(String path) { if (TextUtils.isEmpty(path)) { return true; } return deleteFile(new File(path)); } /** * 删除指定文件或指定目录内的所有文件 * @param file * @return 路径为空或空白字符串,返回true;文件不存在,返回true;文件删除返回true; * 文件删除异常返回false */ public static boolean deleteFile(File file) { if (file == null) throw new NullPointerException("file is null"); if (!file.exists()) { return true; } if (file.isFile()) { return file.delete(); } if (!file.isDirectory()) { return false; } File[] files = file.listFiles(); if (files == null) return true; for (File f : files) { if (f.isFile()) { f.delete(); } else if (f.isDirectory()) { deleteFile(f.getAbsolutePath()); } } return file.delete(); } /** * 删除指定目录中特定的文件 * @param dir * @param filter */ public static void delete(String dir, FilenameFilter filter) { if (TextUtils.isEmpty(dir)) return; File file = new File(dir); if (!file.exists()) return; if (file.isFile()) file.delete(); if (!file.isDirectory()) return; File[] lists = null; if (filter != null) lists = file.listFiles(filter); else lists = file.listFiles(); if (lists == null) return; for (File f : lists) { if (f.isFile()) { f.delete(); } } } /** * 获得文件或文件夹的大小 * @param path 文件或目录的绝对路径 * @return 返回当前目录的大小 ,注:当文件不存在,为空,或者为空白字符串,返回 -1 */ public static long getFileSize(String path) { if (TextUtils.isEmpty(path)) { return -1; } File file = new File(path); return (file.exists() && file.isFile() ? file.length() : -1); }}
二.调用
2.1baseapplication初始化
2.2测试崩溃
2.3查看日志
错误原因以及定位所在位置(精确到所在类和行)
转载地址:https://blog.csdn.net/xxdw1992/article/details/111468772 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
网站不错 人气很旺了 加油
[***.192.178.218]2024年04月22日 17时32分04秒
关于作者
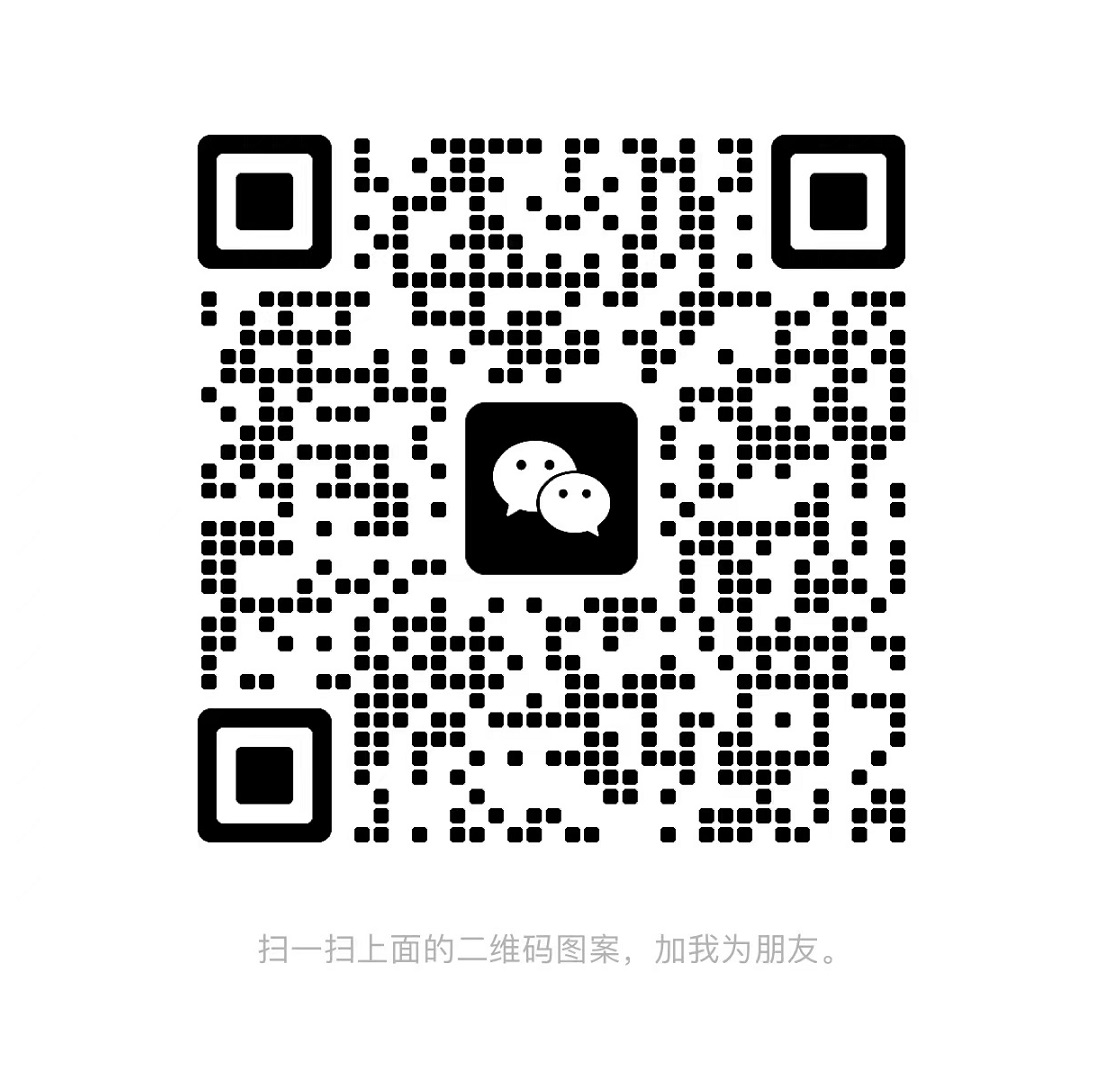
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
基于powershell的渗透测试工具nishang
2019-04-29
WebLogic漏洞复现(CVE-2018-2894)
2019-04-29
常用Linux命令
2019-04-29
Weblogic漏洞复现——XML Decoder(CVE-2017-10271)
2019-04-29
Webug4.0显错注入
2019-04-29
渗透测试流程
2019-04-29
SQL练习(less-2)报错注入
2019-04-29
VMware虚拟机安装Debian
2019-04-29
PC机win10练习v-P-n连接 虚拟机Win2003
2019-04-29
虚拟机-安装VMware Tools
2019-04-29
xshell连接VM centOS7
2019-04-29
bat脚本交互动态输入端口启动jar包
2019-04-29
mysql单个字段查询时设置是否区分大小写
2019-04-29
整合vue开发H5+跨平台app (以开发语音识别为例)
2019-04-29
windows自带的网络调试工具整理
2019-04-29
自定义一个Chrome翻译插件
2019-04-29
motan rpc 接口统一异常处理
2019-04-29
解析文件入库乱码问题解决
2019-04-29
clickhouse 条件语句内decimal除0报错处理
2019-04-29