
本文共 6651 字,大约阅读时间需要 22 分钟。
点击上方“程序IT圈”,选择“置顶公众号”
原创:一个程序员的成长 | 作者:冰峰
SpringBoot现在是很多很多公司应用的后端框架,因为它搭建快,能更好、更快速的整合其他第三方。那么随着业务的不断扩展,业务量的增加,这时候就会牵扯到分库分表,虽然这个词听起来很熟悉,作为程序员也很容易理解,但是我想应该也有不少读者没接触过分库分表,今天我们不聊如何分库分表,而是聊SpringBoot如何整合多个数据源的事情。也就是如何接入不同的(多个)数据库。
我们直接开始,我们直接创建一个干净的SpringBoot应用。
org.springframework.boot spring-boot-starter-parent 2.1.0.RELEASE
org.springframework.boot spring-boot-starter-web org.mybatis.spring.boot mybatis-spring-boot-starter 1.3.2 mysql mysql-connector-java runtime org.springframework.boot spring-boot-starter-jdbc org.projectlombok lombok 1.18.8
引入需要的maven坐标,那么我们这个工程就算搭建起来了,接下来就是配置,如何让SpringBoot整合两个Mysql数据源。首先我们在本地创建两个数据库test1和test2,同时在里面创建两个结构一样的表。
CREATE TABLE `user` ( `id` bigint(20) NOT NULL AUTO_INCREMENT COMMENT '用户ID', `username` varchar(100) CHARACTER SET utf8 NOT NULL COMMENT '用户名', `password` varchar(100) NOT NULL COMMENT '密码', `create_time` datetime DEFAULT NULL COMMENT '创建时间', PRIMARY KEY (`id`) ) ENGINE=MyISAM AUTO_INCREMENT=3 DEFAULT CHARSET=latin1;
在我们的工程中配置application.yml文件,将数据库的信息配置进去
spring: datasource: test1: driver-class-name: com.mysql.cj.jdbc.Driver jdbc-url: jdbc:mysql://localhost:3306/test1?useUnicode=true&characterEncoding=utf-8&useSSL=true&serverTimezone=UTC username: root password: 1234 test2: driver-class-name: com.mysql.cj.jdbc.Driver jdbc-url: jdbc:mysql://localhost:3306/test2?useUnicode=true&characterEncoding=utf-8&useSSL=true&serverTimezone=UTC username: root password: 1234
接下来就是写我们的配置类了,这也是整合多个数据源最为关键的部分。
import org.apache.ibatis.session.SqlSessionFactory; import org.mybatis.spring.SqlSessionFactoryBean; import org.mybatis.spring.SqlSessionTemplate; import org.mybatis.spring.annotation.MapperScan; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.boot.jdbc.DataSourceBuilder; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Primary; import org.springframework.jdbc.datasource.DataSourceTransactionManager; import javax.sql.DataSource; /** * @ClassName DataSource2Config * @Description TODO * @Auther lbt * @Date 2019/6/28/028 10:07 * @Version 1.0 */ @Configuration @MapperScan(basePackages = "com.example.mapper.test1", sqlSessionFactoryRef = "test1SqlSessionFactory") public class DataSource1Config { @Bean(name = "test1DataSource") @ConfigurationProperties(prefix = "spring.datasource.test1") @Primary public DataSource test1DataSource() { return DataSourceBuilder.create().build(); } @Bean(name = "test1SqlSessionFactory") @Primary public SqlSessionFactory test1SqlSessionFactory(@Qualifier("test1DataSource") DataSource dataSource) throws Exception { SqlSessionFactoryBean bean = new SqlSessionFactoryBean(); bean.setDataSource(dataSource); return bean.getObject(); } @Bean(name = "test1TransactionManager") @Primary public DataSourceTransactionManager test1TransactionManager(@Qualifier("test1DataSource") DataSource dataSource) { return new DataSourceTransactionManager(dataSource); } @Bean(name = "test1SqlSessionTemplate") @Primary public SqlSessionTemplate test1SqlSessionTemplate(@Qualifier("test1SqlSessionFactory") SqlSessionFactory sqlSessionFactory) { return new SqlSessionTemplate(sqlSessionFactory); } }
第二个数据源的配置
import org.apache.ibatis.session.SqlSessionFactory; import org.mybatis.spring.SqlSessionFactoryBean; import org.mybatis.spring.SqlSessionTemplate; import org.mybatis.spring.annotation.MapperScan; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.boot.jdbc.DataSourceBuilder; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.jdbc.datasource.DataSourceTransactionManager; import javax.sql.DataSource; @Configuration @MapperScan(basePackages = "com.example.mapper.test2", sqlSessionFactoryRef = "test2SqlSessionFactory") public class DataSource2Config { @Bean(name = "test2DataSource") @ConfigurationProperties(prefix = "spring.datasource.test2") public DataSource test2DataSource() { return DataSourceBuilder.create().build(); } @Bean(name = "test2SqlSessionFactory") public SqlSessionFactory test2SqlSessionFactory(@Qualifier("test2DataSource") DataSource dataSource) throws Exception { SqlSessionFactoryBean bean = new SqlSessionFactoryBean(); bean.setDataSource(dataSource); return bean.getObject(); } @Bean(name = "test2TransactionManager") public DataSourceTransactionManager test2TransactionManager(@Qualifier("test2DataSource") DataSource dataSource) { return new DataSourceTransactionManager(dataSource); } @Bean(name = "test2SqlSessionTemplate") public SqlSessionTemplate test2SqlSessionTemplate(@Qualifier("test2SqlSessionFactory") SqlSessionFactory sqlSessionFactory) { return new SqlSessionTemplate(sqlSessionFactory); } }
这样我们整个的配置其实就算好了,我们接下来写一个Controller类来测试一下,我们整合的数据源是不是真的可以用呢?
