
Android Studio 实现实现学生信息的增删改查 -源代码 四(Servlet + 连接MySql数据库)
完善StudentUpdateServlet
发布日期:2021-06-29 15:04:23
浏览次数:2
分类:技术文章
本文共 7539 字,大约阅读时间需要 25 分钟。
一、完善学生信息显示
1、修改和删除的按钮在student_list.xml当中
2、添加表头的xml,创建list_header.xml
3、在adapter当中动态加载表头
final LayoutInflater inflater = LayoutInflater.from(getApplicationContext()); View headView = inflater.inflate(R.layout.list_header, null); if(stuList.getHeaderViewsCount()==0) { stuList.addHeaderView(headView); }
二、实现修改学生信息的操作
(一)安卓端
1、创建显示学生信息的页面
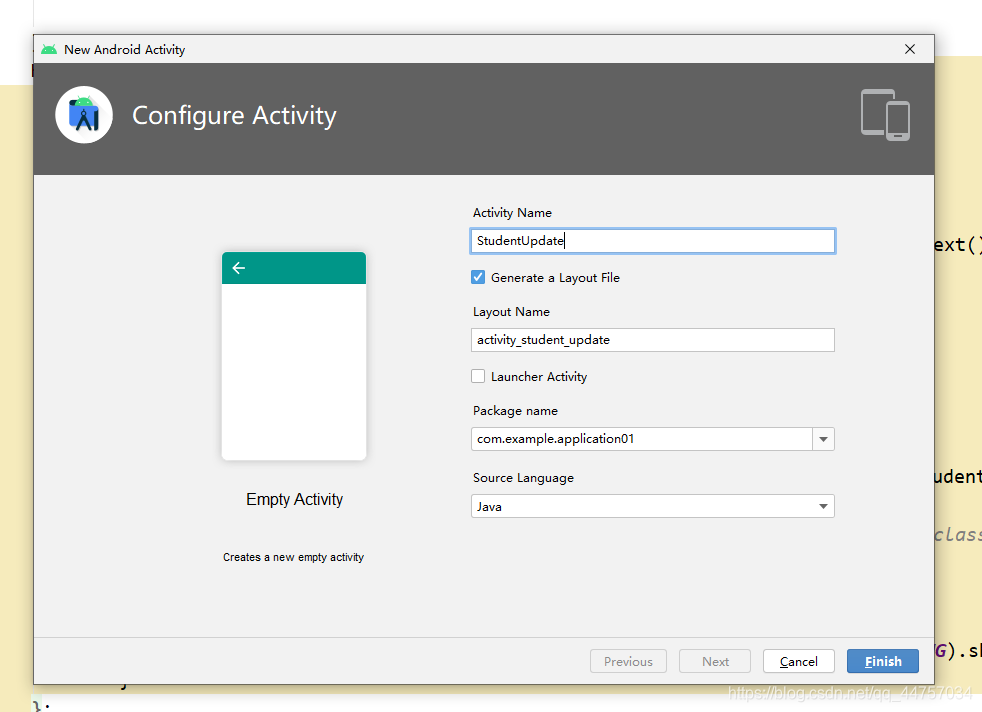
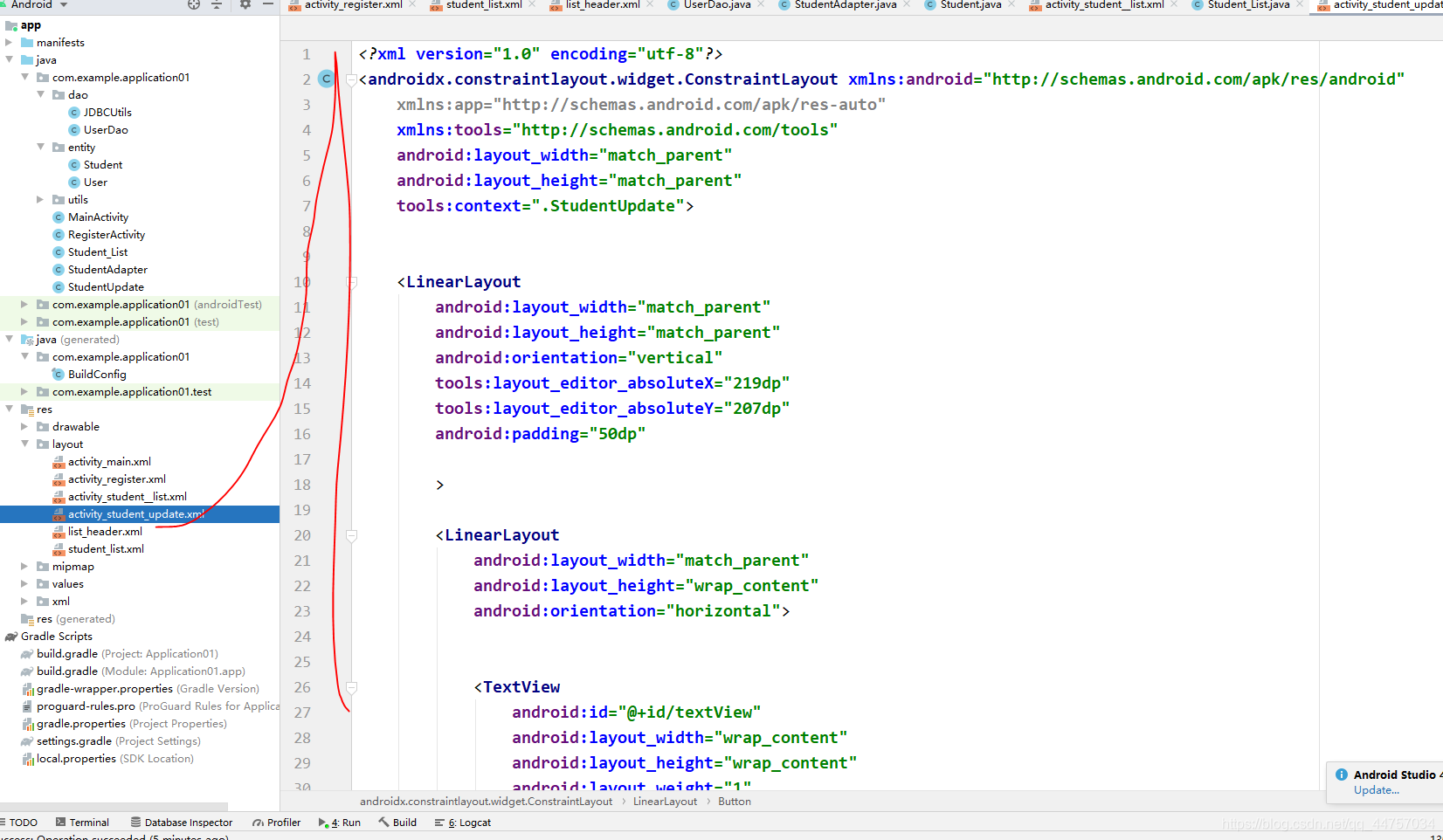
2、在student_list.xml页面上的按钮上添加onClick
3、完善Student_List当中的update方法
public void update(View view){ LinearLayout linearLayout = (LinearLayout)(view.getParent().getParent()); TextView idEd = linearLayout.findViewById(R.id.id); TextView nameEd = linearLayout.findViewById(R.id.name); TextView