
Python类|实例|方法|继承_专题_CodingPark编程公园
发布日期:2021-06-29 15:47:24
浏览次数:2
分类:技术文章
本文共 5595 字,大约阅读时间需要 18 分钟。
类
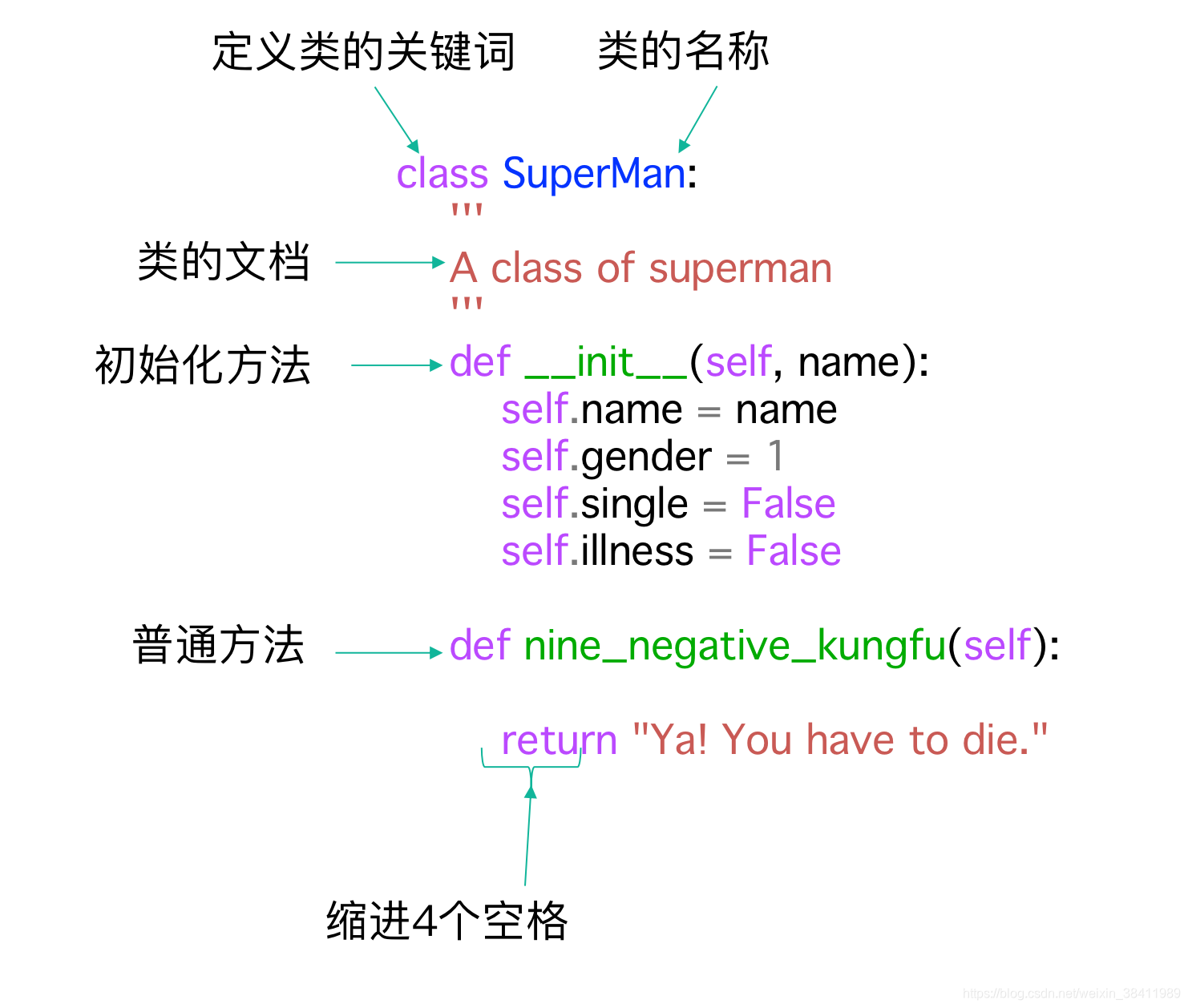
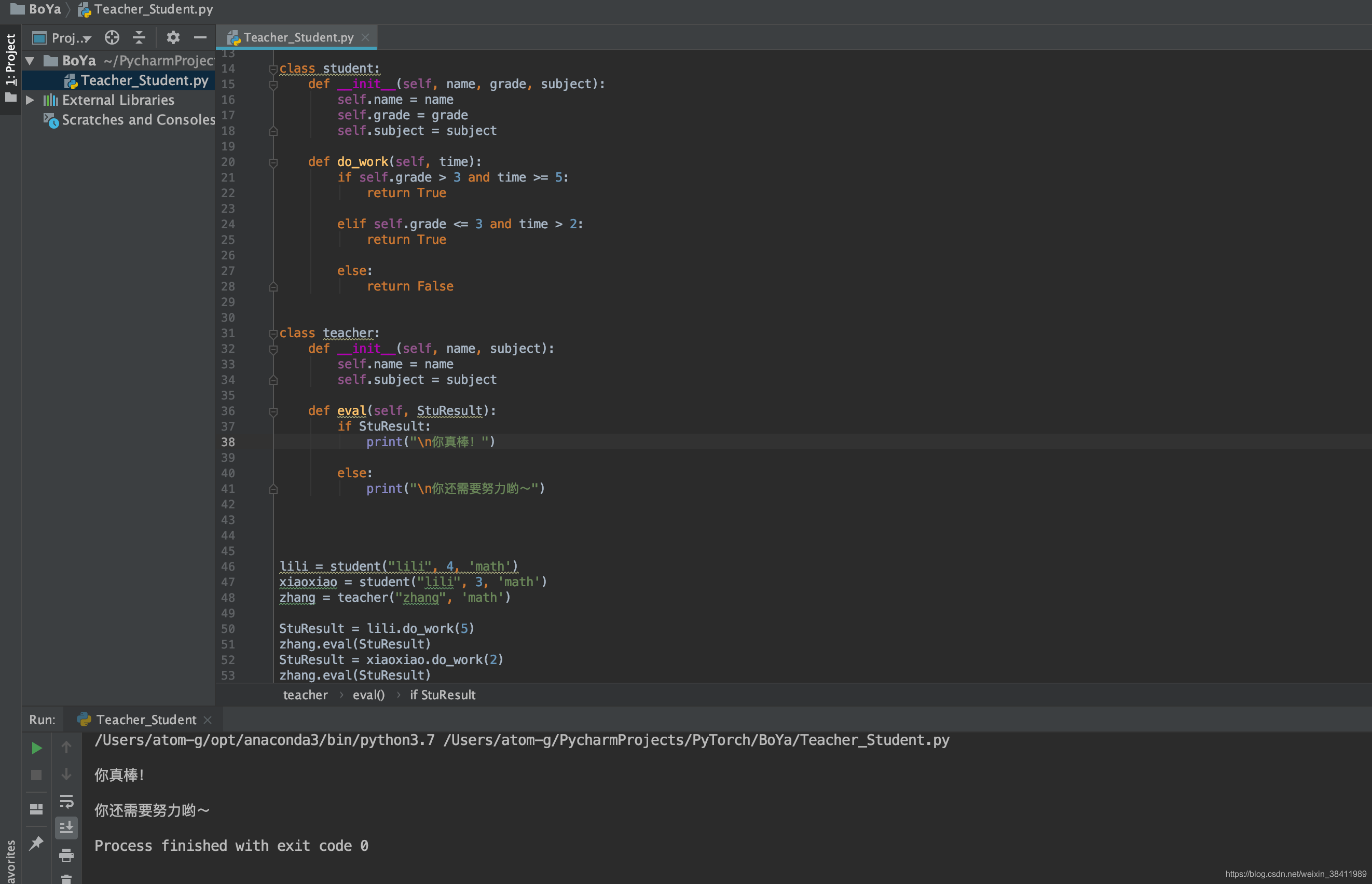
# -*- encoding: utf-8 -*-"""@File : Teacher_Student.py @Contact : ag@team-ag.club@License : (C)Copyright 2019-2020, CodingPark@Modify Time @Author @Version @Desciption------------ ------- -------- -----------2020-08-28 15:20 AG 1.0 类学习"""class student: def __init__(self, name, grade, subject): self.name = name self.grade = grade self.subject = subject def do_work(self, time): if self.grade > 3 and time >= 5: return True elif self.grade <= 3 and time > 2: return True else: return Falseclass teacher: def __init__(self, name, subject): self.name = name self.subject = subject def eval(self, StuResult): if StuResult: print("\n你真棒!") else: print("\n你还需要努力哟~")lili = student("lili", 4, 'math')xiaoxiao = student("lili", 3, 'math')zhang = teacher("zhang", 'math')StuResult = lili.do_work(5)zhang.eval(StuResult)StuResult = xiaoxiao.do_work(2)zhang.eval(StuResult)
实例
