
【Laravel3.0.0源码阅读分析】视图类view.php
发布日期:2021-06-30 20:44:43
浏览次数:2
分类:技术文章
本文共 8889 字,大约阅读时间需要 29 分钟。
* // Create a new view instance * $view = new View('home.index'); * * // Create a new view instance of a bundle's view * $view = new View('admin::home.index'); * * // Create a new view instance with bound data * $view = new View('home.index', array('name' => 'Taylor')); * * * @param string $view * @param array $data * @return void */ public function __construct($view, $data = array()) { $this->view = $view; $this->data = $data; $this->path = $this->path($view); // If a session driver has been specified, we will bind an instance of the // validation error message container to every view. If an errors instance // exists in the session, we will use that instance. // 如果指定了会话驱动程序,我们会将验证错误消息容器的实例绑定到每个视图。 // 如果会话中存在错误实例,我们将使用该实例。 // This makes error display in the view extremely convenient, since the // developer can always assume they have a message container instance // available to them in the view's variables. // 这使视图中的错误显示极为方便,因为开发人员始终可以认为视图变量中有可用的消息容器实例。 if ( ! isset($this->data['errors'])) { if (Session::started() and Session::has('errors')) { $this->data['errors'] = Session::get('errors'); } else { $this->data['errors'] = new Messages; } } } /** * Get the path to a given view on disk. * 获取磁盘上给定视图的路径。 * @param string $view * @return string */ protected function path($view) { $view = str_replace('.', '/', $view); $root = Bundle::path(Bundle::name($view)).'views/'; // Views may have the normal PHP extension or the Blade PHP extension, so // we need to check if either of them exist in the base views directory // for the bundle and return the first one we find. // 视图可能有普通的 PHP 扩展或 Blade PHP 扩展,所以我们需要检查它们是否存在于包的基本视图目录中,并返回我们找到的第一个。 foreach (array(EXT, BLADE_EXT) as $extension) { if (file_exists($path = $root.Bundle::element($view).$extension)) { return $path; } } throw new \Exception("View [$view] does not exist."); } /** * Create a new view instance. * 创建一个新的视图实例。 ** // Create a new view instance * $view = View::make('home.index'); * * // Create a new view instance of a bundle's view * $view = View::make('admin::home.index'); * * // Create a new view instance with bound data * $view = View::make('home.index', array('name' => 'Taylor')); *
* * @param string $view * @param array $data * @return View */ public static function make($view, $data = array()) { return new static($view, $data); } /** * Create a new view instance of a named view. * 创建命名视图的新视图实例。 ** // Create a new named view instance * $view = View::of('profile'); * * // Create a new named view instance with bound data * $view = View::of('profile', array('name' => 'Taylor')); *
* * @param string $name * @param array $data * @return View */ public static function of($name, $data = array()) { return new static(static::$names[$name], $data); } /** * Assign a name to a view. * 为视图指定名称。 ** // Assign a name to a view * View::name('partials.profile', 'profile'); * * // Resolve an instance of a named view * $view = View::of('profile'); *
