
C#练习题
发布日期:2021-06-30 19:56:39
浏览次数:2
分类:技术文章
本文共 14528 字,大约阅读时间需要 48 分钟。
一:求1000以内所有的完数,并输出所有因子(完数:一个数恰好等于它的所有因子之和)
因子和因数:因子不包括数本身,因数包括数本身
using System;class MainClass{ public static void Main(string[] args) { for (int i = 1; i <= 1000; i++) { int sum = 0; string str = ""; for (int j = 1; j < i; j++) { if (i % j == 0) { sum += j; str += j + "+"; } } if (sum == i) { str = str.TrimEnd('+'); Console.WriteLine($"{i}是完数,因子是{str}"); } } }}
二:一只猴子吃桃子,第一天吃掉桃子总数的一半多一个,第二天又吃掉剩下桃子数量的一半多一个,以后每天吃掉前一天剩下桃子数量的一半多一个,到第n天的时候,只剩下一个桃子,请问他第一天吃的时候一共有多少个桃子。用户输入n的值,计算出第一天吃的时候桃子总数是多少
using System;namespace Application{ class MainClass { public static void Main(string[] args) { int count = 1; int num = int.Parse(Console.ReadLine()); for (int i = num - 1; i >= 0; i--) { count = (count + 1) * 2; } Console.WriteLine("总数是:" + count); } }}
三:一组已经从小到大排序好的整数数组,用户输入一个数字,插入到数组中,使新的数组仍然有序
——解法1
using System;class MainClass{ static void Main(string[] args) { int[] rawArray = { 1, 2, 4, 7, 10 }; int[] newArray = new int[rawArray.Length + 1]; int myNum = int.Parse(Console.ReadLine()); int insertIndex = 0; for (int i = 0; i < rawArray.Length; i++) { if (myNum > rawArray[i]) { insertIndex = i + 1; } } bool isInsert = false; for (int i = 0; i < newArray.Length; i++) { if (isInsert == false) { if (i == insertIndex) { newArray[i] = myNum; isInsert = true; } else { newArray[i] = rawArray[i]; } } else { newArray[i] = rawArray[i - 1]; } } foreach (int item in newArray) { Console.Write(item + " "); } }}
——解法2
using System;class MainClass{ static void Main(string[] args) { int[] rawArray = { 1, 2, 4, 7, 10 }; int[] newArray = new int[rawArray.Length + 1]; int myNum = int.Parse(Console.ReadLine()); int rawIndex = 0; bool isInsert = false; for (int i = 0; i < newArray.Length; i++) { if (i >= rawArray.Length && isInsert == false) { newArray[i] = myNum; isInsert = true; break; } if (myNum < rawArray[rawIndex] && isInsert == false) { newArray[i] = myNum; isInsert = true; } else { newArray[i] = rawArray[rawIndex++]; } } foreach (int item in newArray) { Console.Write(item + " "); } }}
四:实现斐波那契数列(1,1,2,3,5,8...)
——函数的递归实现
using System;class Program{ static void Main(string[] args) { Console.WriteLine(F(6)); } static int F(int n) { if (n <= 0) { return 0; } else if (n <= 2) { return 1; } return F(n - 1) + F(n - 2); }}
——普通函数的实现
using System;class Program{ static void Main(string[] args) { int res = Fun(4); Console.WriteLine(res); } static int Fun(int n) { int a = 1; int b = 1; for (int i = 3; i <= n; i++) { b += a; a = b - a; } return b; }}
五:一个小球从100m的高度自由落下,每次落地后反弹回原高度的一半,求它在第十次落地时,共经过多少米?第十次反弹多高?
using System;class MainClass{ static void Main(string[] args) { float dis = 0; float height = 100; for (int i = 0; i < 10; i++) { if (i == 0) { dis += height; } else { dis += height * 2; } height /= 2; } Console.WriteLine($"第十次落地时共经过{dis}米,反弹高度为{height / 2}米"); }}
六:求1!+2!+3!+4!+5!
