
MyBatis研习录(13)——MyBatis二级缓存
发布日期:2021-06-30 11:11:10
浏览次数:2
分类:技术文章
本文共 11186 字,大约阅读时间需要 37 分钟。
版权声明
- 本文原创作者:谷哥的小弟
- 作者博客地址:http://blog.csdn.net/lfdfhl
使用二级缓存的前期准备
在MyBatis中使用二级缓存之前,需要进行如下配置:
1、在mybatis-config.xml中开启二级缓存
2、xxxMapper.xml中使用catch标签
3、pojo实现Serializable接口
public class YourPojo implements Serializable{ }
二级缓存的使用说明
当MyBatis中开启二级缓存,那么缓存的使用顺序如下:
- 1、当执行查询语句时候会先去二级缓存中查询数据,如果有则返回。如果二级缓存中没有则到一级缓存中查找。
- 2、如果一级缓存中有,则返回。如果一级缓存也没有则发送sql语句到数据库中去查询。
- 3、从数据库查询出数据后立马将数据保存到一级缓存中。
- 4、当SqlSession关闭的时候把一级缓存中的数据保存到二级缓存中。
请注意:如果执行增 、删、改 那么会同时清除一级缓存和二级缓存
二级缓存使用示例
数据准备
DROP DATABASE IF EXISTS mybatisDatabase;CREATE DATABASE mybatisDatabase;use mybatisDatabase;CREATE TABLE user( id INT PRIMARY KEY auto_increment, username VARCHAR(50), password VARCHAR(50), gender VARCHAR(10));INSERT INTO user(username,password,gender) VALUES("lucy","123456","female");INSERT INTO user(username,password,gender) VALUES("momo","234567","female");INSERT INTO user(username,password,gender) VALUES("xixi","345678","female");INSERT INTO user(username,password,gender) VALUES("pepe","456123","female");SELECT * FROM user;
搭建开发环境
创建普通的Java工程,结构如下:
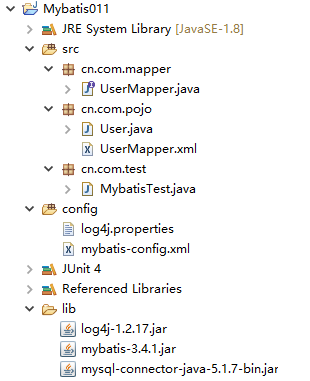
User
package cn.com.pojo;import java.io.Serializable;/** * 本文作者:谷哥的小弟 * 博客地址:http://blog.csdn.net/lfdfhl */public class User implements Serializable{ private static final long serialVersionUID = 112149170027586026L; private Integer id; private String username; private String password; private String gender; public User() { } public User(Integer id, String username, String password, String gender) { super(); this.id = id; this.username = username; this.password = password; this.gender = gender; } public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } @Override public String toString() { return "User [id=" + id + ", username=" + username + ", password=" + password + ", gender=" + gender + "]"; } }
UserMapper .java
package cn.com.mapper;import cn.com.pojo.User;/** * 本文作者:谷哥的小弟 * 博客地址:http://blog.csdn.net/lfdfhl */public interface UserMapper { public User queryUserById(Integer id); public int updateUser(User user);}
UserMapper .xml
update user set username=#{ username},password=#{ password},gender=#{ gender} where id=#{ id}
mybatis-config.xml
log4j.properties
# Global logging configurationlog4j.rootLogger=DEBUG, stdout# Console output...log4j.appender.stdout=org.apache.log4j.ConsoleAppenderlog4j.appender.stdout.layout=org.apache.log4j.PatternLayoutlog4j.appender.stdout.layout.ConversionPattern=%5p [%t] - %m%n
MybatisTest