* * @param string $view * @param string $name * @return void */ public static function name($view, $name) { static::$names[$name] = $view; } /** * Register a view composer with the Event class. * 向Event类注册一个视图编辑器。 ** // Register a composer for the "home.index" view * View::composer('home.index', function($view) * { * $view['title'] = 'Home'; * }); *
* * @param string $view * @param Closure $composer * @return void */ public static function composer($view, $composer) { Event::listen("laravel.composing: {$view}", $composer); } /** * Get the evaluated string content of the view. * 获取视图的评估字符串内容。 * @return string */ public function render() { // To allow bundles or other pieces of the application to modify the // view before it is rendered, we will fire an event, passing in the // view instance so it can modified. // 为了允许包或应用程序的其他部分在呈现之前修改视图,我们将触发一个事件,传入视图实例以便它可以修改。 Event::fire("laravel.composing: {$this->view}", array($this)); $data = $this->data(); ob_start() and extract($data, EXTR_SKIP); // If the view is Bladed, we need to check the view for changes and // get the path to the compiled view file. Otherwise, we'll just // use the regular path to the view. // 如果视图是 Bladed,我们需要检查视图的变化并获取编译视图文件的路径。 否则,我们将只使用视图的常规路径。 // Also, if the Blade view has expired or doesn't exist it will be // re-compiled and placed in the view storage directory. The Blade // views are re-compiled the original view changes. // 此外,如果 Blade 视图已过期或不存在,它将被重新编译并放置在视图存储目录中。 Blade 视图是重新编译原始视图更改的。 if (strpos($this->path, BLADE_EXT) !== false) { $this->path = $this->compile(); } try {include $this->path;} catch(\Exception $e) {ob_get_clean(); throw $e;} return ob_get_clean(); } /** * Get the array of view data for the view instance. * 获取视图实例的视图数据数组。 * The shared view data will be combined with the view data for the instance. * 共享视图数据将与实例的视图数据合并。 * @return array */ protected function data() { $data = array_merge($this->data, static::$shared); // All nested views and responses are evaluated before the main view. // This allows the assets used by nested views to be added to the // asset container before the main view is evaluated. // 在主视图之前评估所有嵌套视图和响应。这允许在评估主视图之前将嵌套视图使用的资产添加到资产容器。 foreach ($data as &$value) { if ($value instanceof View or $value instanceof Response) { $value = $value->render(); } } return $data; } /** * Get the path to the compiled version of the Blade view. * 获取 Blade 视图编译版本的路径。 * @return string */ protected function compile() { // Compiled views are stored in the storage directory using the MD5 // hash of their path. This allows us to easily store the views in // the directory without worrying about structure. // 使用MD5将编译后的视图存储在存储目录中 // 他们路径的哈希值。 这使我们可以轻松地将视图存储在目录中,而无需担心结构。 $compiled = path('storage').'views/'.md5($this->path); // The view will only be re-compiled if the view has been modified // since the last compiled version of the view was created or no // compiled view exists at all in storage. // 只有在创建视图的上次编译版本后视图已被修改或存储中根本不存在编译视图时,才会重新编译视图。 if ( ! file_exists($compiled) or (filemtime($this->path) > filemtime($compiled))) { file_put_contents($compiled, Blade::compile($this->path)); } return $compiled; } /** * Add a view instance to the view data. * 将视图实例添加到视图数据。 ** // Add a view instance to a view's data * $view = View::make('foo')->nest('footer', 'partials.footer'); * * // Equivalent functionality using the "with" method * $view = View::make('foo')->with('footer', View::make('partials.footer')); *