ageEd = linearLayout.findViewById(R.id.age); TextView addressEd = linearLayout.findViewById(R.id.address); Intent intent=new Intent(); intent.putExtra("id", idEd.getText().toString()); intent.putExtra("name", nameEd.getText().toString()); intent.putExtra("age", ageEd.getText().toString()); intent.putExtra("address", addressEd.getText().toString()); intent.setClass(this, StudentUpdate.class); startActivity(intent ); Toast.makeText(getApplicationContext(),"修改",Toast.LENGTH_LONG).show(); }
4、StudentUpdate当中获取对应Intent 对象当中的值并放入到页面当中的修改框当中
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_student_update); Intent intent = getIntent(); String id = intent.getStringExtra("id"); String name = intent.getStringExtra("name"); String age = intent.getStringExtra("age"); String address = intent.getStringExtra("address"); TextView idtv = findViewById(R.id.id); EditText nameet = findViewById(R.id.name); EditText ageet = findViewById(R.id.age); EditText addresset = findViewById(R.id.address); idtv.setText(id); nameet.setText(name); ageet.setText(age); addresset.setText(address); }
运行测试
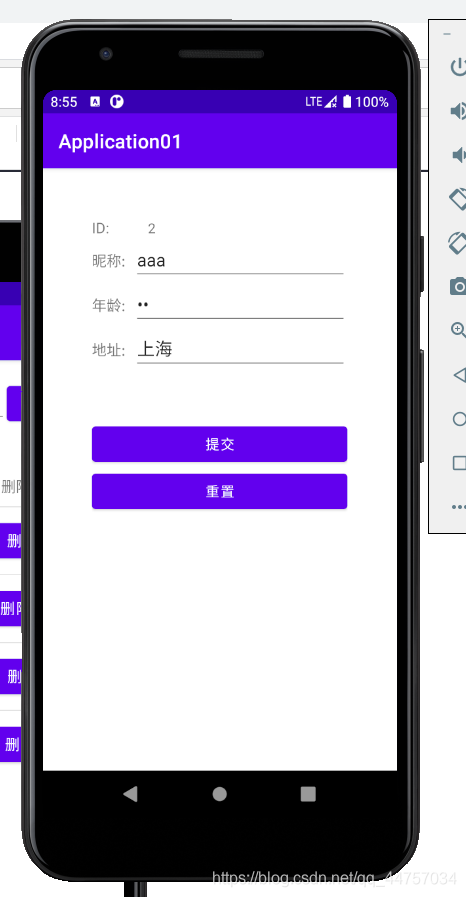
(二)web端
1、创建更新学生信息的dao
public boolean update(Student stu){ String sql="update student set name=?,age=?,address=? where id=?"; Connection con = JDBCUtils.getConn(); try { PreparedStatement pst=con.prepareStatement(sql); pst.setString(1,stu.getName()); pst.setInt(2,stu.getAge()); pst.setString(3,stu.getAddress() ); pst.setInt(4,stu.getId() ); int value = pst.executeUpdate(); if(value>0){ return true; } } catch (SQLException throwables) { throwables.printStackTrace(); }finally { JDBCUtils.close(con); } return false; }
2、创建对应更新学生信息的Servlet