package cn.com.test;import java.io.InputStream;import org.apache.ibatis.io.Resources;import org.apache.ibatis.session.SqlSession;import org.apache.ibatis.session.SqlSessionFactory;import org.apache.ibatis.session.SqlSessionFactoryBuilder;import org.junit.Test;import cn.com.mapper.UserMapper;import cn.com.pojo.User;/** * 本文作者:谷哥的小弟 * 博客地址:http://blog.csdn.net/lfdfhl */public class MybatisTest { static SqlSessionFactory sqlSessionFactory = null; public static SqlSessionFactory getSqlSessionFactory() { try { if (sqlSessionFactory == null) { InputStream inputStream = Resources.getResourceAsStream("mybatis-config.xml"); SqlSessionFactoryBuilder sqlSessionFactoryBuilder = new SqlSessionFactoryBuilder(); sqlSessionFactory = sqlSessionFactoryBuilder.build(inputStream); } return sqlSessionFactory; } catch (Exception e) { // TODO: handle exception } finally { } return null; } public void sqlSessionCatch() { //获取SqlSession SqlSession sqlSession=getSqlSessionFactory().openSession(); //利用SqlSession得到UserMapper UserMapper userMapper = sqlSession.getMapper(UserMapper.class); //利用SqlSession执行数据查询 User user = userMapper.queryUserById(1); System.out.println(user.getUsername()); //关闭SqlSession sqlSession.close(); } @Test public void testSqlSessionCatch() { sqlSessionCatch(); sqlSessionCatch(); sqlSessionCatch(); sqlSessionCatch(); } public void sqlSessionCatchClean() { //获取SqlSession SqlSession sqlSession=getSqlSessionFactory().openSession(); //利用SqlSession得到UserMapper UserMapper userMapper = sqlSession.getMapper(UserMapper.class); //利用SqlSession执行数据查询 User user = userMapper.queryUserById(1); System.out.println(user.getUsername()); //利用SqlSession执行数据更新 userMapper.updateUser(new User(2, "bmbm", "123456", "female")); //关闭SqlSession sqlSession.close(); } @Test public void testSqlSessionCatchClean() { sqlSessionCatchClean(); sqlSessionCatchClean(); sqlSessionCatchClean(); sqlSessionCatchClean(); }}
testSqlSessionCatch测试结果如下:
DEBUG [main] - Logging initialized using 'class org.apache.ibatis.logging.log4j.Log4jImpl' adapter.DEBUG [main] - PooledDataSource forcefully closed/removed all connections.DEBUG [main] - PooledDataSource forcefully closed/removed all connections.DEBUG [main] - PooledDataSource forcefully closed/removed all connections.DEBUG [main] - PooledDataSource forcefully closed/removed all connections.DEBUG [main] - Cache Hit Ratio [cn.com.mapper.UserMapper]: 0.0DEBUG [main] - Opening JDBC ConnectionDEBUG [main] - Created connection 