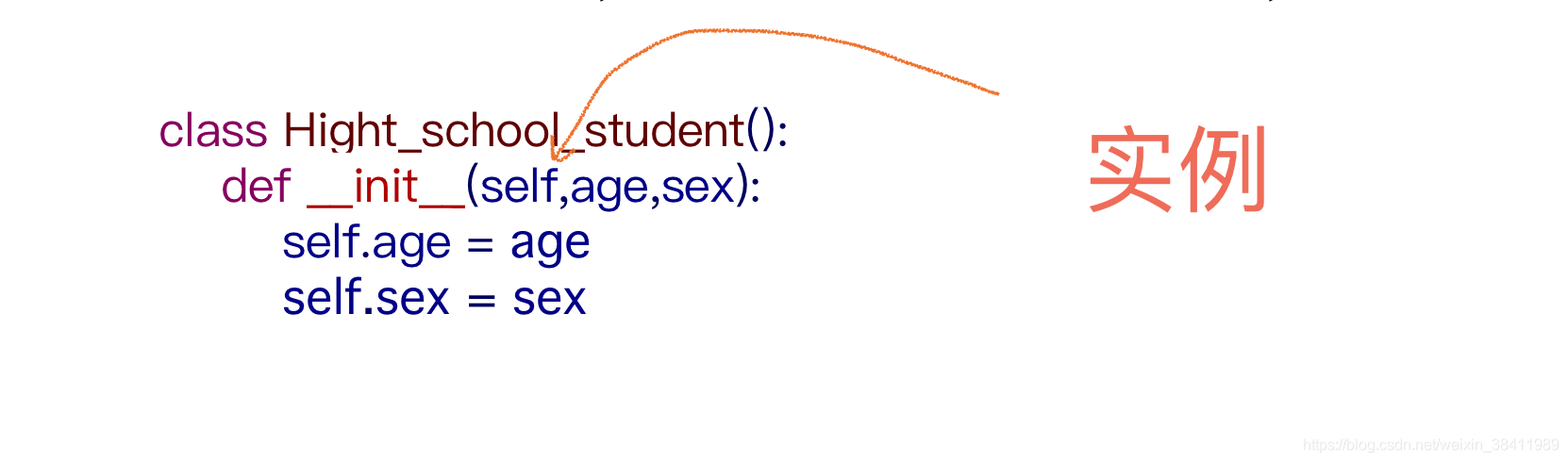
方法
- 静态方法在程序开始时生成内存,实例方法在程序运行中生成内存,所以静态方法可以直接调用。
- 实例方法要先生成实例,通过实例调用方法,静态速度很快,但是多了会占内存。
- 静态内存是连续的,因为是在程序开始时就生成了,而实例申请的是离散的空间,所以当然没有静态方法快,而且静态内存是有限制的,太多了程序会启动不了。
- 类方法常驻内存,实例方法不是,所以类方法效率高但占内存。
- 类方法在堆上分配内存,实例方法在堆栈上。
class Message: msg = "Python is a smart language" def get_msg(self): print('the selg is :', self) print('attrs of class(Message.msg):', Message.msg) @classmethod def get_cls_msg(cls): ''' 需要频繁使用时,使用了类Function ''' print('the selg is :', cls) print('attrs of class(Message.msg):', cls.msg)mess = Message()mess.get_msg()print('----------')mess.get_cls_msg()
结果展示
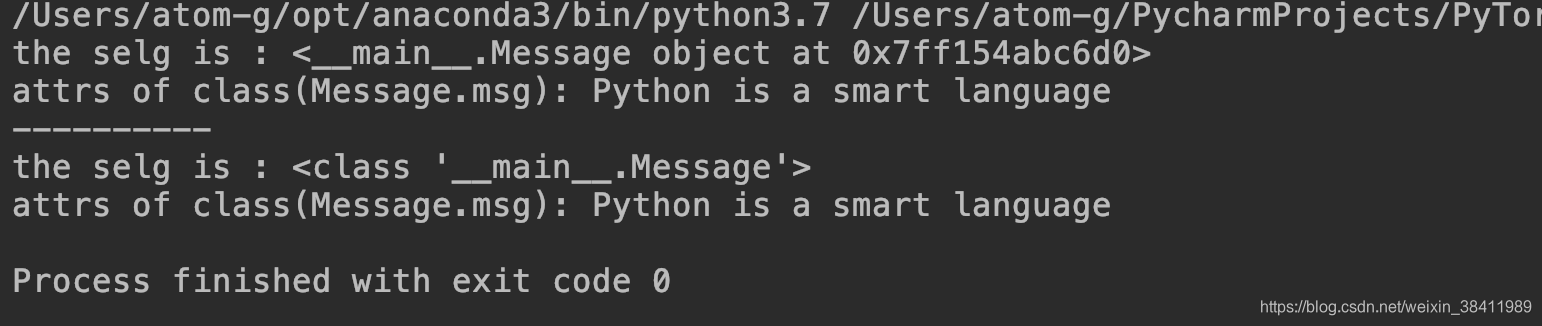
# -*- encoding: utf-8 -*-"""@File : run1.py @Contact : ag@team-ag.club@License : (C)Copyright 2019-2020, CodingPark@Modify Time @Author @Version @Desciption------------ ------- -------- -----------2020-08-29 11:42 AG 1.0 None"""class Cat: ''' 共有的写在这里「类属性」 ''' ears = 2 legs = 4 def __init__(self, color): ''' 私有的写在这里 :param