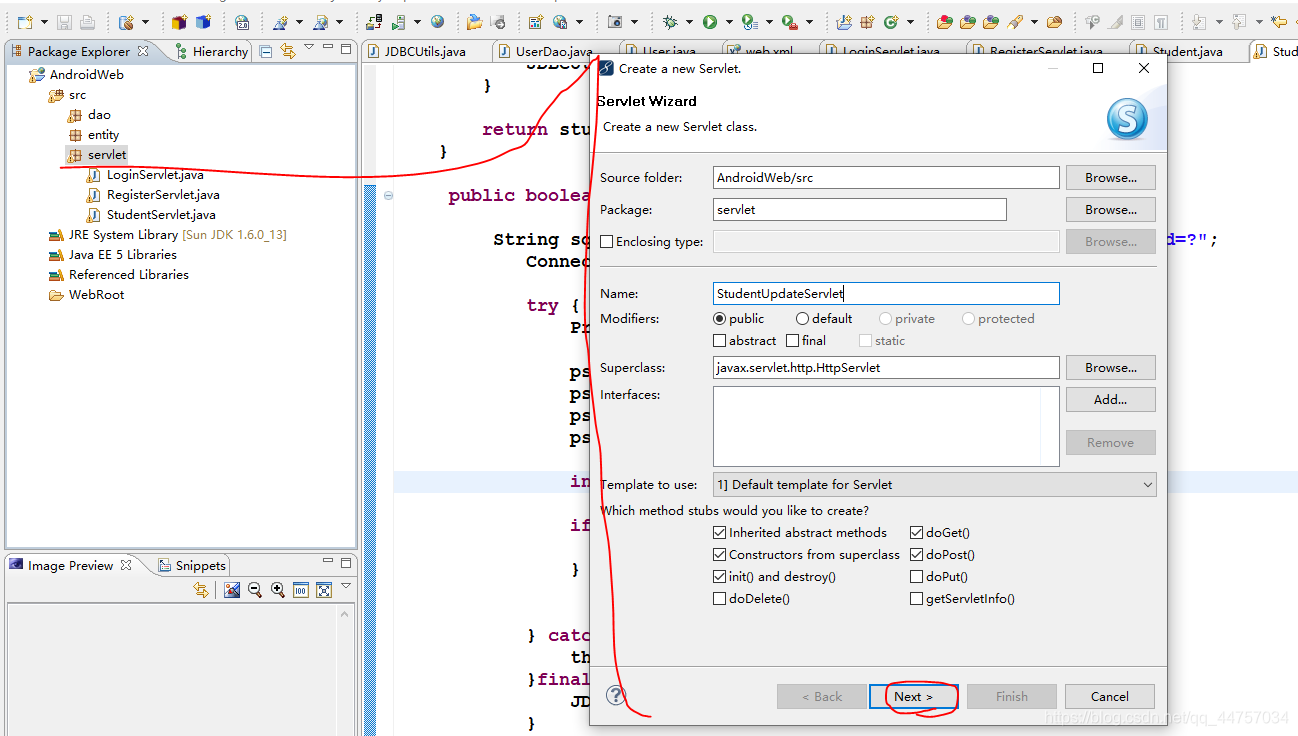
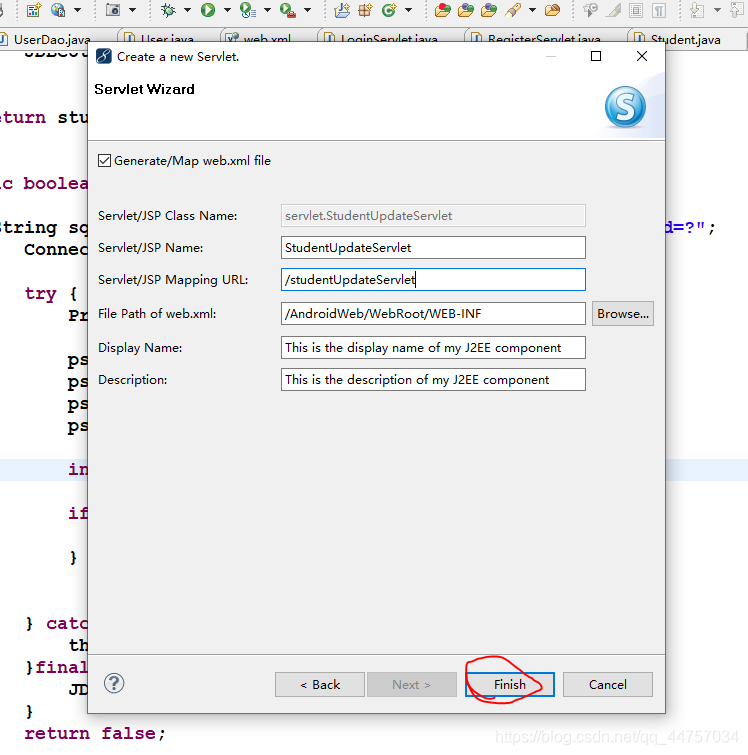
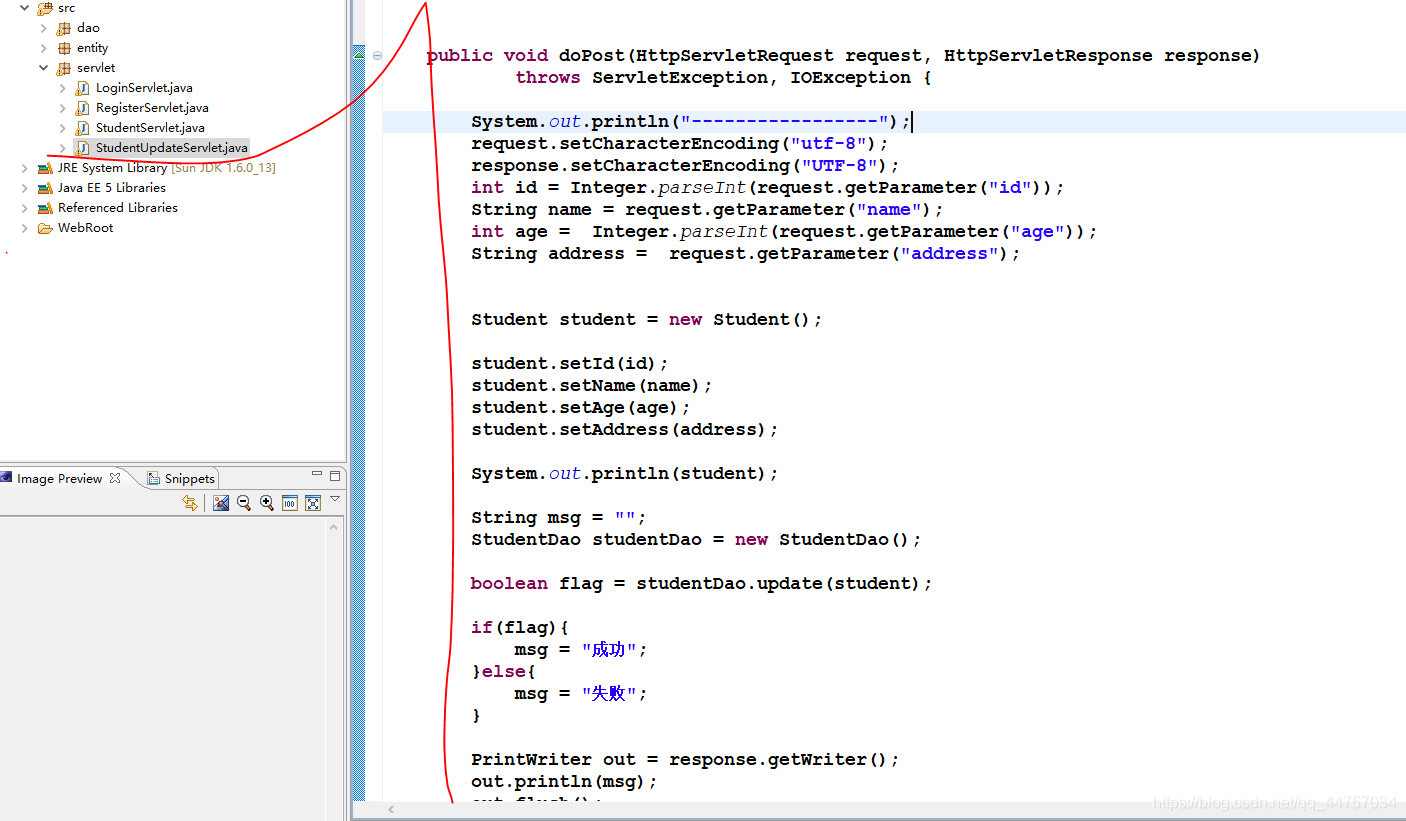
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { System.out.println("-----------------"); request.setCharacterEncoding("utf-8"); response.setCharacterEncoding("UTF-8"); int id = Integer.parseInt(request.getParameter("id")); String name = request.getParameter("name"); int age = Integer.parseInt(request.getParameter("age")); String address = request.getParameter("address"); Student student = new Student(); student.setId(id); student.setName(name); student.setAge(age); student.setAddress(address); String msg = ""; StudentDao studentDao = new StudentDao(); boolean flag = studentDao.update(student); if(flag){ msg = "成功"; }else{ msg = "失败"; } PrintWriter out = response.getWriter(); out.println(msg); out.flush(); out.close(); }
