
安卓压缩/解压缩工具
发布日期:2021-06-29 18:29:30
浏览次数:2
分类:技术文章
本文共 8299 字,大约阅读时间需要 27 分钟。
public class ZipUtils { private static final int BUFF_SIZE = 1024 * 1024; // 1M Byte /** * 压缩目录 * * @param srcPath 被压缩的目录路径 * @param destZipFilePath 目标zip文件路径 * @param excludeTopDir 是否排除掉顶层目录 * @throws IOException */ public static void zip(String srcPath, String destZipFilePath, Boolean excludeTopDir) throws IOException { FileOutputStream fos = new FileOutputStream(destZipFilePath); ZipOutputStream zipOut = new ZipOutputStream(fos); File srcDirFile = new File(srcPath); if (srcDirFile.isDirectory()) { if (excludeTopDir) { zipDirExcludeTopDir(zipOut, srcDirFile); } else { zipFile(srcDirFile, srcDirFile.getName(), zipOut); } } else { zipFile(srcDirFile, srcDirFile.getName(), zipOut); } zipOut.close(); fos.close(); } /** * 压缩目录时,去掉最顶层的目录。 */ private static void zipDirExcludeTopDir(ZipOutputStream zipOut, File srcDir) throws IOException { File[] subFiles = srcDir.listFiles(); for (int i = 0; i < subFiles.length; i++) { File subFile = subFiles[i]; zipFile(subFile, subFile.getName(), zipOut); } } private static void zipFile(File fileToZip, String fileName, ZipOutputStream zipOut) throws IOException { if (fileToZip.isDirectory()) { if (fileName.endsWith("/")) { zipOut.putNextEntry(new ZipEntry(fileName)); zipOut.closeEntry(); } else { zipOut.putNextEntry(new ZipEntry(fileName + "/")); zipOut.closeEntry(); } File[] children = fileToZip.listFiles(); for (File childFile : children) { zipFile(childFile, fileName + "/" + childFile.getName(), zipOut); } return; } FileInputStream fis = new FileInputStream(fileToZip); ZipEntry zipEntry = new ZipEntry(fileName); zipOut.putNextEntry(zipEntry); byte[] bytes = new byte[1024]; int length; while ((length = fis.read(bytes)) >= 0) { zipOut.write(bytes, 0, length); } fis.close(); } /** * 解压缩一个文件 * * @param zipFile 压缩文件 * @param folderPath 解压缩的目标目录 * @throws IOException 当解压缩过程出错时抛出 */ public static void upZipFile(File zipFile, String folderPath) throws ZipException, IOException { File desDir = new File(folderPath); if (!desDir.exists()) { desDir.mkdirs(); } ZipFile zf = new ZipFile(zipFile); for (Enumeration entries = zf.entries(); entries.hasMoreElements(); ) { ZipEntry entry = ((ZipEntry) entries.nextElement()); if (entry.isDirectory()) { continue; } InputStream in = zf.getInputStream(entry); String str = folderPath + File.separator + entry.getName(); str = new String(str.getBytes(), "utf-8"); File desFile = new File(str); if (!desFile.exists()) { File fileParentDir = desFile.getParentFile(); if (!fileParentDir.exists()) { fileParentDir.mkdirs(); } desFile.createNewFile(); } OutputStream out = new FileOutputStream(desFile); byte buffer[] = new byte[BUFF_SIZE]; int realLength; while ((realLength = in.read(buffer)) > 0) { out.write(buffer, 0, realLength); } in.close(); out.close(); } } /** * 解压文件名包含传入文字的文件 * * @param zipFile 压缩文件 * @param folderPath 目标文件夹 * @param nameContains 传入的文件匹配名 * @throws ZipException 压缩格式有误时抛出 * @throws IOException IO错误时抛出 */ public static ArrayListupZipSelectedFile(File zipFile, String folderPath, String nameContains) throws ZipException, IOException { ArrayList fileList = new ArrayList (); File desDir = new File(folderPath); if (!desDir.exists()) { desDir.mkdir(); } ZipFile zf = new ZipFile(zipFile); for (Enumeration entries = zf.entries(); entries.hasMoreElements(); ) { ZipEntry entry = ((ZipEntry) entries.nextElement()); if (entry.getName().contains(nameContains)) { InputStream in = zf.getInputStream(entry); String str = folderPath + File.separator + entry.getName(); str = new String(str.getBytes("utf-8"), "gbk"); // str.getBytes("GB2312"),"8859_1" 输出 // str.getBytes("8859_1"),"GB2312" 输入 File desFile = new File(str); if (!desFile.exists()) { File fileParentDir = desFile.getParentFile(); if (!fileParentDir.exists()) { fileParentDir.mkdirs(); } desFile.createNewFile(); } OutputStream out = new FileOutputStream(desFile); byte buffer[] = new byte[BUFF_SIZE]; int realLength; while ((realLength = in.read(buffer)) > 0) { out.write(buffer, 0, realLength); } in.close(); out.close(); fileList.add(desFile); } } return fileList; } /** * 获得压缩文件内文件列表 * * @param zipFile 压缩文件 * @return 压缩文件内文件名称 * @throws ZipException 压缩文件格式有误时抛出 * @throws IOException 当解压缩过程出错时抛出 */ public static ArrayList getEntriesNames(File zipFile) throws ZipException, IOException { ArrayList entryNames = new ArrayList (); Enumeration entries = getEntriesEnumeration(zipFile); while (entries.hasMoreElements()) { ZipEntry entry = ((ZipEntry) entries.nextElement()); entryNames.add(new String(getEntryName(entry).getBytes("GB2312"), "8859_1")); } return entryNames; } /** * 获得压缩文件内压缩文件对象以取得其属性 * * @param zipFile 压缩文件 * @return 返回一个压缩文件列表 * @throws ZipException 压缩文件格式有误时抛出 * @throws IOException IO操作有误时抛出 */ public static Enumeration getEntriesEnumeration(File zipFile) throws ZipException, IOException { ZipFile zf = new ZipFile(zipFile); return zf.entries(); } /** * 取得压缩文件对象的名称 * * @param entry 压缩文件对象 * @return 压缩文件对象的名称 * @throws UnsupportedEncodingException */ public static String getEntryName(ZipEntry entry) throws UnsupportedEncodingException { return new String(entry.getName().getBytes("GB2312"), "8859_1"); } public static void unzip(String zipFileName, String outputDirectory) throws IOException { ZipFile zipFile = null; try { zipFile = new ZipFile(zipFileName); Enumeration e = zipFile.entries(); ZipEntry zipEntry = null; File dest = new File(outputDirectory); dest.mkdirs(); while (e.hasMoreElements()) { zipEntry = (ZipEntry) e.nextElement(); String entryName = zipEntry.getName(); InputStream in = null; FileOutputStream out = null; try { if (zipEntry.isDirectory()) { String name = zipEntry.getName(); name = name.substring(0, name.length() - 1); File f = new File(outputDirectory + File.separator + name); f.mkdirs(); } else { int index = entryName.lastIndexOf("\\"); if (index != -1) { File df = new File(outputDirectory + File.separator + entryName.substring(0, index)); df.mkdirs(); } index = entryName.lastIndexOf("/"); if (index != -1) { File df = new File(outputDirectory + File.separator + entryName.substring(0, index)); df.mkdirs(); } File f = new File(outputDirectory + File.separator + zipEntry.getName()); // f.createNewFile(); in = zipFile.getInputStream(zipEntry); out = new FileOutputStream(f); int c; byte[] by = new byte[1024]; while ((c = in.read(by)) != -1) { out.write(by, 0, c); } out.flush(); } } catch (IOException ex) { ex.printStackTrace(); throw new IOException("解压失败:" + ex.toString()); } finally { if (in != null) { try { in.close(); } catch (IOException ex) { } } if (out != null) { try { out.close(); } catch (IOException ex) { } } } } } catch (IOException ex) { ex.printStackTrace(); throw new IOException("解压失败:" + ex.toString()); } finally { if (zipFile != null) { try { zipFile.close(); } catch (IOException ex) { } } } }}
转载地址:https://cxyxy.blog.csdn.net/article/details/106244370 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
能坚持,总会有不一样的收获!