@RestController public class TestController { @Autowired private User1Service user1Service; @Autowired private User2Service user2Service; @RequestMapping("/user1") public Object user1Controller() { Listall = user1Service.findAll(); return all; } @RequestMapping("/user2") public Object user2Controller() { List all = user2Service.findAll(); return all; } }
我写了个两个Controller方法,分别访问不同的接口,我们来看下访问结果。
当我们访问user1的时候返回如下:
当我们访问user2的时候访问如下
看到这里其实我们的整个整合也就完成了, 虽然看起来很简单,但是你如果没写过确实会走很多坑,我刚整合的时候就遇到了很多坑,为了帮助大家重复采坑,分享出来供大家参考,另外我已经上传GitHub,大家可以直接拉下来跑。
GitHub地址:
https://github.com/liangbintao/springboot_datasources.git
作者是一个转行过来的程序员,在学习的过程中碰了太多了的坑,走了很多弯路,我的很多读者也是刚转行过来的,也有很多学生,我把的学习过程中遇到的问题已经一些经验,分享在我的公众号,有思路有代码,做一个你肯定看的懂的公众平台。
~完~
打卡赠书:
送什么书?请点击左下角阅读原文查看!
签到方式:长按以下二维码参与打卡↓↓↓↓↓
欢迎转发,欢迎关注!
转载地址:https://cxydev.blog.csdn.net/article/details/95558163 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关于作者
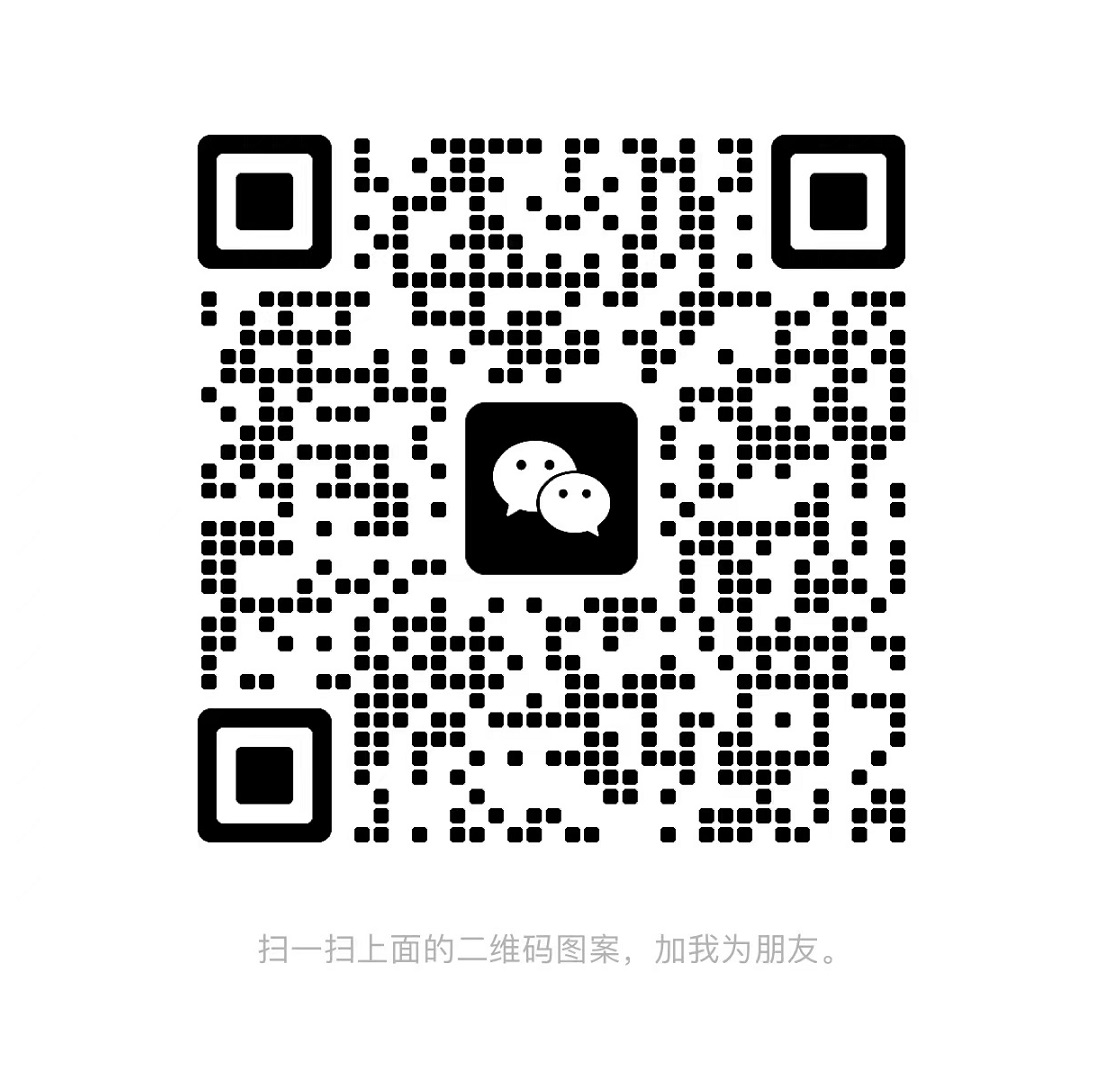