(三)安卓端
1、在StudentUpdate当中创建updatestudent实现向studentUpdateServlet发送更新信息的请求
package com.example.application01;import androidx.appcompat.app.AppCompatActivity;import android.content.Intent;import android.os.Bundle;import android.os.Handler;import android.os.Message;import android.view.LayoutInflater;import android.view.View;import android.widget.EditText;import android.widget.ListView;import android.widget.TextView;import android.widget.Toast;import com.example.application01.utils.PostUtil;import java.io.UnsupportedEncodingException;import java.net.URLEncoder;public class StudentUpdate extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_student_update); Intent intent = getIntent(); String id = intent.getStringExtra("id"); String name = intent.getStringExtra("name"); String age = intent.getStringExtra("age"); String address = intent.getStringExtra("address"); TextView idtv = findViewById(R.id.id); EditText nameet = findViewById(R.id.name); EditText ageet = findViewById(R.id.age); EditText addresset = findViewById(R.id.address); idtv.setText(id); nameet.setText(name); ageet.setText(age); addresset.setText(address); } public void updatestudent(View view){ TextView idtv = findViewById(R.id.id); EditText nameet = findViewById(R.id.name); EditText ageet = findViewById(R.id.age); EditText addresset = findViewById(R.id.address); String id = idtv.getText().toString(); String name = nameet.getText().toString(); String age = ageet.getText().toString(); String address = addresset.getText().toString(); new Thread(){ @Override public void run() { String data=""; try { data = "&id="+ URLEncoder.encode(id, "UTF-8")+ "&name="+ URLEncoder.encode(name, "UTF-8")+ "&age="+ URLEncoder.encode(age, "UTF-8")+ "&address="+ URLEncoder.encode(address, "UTF-8"); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } String request = PostUtil.Post("studentUpdateServlet",data); int msg = 0; if(request.equals("成功")){ msg = 1; } hand.sendEmptyMessage(msg); } }.start(); } Handler hand=new Handler(){ @Override public void handleMessage(Message msg) { if(msg.what == 1) { startActivity(new Intent( getApplicationContext(), Student_List.class ) ); //startActivity(new Intent( getApplicationContext(), StuInfoActivity.class ) ); } else { Toast.makeText(getApplicationContext(),"更新失败",Toast.LENGTH_LONG).show(); } } };}
2、运行测试
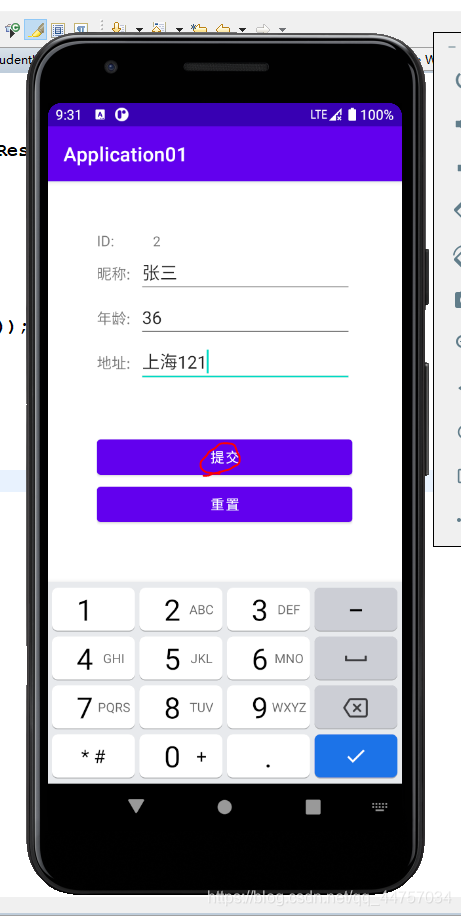
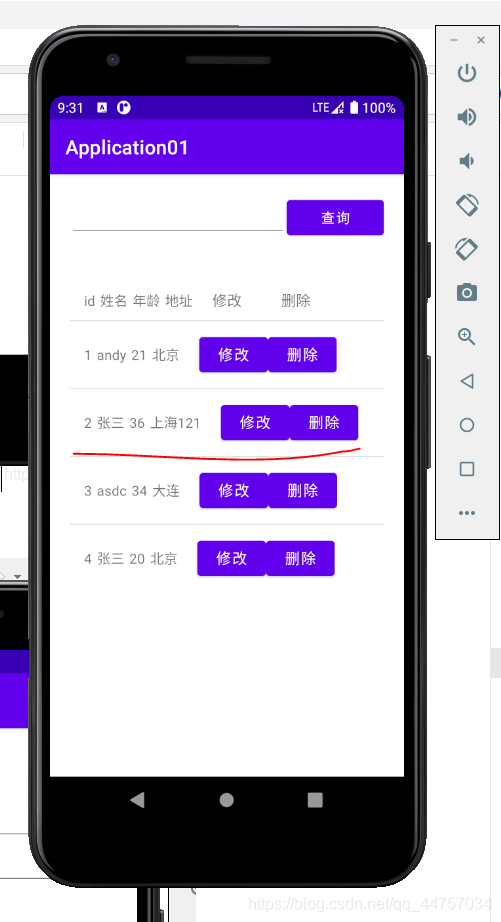
转载地址:https://code100.blog.csdn.net/article/details/116993494 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