1392425346.DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - ==> Preparing: select id,username,password,gender from user where id = ? DEBUG [main] - ==> Parameters: 1(Integer)DEBUG [main] - <== Total: 1lucyDEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Returned connection 1392425346 to pool.DEBUG [main] - Cache Hit Ratio [cn.com.mapper.UserMapper]: 0.5lucyDEBUG [main] - Cache Hit Ratio [cn.com.mapper.UserMapper]: 0.6666666666666666lucyDEBUG [main] - Cache Hit Ratio [cn.com.mapper.UserMapper]: 0.75lucy
请注意命中率Cache Hit Ratio的变化:
- 1、第一次查询时无缓存,所以Cache Hit Ratio=0
- 2、第二次查询时有缓存,共两次查询其中一次来自于缓存;所以,Cache Hit Ratio=0.5
- 3、第三次查询时有缓存,共三次查询其中二次来自于缓存;所以,Cache Hit Ratio=0.66666666666666666666
- 4、第四次查询时有缓存,共四次查询其中三次来自于缓存;所以,Cache Hit Ratio=0.75
testSqlSessionCatchClean测试结果如下:
DEBUG [main] - Logging initialized using 'class org.apache.ibatis.logging.log4j.Log4jImpl' adapter.DEBUG [main] - PooledDataSource forcefully closed/removed all connections.DEBUG [main] - PooledDataSource forcefully closed/removed all connections.DEBUG [main] - PooledDataSource forcefully closed/removed all connections.DEBUG [main] - PooledDataSource forcefully closed/removed all connections.DEBUG [main] - Cache Hit Ratio [cn.com.mapper.UserMapper]: 0.0DEBUG [main] - Opening JDBC ConnectionDEBUG [main] - Created connection 1392425346.DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - ==> Preparing: select id,username,password,gender from user where id = ? DEBUG [main] - ==> Parameters: 1(Integer)DEBUG [main] - <== Total: 1lucyDEBUG [main] - ==> Preparing: update user set username=?,password=?,gender=? where id=? DEBUG [main] - ==> Parameters: bmbm(String), 123456(String), female(String), 2(Integer)DEBUG [main] - <== Updates: 1DEBUG [main] - Rolling back JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Returned connection 1392425346 to pool.DEBUG [main] - Cache Hit Ratio [cn.com.mapper.UserMapper]: 0.0DEBUG [main] - Opening JDBC ConnectionDEBUG [main] - Checked out connection 1392425346 from pool.DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - ==> Preparing: select id,username,password,gender from user where id = ? DEBUG [main] - ==> Parameters: 1(Integer)DEBUG [main] - <== Total: 1lucyDEBUG [main] - ==> Preparing: update user set username=?,password=?,gender=? where id=? DEBUG [main] - ==> Parameters: bmbm(String), 123456(String), female(String), 2(Integer)DEBUG [main] - <== Updates: 1DEBUG [main] - Rolling back JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Returned connection 1392425346 