color: ''' self.color = color @staticmethod # 静态方法 def speak(): print("Meow,Meow ~ ")bla_cat = Cat('black')whi_cat = Cat('white')print(bla_cat.color)print(whi_cat.color)bla_cat.speak()whi_cat.speak()print(bla_cat.speak is whi_cat.speak)
结果展示
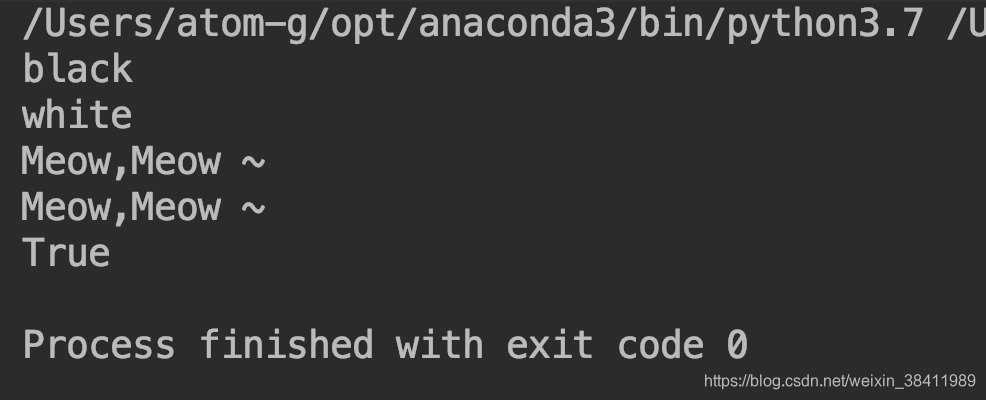
在一个类中,调用自己的函数
继承
简单直接的继承
这里Fruit为父类,Apple和Orange为子类,子类继承了父类的特性,因此Apple和Orange也拥有Color方法。
class Fruit(): def color(self): print("colorful")class Apple(Fruit): passclass Orange(Fruit): passapple = Apple()orange = Orange()apple.color()orange.color()# 输出# colorful# colorful
方法覆盖的继承
子类覆盖了父类的方法
class Fruit(): def color(self): print("colorful")class Apple(Fruit): def color(self): print("red")class Orange(Fruit): def color(self): print("orange")apple = Apple()orange = Orange()apple.color()orange.color()# 输出# red# orange
方法重构的继承
- super( )
子类可以在继承父类方法的同时,对方法进行重构。这样一来,子类的方法既包含父类方法的特性,同时也包含子类自己的特性
快速书写: 直接按黄色那么写
深入讲解
