
Python100行-贪吃蛇小游戏
发布日期:2021-06-29 17:34:09
浏览次数:2
分类:技术文章
本文共 3715 字,大约阅读时间需要 12 分钟。
今天来尝试下用python写一个贪吃小游戏,哈哈哈哈,毕竟贪吃蛇,大家小时候都玩过,但现在却有机会自己实现一个!!!
效果图
绘制蛇
1.如何画turtle画一个正方形?
其实就是一个点,往右,往下,往左,往上,回到原点…
# 坐标,长度,颜色名称def square(x, y, size, color): import turtle turtle.up() turtle.goto(x, y) turtle.down() turtle.color(color) turtle.begin_fill() for count in range(4): turtle.forward(size) turtle.left(90) turtle.end_fill()
然后我们就可以画出一个正方啦。
2.画出多个连续的正方形,来模拟蛇的身子。
蛇的身子其实就是一个一个方块列表,所以我们来尝试画多个方块。
snake = [[0,0],[0,10]]for body in snake: square(body[0], body[1], 10, 'black')
移动蛇
1. 移动方向
蛇需要可以朝四个方向移动。
x,y代表坐标
- (10,0):代表向右移动
- (-10,0):代表想左移动
- (0,10):代表向上移动
- (0,-10):代表向下移动
aim = [0, 10]# 设置方向def change_direction(x, y): aim[0] = x aim[1] = y
2. 移动逻辑
有了移动方向,我们就可以开始写移动的逻辑啦
思路是这样的:我们把列表看成一条蛇,这条蛇的右边在右边,尾部在左边!
- 移动时,我们消除尾部的一个方块。
- 根据方向,在头部添加一个方块。
- 然后在刷新动画。
- 就可以完成蛇移动的效果啦。
下面是代码
import copydef snake_move(): # 获取蛇头 head = [snake[-1][0],snake[-1][1]] # 最后一个加方向 head = [head[0] + aim[0], head[1] + aim[1]] # 加过后还在蛇里面,不在画布里面 snake.append(head) # 蛇的头部添加这个新的方块 snake.pop(0) # 蛇的尾部,去除一个方块 turtle.clear()# 清除方块 # 重新画一遍蛇的整个身子 for body in snake: square(body[0], body[1], 10, 'black') turtle.update()# 更新动画 turtle.ontimer(snake_move, 300)turtle.hideturtle()turtle.tracer(False)
3. 移动蛇
现在蛇就可以移动啦,但是我们还不能控制它的方向!
我们来监听键盘的按键,用上下左右来控制蛇的移动!
turtle.listen()turtle.onkey(lambda: change_direction(10, 0), "Right") # 右turtle.onkey(lambda: change_direction(-10, 0), "Left") # 左turtle.onkey(lambda: change_direction(0, 10), "Up") # 上turtle.onkey(lambda: change_direction(0, -10), "Down") # 下
食物
设置随机产生食物
首先一个食物被吃掉时,我们就在一个指定的区间里,随机产生食物。
# 如果蛇头吃到了食物,我们就不删除蛇的尾巴最后一个方块if head == food: print("snake的长度", len(snake)) food[0] = randrange(-15, 15)*10 # 设置食物的区间,必须是10的倍数 food[1] = randrange(-15, 15)*10else: snake.pop(0) # 蛇的尾部,去除一个方块
边界
设置边界条件
当蛇碰到自己或者当蛇碰到边界的时候,我们就算输啦!!
# 先给屏幕设定一个初始值500*500turtle.setup(500, 500)# 设置边界条件判断def inside(head): return -250 < head[0] < 250 and -250 < head[1] < 250# 在蛇移动的过程中进行判断,如果发生碰撞我们就把头部标记为红色,结束游戏。 if head in snake or not inside(head): print(head) square(head[0], head[1], 10, 'red') turtle.update()
全部完整代码
import turtlefrom random import randrangesnake = [[0, 0]]aim = [0, 10]food = [-10, 0]def change_direction(x, y): aim[0] = x aim[1] = ydef sqaure(x, y, size, color): turtle.penup() turtle.goto(x, y) turtle.pendown() turtle.begin_fill() turtle.color(color) for i in range(4): turtle.forward(size) turtle.left(90) turtle.end_fill()import copydef inside(head): return -250 <250 and -250 <250def snake_move(): #head = snake[-1][:] # 获取蛇头 head = [snake[-1][0],snake[-1][1]] # 最后一个加方向 head = [head[0] + aim[0], head[1] + aim[1]] # 加过后还在蛇里面,不在画布里面 if head in snake or not inside(head): # 红色 sqaure(head[0],head[1],10,'red') turtle.update() return if head == food: # 遇到食物 print("snake", len(snake)) food[0] = randrange(-15, 15) * 10 food[1] = randrange(-15, 15) * 10 else: snake.pop(0) # 删除蛇尾 snake.append(head) turtle.clear() sqaure(food[0], food[1], 10, "green") for body in snake: sqaure(body[0], body[1], 10, "black") turtle.update() turtle.ontimer(snake_move, 300)turtle.setup(500,500)turtle.hideturtle()turtle.listen()turtle.onkey(lambda: change_direction(0, 10), "Up")turtle.onkey(lambda: change_direction(0, -10), "Down")turtle.onkey(lambda: change_direction(-10, 0), "Left")turtle.onkey(lambda: change_direction(10, 0), "Right")turtle.tracer(False)snake_move()turtle.done()
结尾
这样我们就我完成了一个简单的贪吃蛇的游戏啦,我们还可以调成速度,记录分数,还有更多的功能,大家可以自行添加。
视频教程
参考
黄九九 https://zhuanlan.zhihu.com/p/102504395
转载地址:https://cpython.blog.csdn.net/article/details/111714954 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
不错!