——定义函数去完成每个数字的阶乘
using System;class Program{ static void Main(string[] args) { int sum = 0; for (int i = 1; i <= 5; i++) { sum += Fun(i); } Console.WriteLine(sum); } static int Fun(int n) { if (n == 1) return 1; return n * Fun(n - 1); }}
——在Main函数中完成所有操作
using System;class Program{ static void Main(string[] args) { long sum = 1; for (int i = 1; i <= 20; i++) { long num = 1; for (int j = i; j >= 1; j--) { num *= j; } sum += num; } Console.WriteLine(sum); }}
七:对一个float类型的小数进行四舍五入
——未优化版
using System;class MainClass{ static void Main(string[] args) { float num = float.Parse(Console.ReadLine()); int numInteger = (int)num; float numFloat = num - numInteger; int res = 0; if (numFloat >= 0.5f) { res = ++numInteger; } else { res = numInteger; } Console.WriteLine(res); }}
——优化版
using System;class Program{ static void Main(string[] args) { float num = float.Parse(Console.ReadLine()); float res = (int)(num + 0.5f); Console.WriteLine(res); }}
八:3个可乐瓶可以换一瓶可乐,现在有364瓶可乐。问一共可以喝多少瓶可乐?剩下几个空瓶?
——解法1
using System;class MainClass{ static void Main(string[] args) { int drinkCount = 0; int totalCount = 364; for (int i = 0; i < totalCount; i++) { drinkCount++; if (drinkCount % 3 == 0) { totalCount++; } } int remain = totalCount % 3; Console.WriteLine($"一共可以喝{totalCount}瓶可乐,剩下{remain}个空瓶"); }}
——解法2
using System;class MainClass{ public static void Main(string[] args) { int totalCount = 364; int emptyCount = totalCount; while (emptyCount >= 3) { totalCount += emptyCount / 3; emptyCount = emptyCount / 3 + emptyCount % 3; } Console.WriteLine($"一共可以喝{totalCount}瓶可乐,剩下{emptyCount}个空瓶"); }}
九:输入一个字符串,字母加密(a-d A-D x-a X-A),其他字符原样输出
using System;class MainClass{ static void Main(string[] args) { char[] charArray = Console.ReadLine().ToCharArray(); string str = ""; for (int i = 0; i < charArray.Length; i++) { if (charArray[i] >= 'a' && charArray[i] <= 'z') { if (charArray[i] >= 'x') { char temp = (char)(charArray[i] - 'x' + 'a'); str += temp; } else { str += (char)(charArray[i] + 3); } continue; } if (charArray[i] >= 'A' && charArray[i] <= 'Z') { if (charArray[i] >= 'X') { char temp = (char)(charArray[i] - 'X' + 'A'); str += temp; } else { str += (char)(charArray[i] + 3); } continue; } } Console.WriteLine("加密后的密码为:" + str); }}
十:控制台输出九九乘法表
using System;class MainClass{ public static void Main(string[] args) { for (int i = 1; i <= 9; i++) { for (int j = 1; j <= i; j++) { Console.Write($"{j}*{i}={i * j} "); } Console.WriteLine(); } }}
十一:输出1000以内的素数
素数(质数):大于1的自然数,除了1和本身外,不再有其他的因数
using System;class MainClass{ public static void Main(string[] args) { for (int i = 2; i <= 1000; i++) { bool right = true; //这里的i/2进行了算法优化 for (int j = 2; j < i / 2; j++) { if (i % j == 0) { right = false; break; } } if (right) { Console.WriteLine(i + "是素数"); } } }}