class Fruit(): def color(self): print("Fruits are colorful")class Apple(Fruit): def color(self): super().color() print("Apple is red")class Orange(Fruit): def color(self): super(Orange, self).color() print("Orange is orange")apple = Apple()orange = Orange()apple.color()orange.color()# 输出# Fruits are colorful# Apple is red# Fruits are colorful# Orange is orange
初始化函数继承
如果在子类中也定义了_init_()函数,那么该如何调用基类的_init_()函数
方法一、明确指定 :class C(P): def __init__(self): P.__init__(self) print 'calling Cs construtor'
方法二、使用super()方法 :
super()的好处就是可以避免直接使用父类的名字
class C(P): def __init__(self): super(C,self).__init__() print 'calling Cs construtor' c=C()
举个例子🌰
class Fruit: def __init__(self, color, shape): self.color = color self.shape = shapeclass Apple(Fruit): def __init__(self, color, shape, taste): Fruit.__init__(self, color, shape) # 等价于super().__init__(color, shape) self.taste = taste def feature(self): print("Apple's color is {}, shape is {} and taste {}".format( self.color, self.shape, self.taste))class Orange(Fruit): def __init__(self, color, shape, taste): super(Orange, self).__init__(color, shape) self.taste = taste def feature(self): print("Orange's color is {}, shape is {} and taste {}".format( self.color, self.shape, self.taste))apple = Apple("red", "square", "sour")orange = Orange("orange", "round", "sweet")apple.feature()orange.feature()# 输出# Apple's color is red, shape is square and taste sour# Orange's color is orange, shape is round and taste sweet
Python中多类继承
在单类继承中,super()函数用于指向要继承的父类,且不需要显式的写出父类名称。但是在多类继承中,会涉及到查找顺序(MRO)、钻石继承等问题。MRO 是类的方法解析顺序表, 也就是继承父类方法时的顺序表。钻石继承:
A / \ B C \ / D
如图所示,A是父类,B和C继承A,D继承B和C。下面举例说明钻石继承的继承顺序:
class Plant(): def __init__(self): print("Enter plant") print("Leave plant")class Fruit(Plant): def __init__(self): print("Enter Fruit") super().__init__() print("Leave Fruit")class Vegetable(Plant): def __init__(self): print("Enter vegetable") super().__init__() print("Leave vegetable")class Tomato(Fruit, Vegetable): def __init__(self): print("Enter Tomato") super().__init__() print("Leave Tomato")tomato = Tomato()print(Tomato.__mro__)# 输出# Enter Tomato# Enter Fruit# Enter vegetable# Enter plant# Leave plant# Leave vegetable# Leave Fruit# Leave Tomato# (, , , , )
