
并发编程 线程池以及@Async注解的使用
1:启动类添加
发布日期:2021-06-30 12:37:00
浏览次数:3
分类:技术文章
本文共 6788 字,大约阅读时间需要 22 分钟。
建议先看我另外一篇文章:
开发环境
- SpringBoot 2.1.10.RELEASE
- JDK 1.8
1:启动类添加@EnableAsync
注解
package com.nobody;import org.springframework.boot.SpringApplication;import org.springframework.boot.autoconfigure.SpringBootApplication;import org.springframework.scheduling.annotation.EnableAsync;@SpringBootApplication@EnableAsyncpublic class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); }}
2:线程池配置
package com.nobody.config;import java.util.concurrent.Executor;import java.util.concurrent.ThreadPoolExecutor;import org.springframework.context.annotation.Bean;import org.springframework.context.annotation.Configuration;import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor;@Configurationpublic class ExecutorConfig { public static final int CORE_POOL_SIZE = 5; public static final int MAX_POOL_SIZE = 10; public static final int QUEUE_CAPACITY = 100; @Bean("myExecutor") public Executor asyncServiceExecutor() { ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor(); // 核心线程数大小 executor.setCorePoolSize(CORE_POOL_SIZE); // 最大线程数大小 executor.setMaxPoolSize(MAX_POOL_SIZE); // 阻塞队列容量 executor.setQueueCapacity(QUEUE_CAPACITY); // 线程名前缀 executor.setThreadNamePrefix("myTask-"); // rejectionPolicy:当queue达到maxSize并且此时maxPoolSize也达到最大值的时候,对于新任务的处理策略 // CallerRunsPolicy:不在新线程中执行任务,而是交由调用者所在的线程来执行 executor.setRejectedExecutionHandler(new ThreadPoolExecutor.CallerRunsPolicy()); executor.initialize(); return executor; }}
3:编写异步方法
package com.nobody.domain;import java.util.Map;import java.util.concurrent.CountDownLatch;import java.util.concurrent.Future;import java.util.concurrent.TimeUnit;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import org.slf4j.MDC;import org.springframework.scheduling.annotation.Async;import org.springframework.scheduling.annotation.AsyncResult;import org.springframework.stereotype.Service;@Servicepublic class AsyncService { private static final Logger LOGGER = LoggerFactory.getLogger(AsyncService.class); // 简单版本 @Async public void asyncTaskA() { LOGGER.info("asyncTaskA 异步方法执行."); } // 入参类型,此处将调用者(线程)的MDC信息传入,主要是保持日志输出时,两个线程有同样的traceId @Async public void asyncTaslB(MapcontextMap) { MDC.setContextMap(contextMap); LOGGER.info("asyncTaskB 异步方法执行."); } // 线程执行后计数器减1 @Async public void asyncTaskC(CountDownLatch cdl) { LOGGER.info("asyncTaskC 异步方法执行."); try { TimeUnit.SECONDS.sleep(2); } catch (InterruptedException e) { e.printStackTrace(); } cdl.countDown(); } // 有返回参数类型 @Async public Future asyncTaskD(String message) { LOGGER.info("asyncTaskD 异步方法执行."); return new AsyncResult ("@" + message); }}
4:异步调用
package com.nobody.controller;import java.util.concurrent.ExecutionException;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;import com.nobody.domain.AsyncService;@RestController@RequestMapping("demo")public class DemoController { private static final Logger LOGGER = LoggerFactory.getLogger(DemoController.class); @Autowired private AsyncService asyncService; @GetMapping("test") public void test() throws InterruptedException, ExecutionException { asyncService.asyncTaskA(); LOGGER.info("主线程执行..."); }}
输出结果:

package com.nobody.controller;import java.util.UUID;import java.util.concurrent.ExecutionException;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import org.slf4j.MDC;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;import com.nobody.domain.AsyncService;@RestController@RequestMapping("demo")public class DemoController { private static final Logger LOGGER = LoggerFactory.getLogger(DemoController.class); @Autowired private AsyncService asyncService; @GetMapping("test") public void test() throws InterruptedException, ExecutionException { MDC.put("traceId", UUID.randomUUID().toString().replaceFirst("-", "")); asyncService.asyncTaskB(MDC.getCopyOfContextMap()); LOGGER.info("主线程执行..."); }}
输出结果:

package com.nobody.controller;import java.util.concurrent.CountDownLatch;import java.util.concurrent.ExecutionException;import java.util.concurrent.TimeUnit;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;import com.nobody.domain.AsyncService;@RestController@RequestMapping("demo")public class DemoController { private static final Logger LOGGER = LoggerFactory.getLogger(DemoController.class); @Autowired private AsyncService asyncService; @GetMapping("test") public void test() throws InterruptedException, ExecutionException { CountDownLatch cdl = new CountDownLatch(2); asyncService.asyncTaskC(cdl); asyncService.asyncTaskC(cdl); try { // 等等两个线程执行后,再继续下面的执行,如果超过5s则不等待 cdl.await(5, TimeUnit.SECONDS); } catch (InterruptedException e) { e.printStackTrace(); } LOGGER.info("主线程执行..."); }}
输出结果:

package com.nobody.controller;import java.util.concurrent.ExecutionException;import java.util.concurrent.Future;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RestController;import com.nobody.domain.AsyncService;@RestController@RequestMapping("demo")public class DemoController { private static final Logger LOGGER = LoggerFactory.getLogger(DemoController.class); @Autowired private AsyncService asyncService; @GetMapping("test") public void test() throws InterruptedException, ExecutionException { Futurefuture = asyncService.asyncTaskD("Mr.nobody"); LOGGER.info("future result:" + future.get()); LOGGER.info("主线程执行..."); }}
转载地址:https://javalib.blog.csdn.net/article/details/106280373 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
初次前来,多多关照!
[***.217.46.12]2024年04月14日 06时20分38秒
关于作者
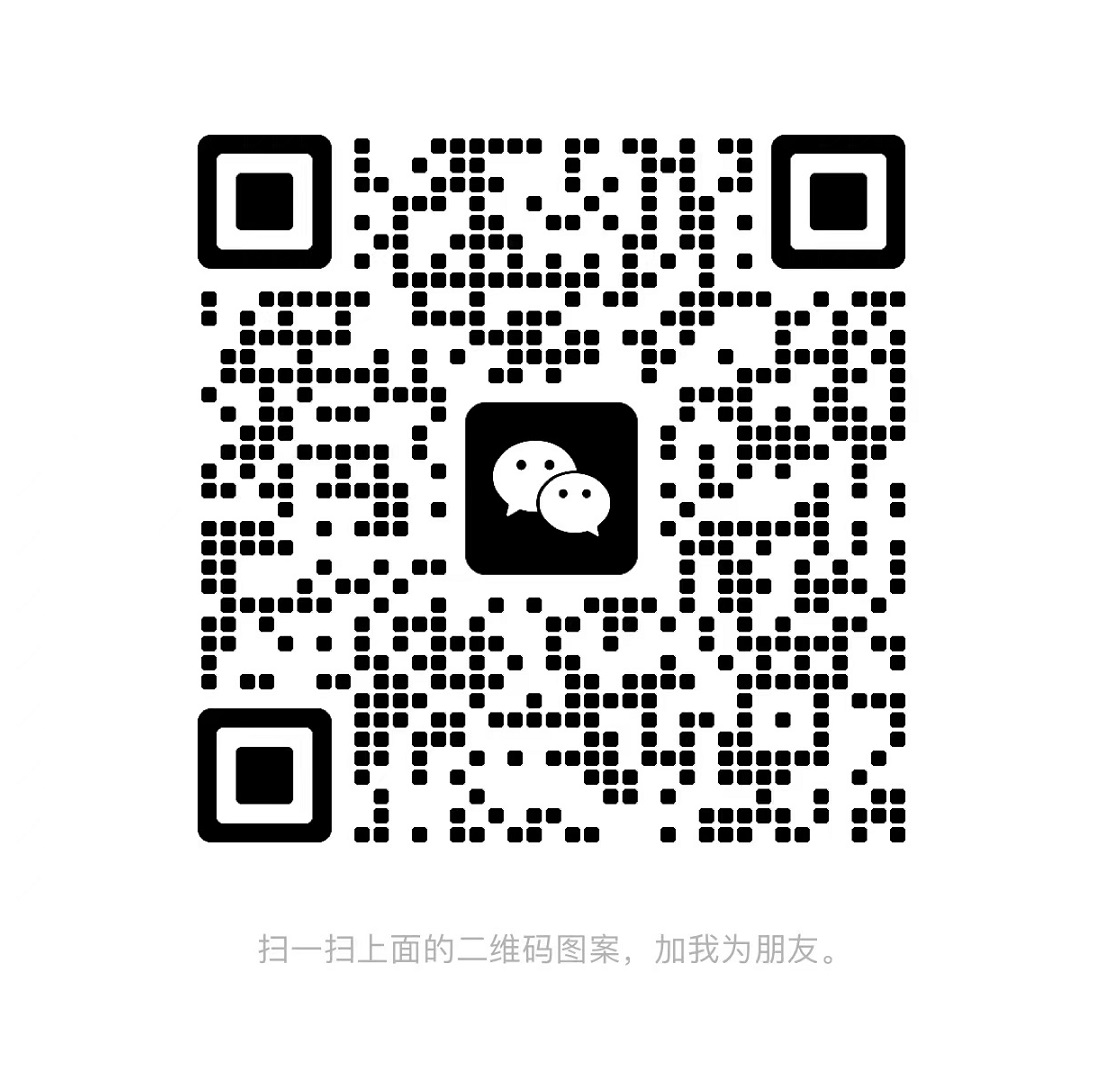
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
scala list
2019-05-01
svn服务器安装
2019-05-01
spark 笔记1
2019-05-01
svn 没有作者信息) | (没有时间信息
2019-05-01
shell dirname basename
2019-05-01
未来已至,5G加持下的云游戏将走向何方?
2019-05-01
计算机网络 —— 网络层 1.
2019-05-01
Android生命周期
2019-05-01
Android进度条自定义——类似ProgressDialog效果的Dialog
2019-05-01
Android 之 ContentProvider 与 ContentResolver
2019-05-01
【接口自动化】
2019-05-01
SpringBoot搭建一个Web工程
2019-05-01
Spring Boot 安全框架 Shiro 入门
2019-05-01
如何用一句话激怒互联网人?
2019-05-01
用 Python 爬了点你们喜欢的电影
2019-05-01
推荐一位川大零基础转行 Python 的人生勇士
2019-05-01
讲真,做Python一定不要只会一个方向!
2019-05-01
Python 2大限来了!113天后自生自灭,官方不再维护更新
2019-05-01
GitHub 热榜第一的 Python 抢票神器!节假日能用上
2019-05-01
1.6w 星开源项目,但作者月薪却不到 5K
2019-05-01