* * @param string $key * @param string $view * @param array $data * @return View */ public function nest($key, $view, $data = array()) { return $this->with($key, static::make($view, $data)); } /** * Add a key / value pair to the view data. * 向视图数据添加键/值对。 * Bound data will be available to the view as variables. * 绑定数据将作为变量提供给视图。 * @param string $key * @param mixed $value * @return View */ public function with($key, $value) { $this->data[$key] = $value; return $this; } /** * Add a key / value pair to the shared view data. * 将键/值对添加到共享视图数据中。 * Shared view data is accessible to every view created by the application. * 应用程序创建的每个视图都可以访问共享视图数据。 * @param string $key * @param mixed $value * @return void */ public static function share($key, $value) { static::$shared[$key] = $value; } /** * Implementation of the ArrayAccess offsetExists method. * ArrayAccess offsetExists 方法的实现。 */ public function offsetExists($offset) { return array_key_exists($offset, $this->data); } /** * Implementation of the ArrayAccess offsetGet method. * ArrayAccess offsetGet方法的实现。 */ public function offsetGet($offset) { if (isset($this[$offset])) return $this->data[$offset]; } /** * Implementation of the ArrayAccess offsetSet method. * ArrayAccess offsetSet 方法的实现。 */ public function offsetSet($offset, $value) { $this->data[$offset] = $value; } /** * Implementation of the ArrayAccess offsetUnset method. * ArrayAccess offsetUnset 方法的实现。 */ public function offsetUnset($offset) { unset($this->data[$offset]); } /** * Magic Method for handling dynamic data access. * 处理动态数据访问的魔术方法。 */ public function __get($key) { return $this->data[$key]; } /** * Magic Method for handling the dynamic setting of data. * 处理数据动态设置的魔术方法。 */ public function __set($key, $value) { $this->data[$key] = $value; } /** * Magic Method for checking dynamically-set data. * 用于检查动态设置数据的魔术方法。 */ public function __isset($key) { return isset($this->data[$key]); } /** * Get the evaluated string content of the view. * 获取视图的评估字符串内容。 * @return string */ public function __toString() { return $this->render(); }}
github地址:
转载地址:https://liushilong.blog.csdn.net/article/details/117396789 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
逛到本站,mark一下
[***.202.152.39]2024年05月03日 15时42分30秒
关于作者
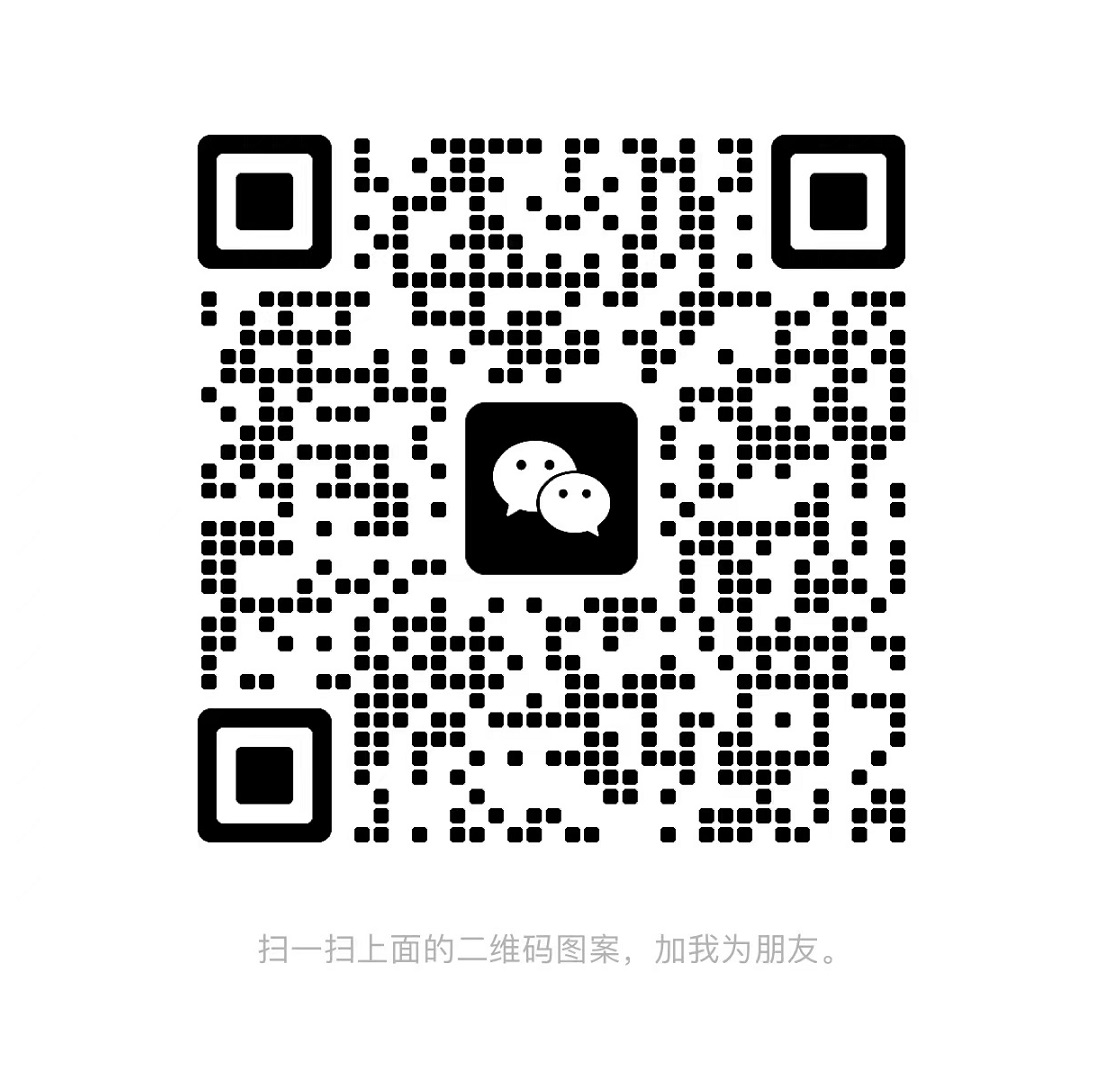
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
[fmt+shellcode]string
2019-04-30
fmt在bss段(neepusec_easy_format)
2019-04-30
[double free] 9447 CTF : Search Engine
2019-04-30
python 函数式编程
2019-04-30
python编码
2019-04-30
flink 1-个人理解
2019-04-30
redis cli
2019-04-30
redis api
2019-04-30
flink physical partition
2019-04-30
java 解析json
2019-04-30
java http请求
2019-04-30
tensorflow 数据格式
2019-04-30
tf rnn layer
2019-04-30
tf input layer
2019-04-30
tf model create
2019-04-30
tf dense layer两种创建方式的对比和numpy实现
2019-04-30
tf initializer
2019-04-30
tf 从RNN到BERT
2019-04-30
tf keras SimpleRNN源码解析
2019-04-30