[***.219.124.196]2024年04月30日 05时34分17秒
关于作者
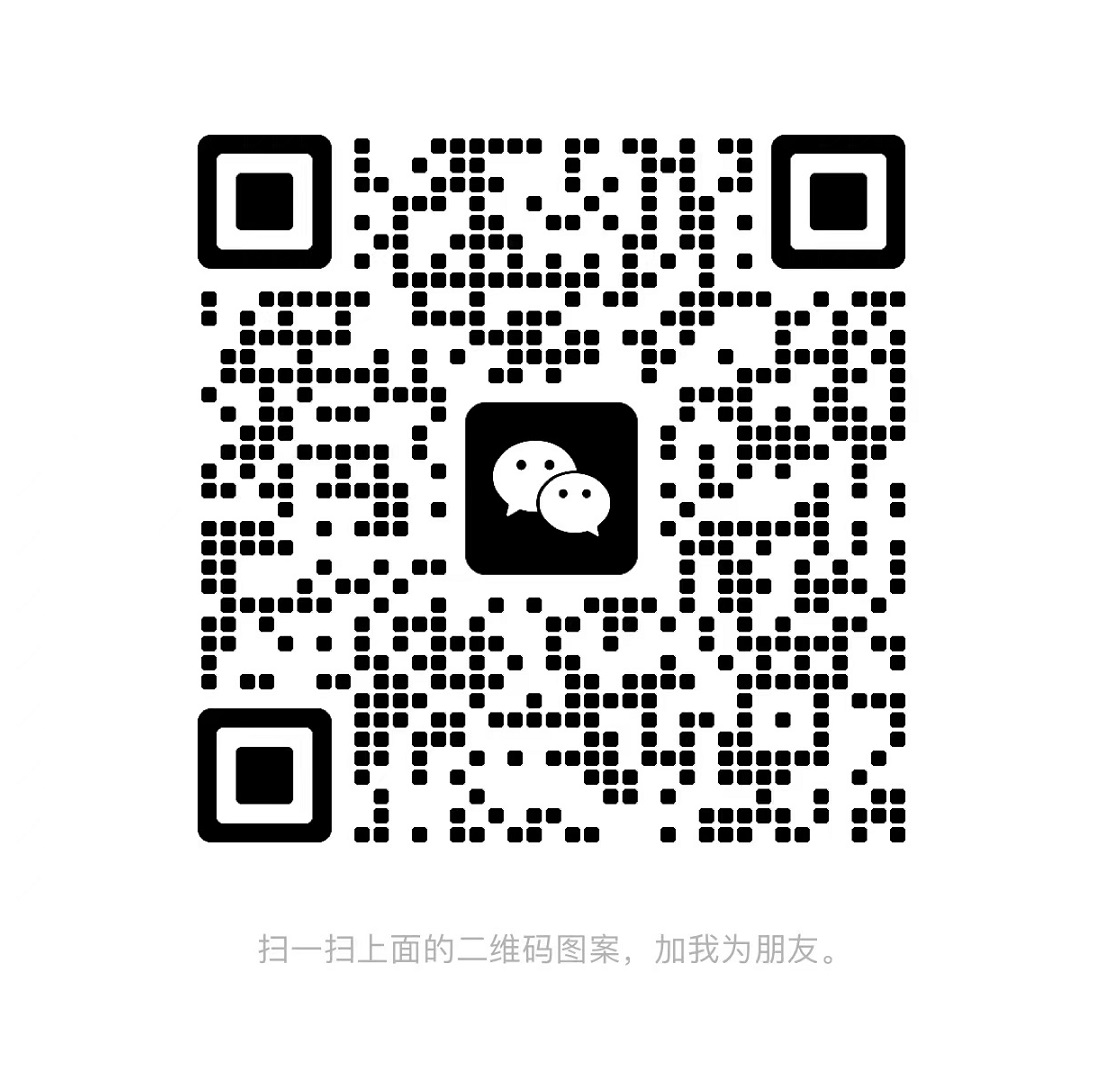
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
http-server的安装和使用(vue 打包后项目运行)
2019-04-30
vuex中使用typescript
2019-04-30
vue使用typescript / uniapp 使用typescript
2019-04-30
webpack打包html里面img后src为“[object Module]”
2019-04-30
使用webpack打包发现css单行注释报错
2019-04-30
webpack 打包拆分原则和理解(SplitChunksPlugin)
2019-04-30
Object.fromEntries方法报错
2019-04-30
MyEclipse10 加载慢内存消耗严重优化方案
2019-04-30
二叉排序树、二叉平衡树
2019-04-30
21、栈的压入、弹出序列
2019-04-30
如何利用平台的不同去推广?
2019-04-30
如何在 MacOS 中删除 Time Machine 本地快照?
2019-04-30
如何使用 SSD 升级 MacBook Pro
2019-04-30
Windows 11无法支持 Mac 安装?用这招轻松绕过 TPM 限制
2019-04-30
MacBook 键盘出现故障,如何修复?
2019-04-30
M1 Mac专用utm虚拟机安装Windows 11教程
2019-04-30
无需升级 macOS Monterey 使用新版本 safari 浏览器的方法
2019-04-30