to pool.DEBUG [main] - Cache Hit Ratio [cn.com.mapper.UserMapper]: 0.0DEBUG [main] - Opening JDBC ConnectionDEBUG [main] - Checked out connection 1392425346 from pool.DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - ==> Preparing: select id,username,password,gender from user where id = ? DEBUG [main] - ==> Parameters: 1(Integer)DEBUG [main] - <== Total: 1lucyDEBUG [main] - ==> Preparing: update user set username=?,password=?,gender=? where id=? DEBUG [main] - ==> Parameters: bmbm(String), 123456(String), female(String), 2(Integer)DEBUG [main] - <== Updates: 1DEBUG [main] - Rolling back JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Returned connection 1392425346 to pool.DEBUG [main] - Cache Hit Ratio [cn.com.mapper.UserMapper]: 0.0DEBUG [main] - Opening JDBC ConnectionDEBUG [main] - Checked out connection 1392425346 from pool.DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - ==> Preparing: select id,username,password,gender from user where id = ? DEBUG [main] - ==> Parameters: 1(Integer)DEBUG [main] - <== Total: 1lucyDEBUG [main] - ==> Preparing: update user set username=?,password=?,gender=? where id=? DEBUG [main] - ==> Parameters: bmbm(String), 123456(String), female(String), 2(Integer)DEBUG [main] - <== Updates: 1DEBUG [main] - Rolling back JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@52feb982]DEBUG [main] - Returned connection 1392425346 to pool.
在此测试中命中率Cache Hit Ratio=0;再次验证了:如果执行增 、删、改 那么会同时清除一级缓存和二级缓存
二级缓存策略
MyBatis二级缓存常用策略如下:
• LRU – 最近最少使用的,即移除最长时间不被使用的对象(默认策略)。
• FIFO – 先进先出,即按对象进入缓存的顺序来移除它们。 • SOFT – 软引用,即移除基于垃圾回收器状态和软引用规则的对象。 • WEAK – 弱引用,即更积极地移除基于垃圾收集器状态和弱引用规则的对象。转载地址:https://it9527.blog.csdn.net/article/details/103284317 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
逛到本站,mark一下
[***.202.152.39]2024年04月14日 22时58分05秒
关于作者
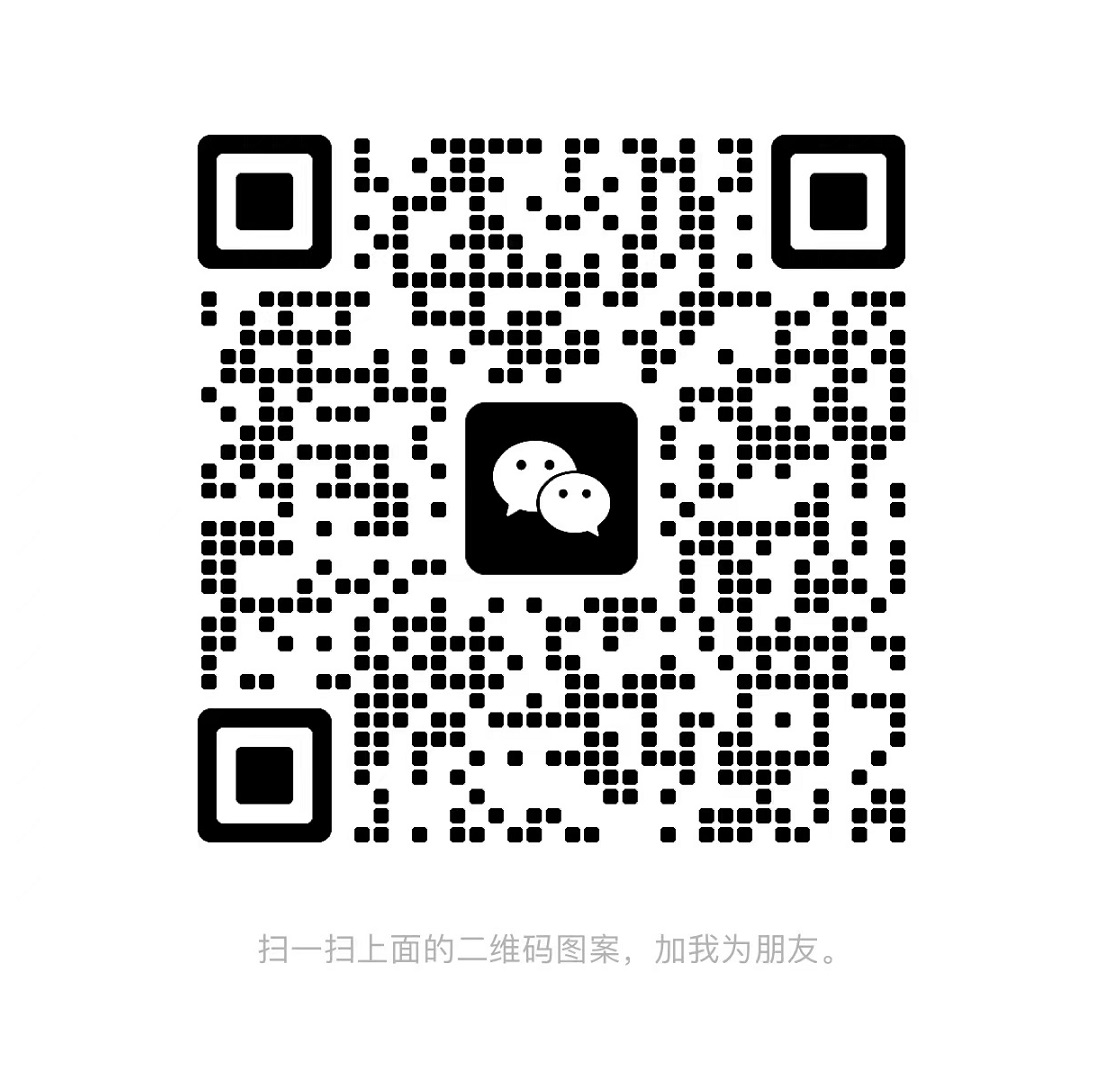
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
169.实现秒级作业的案例
2019-04-30
170.解决计算机名修改或作业移植导致的服务名称问题
2019-04-30
171.操作SQL SERVERAGENT服务的扩展存储过程
2019-04-30
rtf格式的一些说明,转载的,我找到的rtf资料中比较实用的一片文章了
2019-04-30
RTF文件格式编码说明
2019-04-30
RTF 语法1
2019-04-30
RTF文件格式研究报告
2019-04-30
RichEdit的用法总结
2019-04-30
BCB 中测量Richedit 的文本总行高
2019-04-30
200.自定义数据类型修改
2019-04-30
201.创建与删除用户定义数据类型-案例
2019-04-30
202.为用户定义的数据类型绑定规则案例
2019-04-30
203.为用户定义的数据类型绑定默认值案例
2019-04-30
204.修改已经被表引用的数据定义数据类型-案例
2019-04-30
205.修改用户定义数据类型对已经编译的存储过程的影响的案例
2019-04-30
控件命名
2019-04-30
C++builder常用函数
2019-04-30
C++Builder文件操作大全
2019-04-30
用C++ Builder XE 10编译生成EXE运行问题
2019-04-30
212.全文检索
2019-04-30