能坚持,总会有不一样的收获!
[***.219.124.196]2024年04月03日 18时21分57秒
关于作者
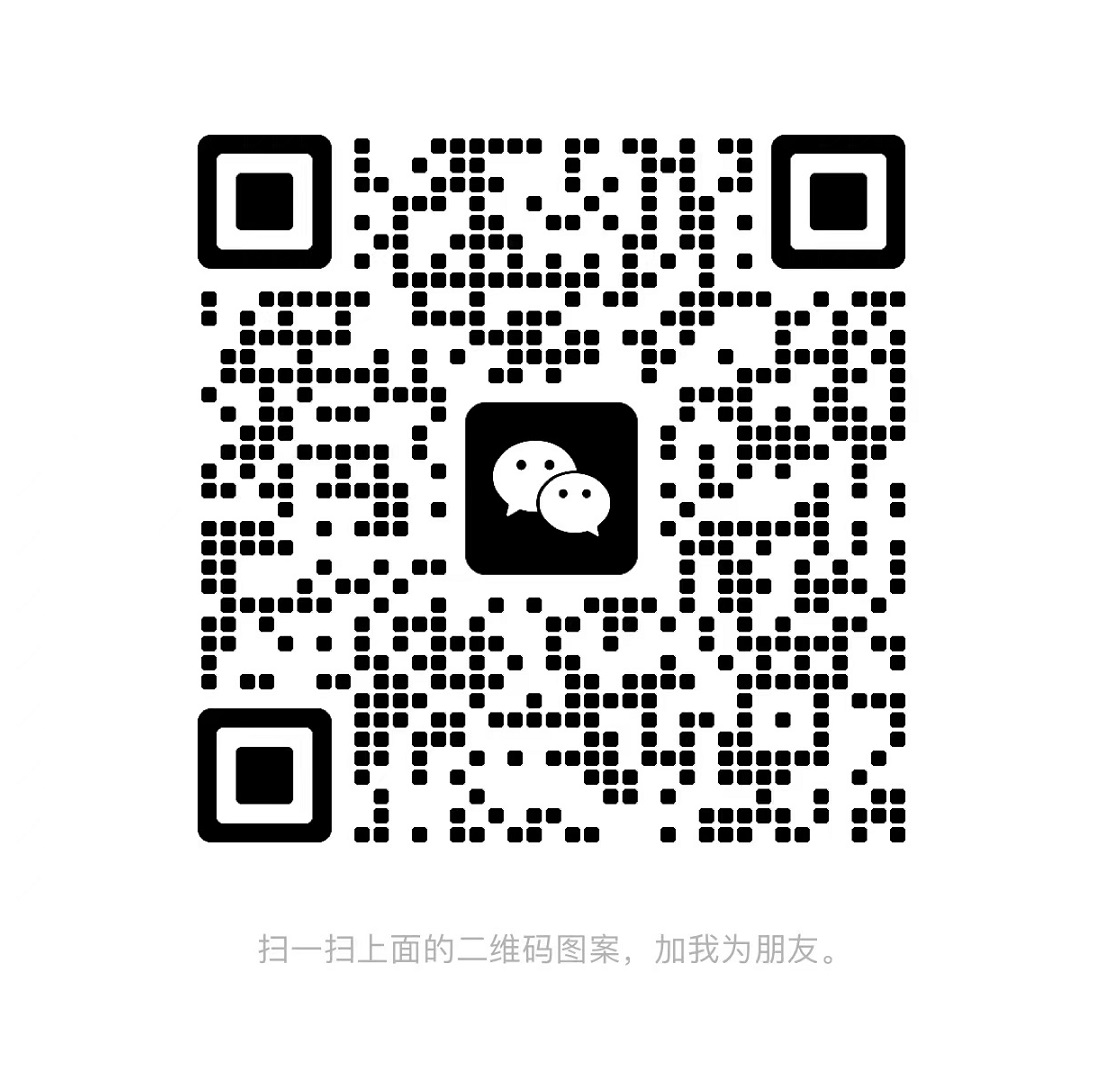
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
国会大厦骚乱,与一家极不可靠的面部识别公司……
2019-04-29
解锁宇宙密码:为什么是3、6、9?
2019-04-29
数据可视化中的格式塔心理学
2019-04-29
电动汽车的“专属危险”:网络威胁问题不容小觑
2019-04-29
短暂的告别,马上再回来
2019-04-29
统治50年:为什么SQL在如今仍然很重要?
2019-04-29
测试是一场竞争,而数据每次都会获得胜利
2019-04-29
读心的测谎系统:究竟是骗子还是个天才?
2019-04-29
最大规模技术重建:数据库连接从15000个到100个以下
2019-04-29
复工之后:员工如何改善网络安全?
2019-04-29
70%求职者因此被拒,你还不避开这些“雷区”?!
2019-04-29
办法不在多,有用就行!用Dropout解决过度拟合问题
2019-04-29
色情演员识别?绝对是人脸识别最糟糕的应用……
2019-04-29
让强化学习逃离“乏味区域陷阱”,试着加点噪音吧!
2019-04-29
超详细Spring Boot面试问题集锦,死角一个不留!
2019-04-29
10个业余时间可完成的项目,助你飞速提升编码能力!
2019-04-29
用深度学习打造艺术大师:照片变身的“魔法”
2019-04-29
必看!今年最火的五大Python框架
2019-04-29
掌握代码背后的这种语言,让你一招通吃天下!
2019-04-29
最终榜单!2019年人工智能的15个热门趋势
2019-04-29