十二:控制台随机一个1-100的数字,让用户猜数字,如果输入的数字比随机数大或者小就提示用户并且继续输入,直到输入正确的数字后退出程序
using System;class MainClass{ static void Main(string[] args) { Random r = new Random(); int n = r.Next(1, 101); Console.WriteLine("please input a num"); while (true) { int num = int.Parse(Console.ReadLine()); if (num > n) { Console.WriteLine("输入的数字大了"); } else if (num < n) { Console.WriteLine("输入的数字小了"); } else { Console.WriteLine("猜对了!"); break; } } }}
十三:输出100-9999之间的水仙花数(水仙花数:153=1*1*1+5*5*5+3*3*3)
using System;class Program{ static void Main(string[] args) { for (int i = 100; i <= 9999; i++) { double sum = 0; char[] charArray = i.ToString().ToCharArray(); for (int j = 0; j < charArray.Length; j++) { charArray[j] -= '0'; sum += Math.Pow(charArray[j], 3); } if (sum == i) { Console.WriteLine(i + "是水仙花数"); } } }}
十四:输入两个数,求最大公约数和最小公倍数
using System;class Program{ static void Main(string[] args) { Console.WriteLine("请输入两个数"); int n1 = int.Parse(Console.ReadLine()); int n2 = int.Parse(Console.ReadLine()); int res1 = 0; for (int i = 1; i <= n1 && i <= n2; i++) { if (n1 % i == 0 && n2 % i == 0) { res1 = i; } } int res2 = 0; if (n1 > n2) { res2 = n1; } else { res2 = n2; } while (true) { if (res2 % n1 == 0 && res2 % n2 == 0) { break; } res2++; } Console.WriteLine($"最大公约数是{res1},最小公倍数是{res2}"); }}
十五:初始化一个10个元素的数组,赋值为0-9,乱序后,输出
using System;class Program{ static void Main(string[] args) { int[] num = new int[10]; for (int i = 0; i < num.Length; i++) { num[i] = i; } Console.WriteLine("正序数组————"); foreach (int item in num) { Console.Write(item + " "); } Console.WriteLine(); Random r = new Random(); for (int i = 0; i < num.Length; i++) { int newIndex = r.Next(0, 10); int temp = num[i]; num[i] = num[newIndex]; num[newIndex] = temp; } Console.WriteLine("乱序数组————"); foreach (int item in num) { Console.Write(item + " "); } }}
十六:先用随机数初始化一个数组(20个元素),之后将奇数放左边,偶数放右边
using System;using System.Collections.Generic;class Program{ static void Main(string[] args) { int[] num = new int[20]; Random r = new Random(); for (int i = 0; i < num.Length; i++) { int n = r.Next(1, 21); num[i] = n; } Console.WriteLine("排序前————"); foreach (int item in num) { Console.Write(item + " "); } List oddList = new List (); List evenList = new List (); for (int i = 0; i < num.Length; i++) { if (num[i] % 2 == 0) { evenList.Add(num[i]); } else { oddList.Add(num[i]); } } int[] newNum = new int[20]; int oddIndex = 0; int evenIndex = 0; for (int i = 0; i < newNum.Length; i++) { if (oddIndex < oddList.Count) { newNum[i] = oddList[oddIndex++]; } else { newNum[i] = evenList[evenIndex++]; } } Console.WriteLine("排序后————"); foreach (int item in newNum) { Console.Write(item + " "); } }}