[***.144.177.141]2024年04月16日 08时01分41秒
关于作者
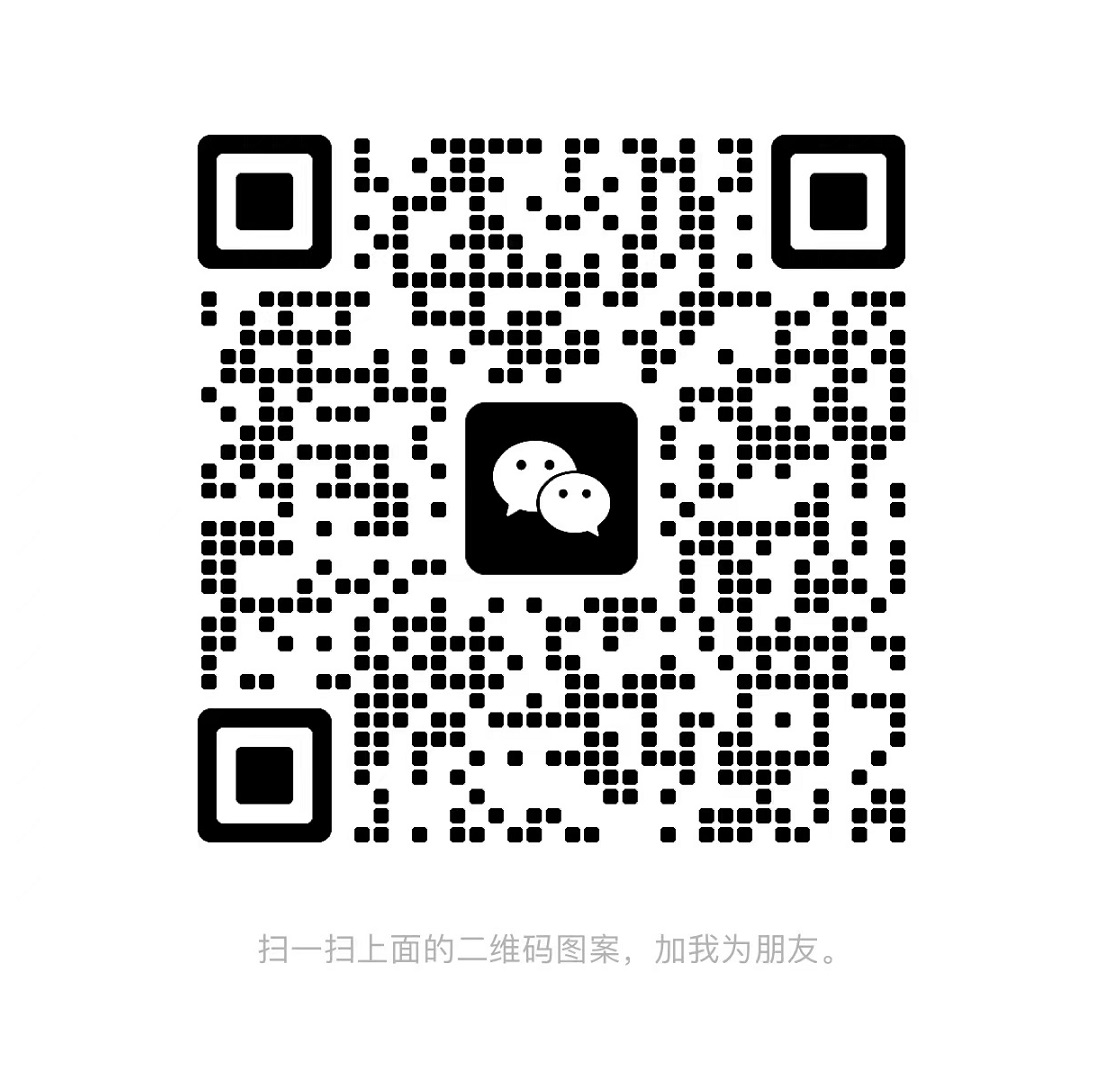
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
基于CH568芯片的SATA电子盘方案
2019-04-29
linux下C语言判断网络是否连接
2019-04-29
2021/4/27课堂总结和作业
2019-04-29
2021.4.28课堂总结和作业
2019-04-29
2021.4.29课堂总结
2019-04-29
2021.4.30课堂总结和作业
2019-04-29
需要吗?2000GB+学习视频教程 面试资料免费下载
2019-04-29
MySQL对已存在数据库表添加自增ID字段
2019-04-29
idea中的一些常用快捷键
2019-04-29
js校验表单后提交表单的三种方法总结【转载】
2019-04-29
欢迎使用CSDN-markdown编辑器
2019-04-29
a标签中href调用js的几种方法
2019-04-29
jstl标签详解
2019-04-29
Eclipse中使用SVN的使用
2019-04-29
JSON.parse和eval的区别
2019-04-29
JQuery中$.ajax()方法参数详解
2019-04-29
正则表达式的数字实例
2019-04-29
【转】EasyUI 验证
2019-04-29