转载地址:https://codingpark.blog.csdn.net/article/details/108359909 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关注你微信了!
[***.104.42.241]2024年04月28日 05时37分39秒
关于作者
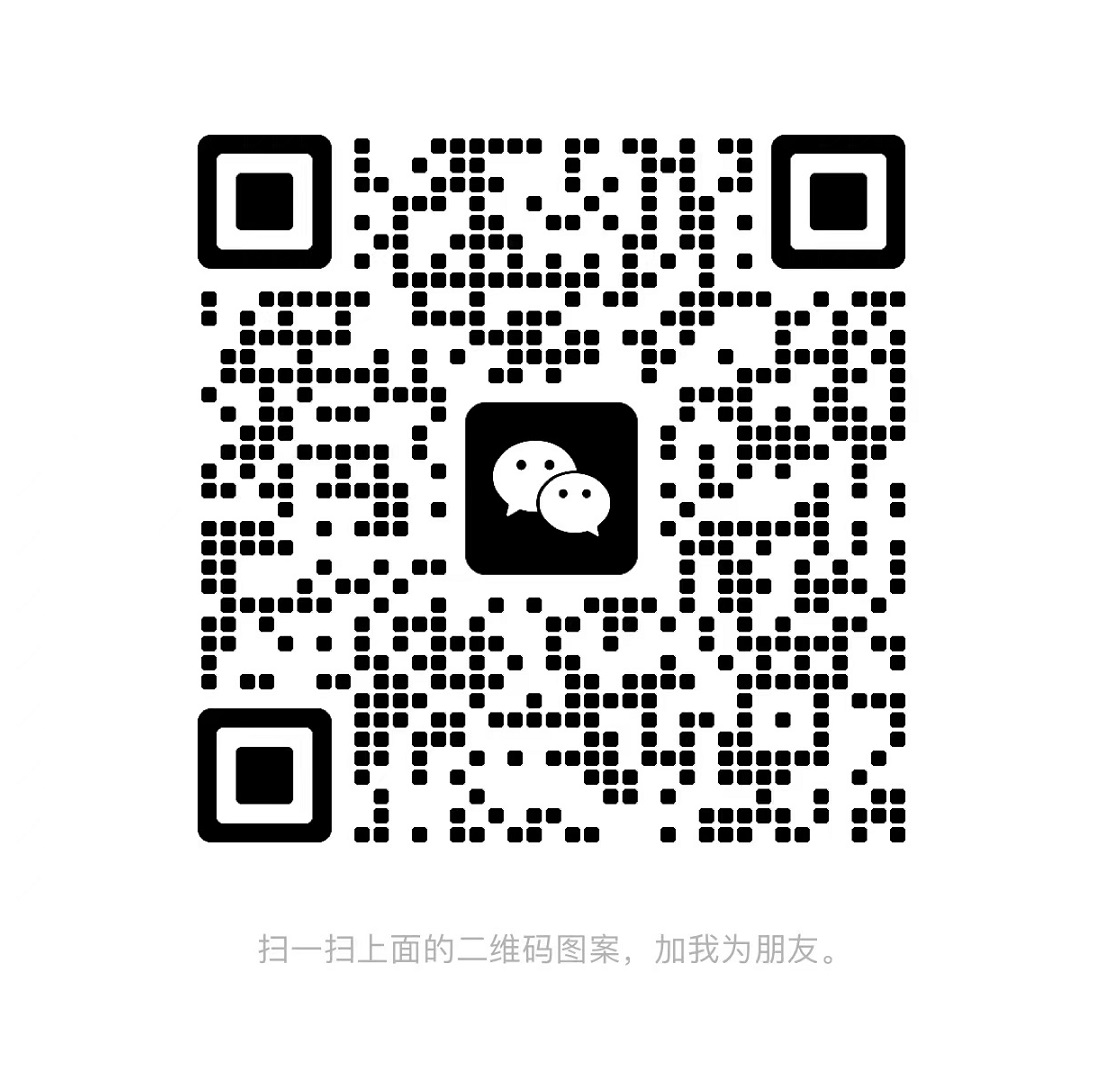
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
Python抓取哔哩哔哩up主信息:只要爬虫学的好,牢饭吃的早
2019-04-29
有个码龄5年的程序员跟我说:“他连wifi从来不用密码”
2019-04-29
领导让我整理上个季度的销售额,幸好我会Python数据分析,你猜我几点下班
2019-04-29
【Python爬虫实战】为何如此痴迷Python?还不是因为爱看小姐姐图
2019-04-29
零基础自学Python,你也可以实现经济独立!
2019-04-29
数字化转型的主干道上,华为云以“三大关键”成企业智能化推手
2019-04-29
数字化为何不走“捷”“径”?
2019-04-29
和总裁、专家交朋友,华为云助推政企智能化升级又做到前面去了
2019-04-29
BCOP章鱼船长,6月22日晚上8点上线薄饼
2019-04-29
为战疫助力,半导体功不可没
2019-04-29
了解这些操作,Python中99%的文件操作都将变得游刃有余!
2019-04-29
知道如何操作还不够!深入了解4大热门机器学习算法
2019-04-29
只有经历过,才能深刻理解的9个编程道理
2019-04-29
发现超能力:这些数据科学技能助你更高效专业
2019-04-29
AI当道,人工智能将如何改变金融业?
2019-04-29
消除性别成见,技术领域需要更多“乘风破浪的姐姐”
2019-04-29
7行代码击败整个金融业,这对20多岁的爱尔兰兄弟是如何做到的?
2019-04-29
2020十大编程博客:私藏的宝藏编程语言博客大放送!
2019-04-29
编程中的角色选择:哪类工作角色最适合你?
2019-04-29