十七:输出以下数组 1 1 1 1 1 1 1 1 1 1 2 2 2 2 2 2 2 1 1 2 3 3 3 3 3 2 1 1 2 3 4 4 4 3 2 1 1 2 3 4 5 4 3 2 1 1 2 3 4 4 4 3 2 1 1 2 3 3 3 3 3 2 1 1 2 2 2 2 2 2 2 1 1 1 1 1 1 1 1 1 1
class Program{ static void Main(string[] args) { for (int i = 1; i <= 9; i++) { for (int j = 1; j <= 9; j++) { if (i == 1 || j == 1 || i == 9 || j == 9) { Console.Write("1 "); } else if (i == 2 || j == 2 || i == 8 || j == 8) { Console.Write("2 "); } else if (i == 3 || j == 3 || i == 7 || j == 7) { Console.Write("3 "); } else if (i == 4 || j == 4 || i == 6 || j == 6) { Console.Write("4 "); } else if (i == 5 || j == 5) { Console.Write("5 "); } } Console.WriteLine(); } }}
十八:输出杨辉三角 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
using System;class Program{ static void Main(string[] args) { Console.Write("输入杨辉三角长度:"); int length = Convert.ToInt32(Console.ReadLine());//指定杨辉三角形的长度 int[][] a = new int[length][]; for (int i = 0; i < a.Length; i++) a[i] = new int[i + 1]; for (int j = 0; j < a.Length; j++) { a[j][0] = 1; a[j][j] = 1; for (int m = 1; m < a[j].Length - 1; m++) a[j][m] = a[j - 1][m - 1] + a[j - 1][m]; } for (int i = 0; i < a.Length; i++) { for (int k = 0; k < length - i; k++) Console.Write(" "); for (int j = 0; j < a[i].Length; j++) Console.Write("{0} ", a[i][j]); Console.Write("\n"); } }}
十九:输入一个十进制数,转换为二进制数输出
using System;class Program{ static void Main(string[] args) { Console.WriteLine("请输入一个数字"); int num = int.Parse(Console.ReadLine()); int rawNum = num; string str = ""; while (num != 0) { str += num % 2; num /= 2; } string res = ""; for (int i = str.Length - 1; i >= 0; i--) { res += str[i]; } Console.WriteLine($"{rawNum}的二进制数为:{res}"); }}
二十:
转载地址:https://liuhaowen.blog.csdn.net/article/details/100104537 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
很好
[***.229.124.182]2024年05月03日 05时23分36秒
关于作者
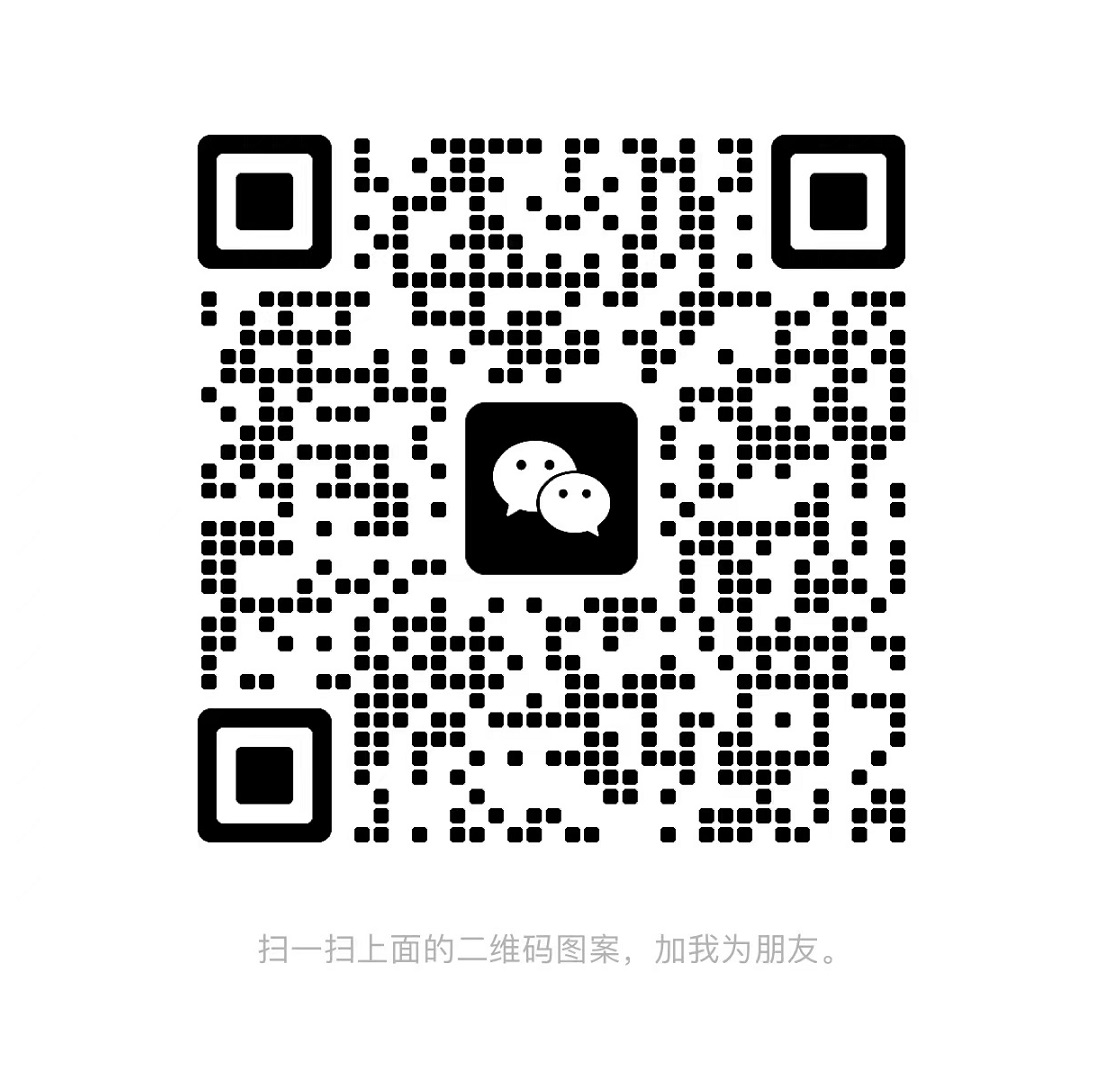
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
Django + REST学习笔记
2019-04-30
【转载】将Ubuntu16.04 中gedit在仅显示一个文件时显示文件名tab
2019-04-30
fstream 对象多次使用时注意clear
2019-04-30
调试 LenaCV 3D Camera (Linux)
2019-04-30
OpenCV杂记 - Mat in C++
2019-04-30
lnmp部署
2019-04-30
location区段
2019-04-30
nginx访问控制、基于用户认证、https配置
2019-04-30
用zabbix监控nginx
2019-04-30
SaltStack
2019-04-30
Jenkins 控制台输出中的奇怪字符
2019-04-30
Linux添加系统调用
2019-04-30
linux内存的寻址方式
2019-04-30
ubunut16.04的pip3出现问题,重新安装pip3
2019-04-30
how2heap-double free
2019-04-30
how2heap-fastbin_dup_consolidate
2019-04-30
orw_shellcode_模板
2019-04-30
[fmt+shellcode]string
2019-04-30