
【Laravel3.0.0源码阅读分析】字符串类str.php
发布日期:2021-06-30 20:44:45
浏览次数:2
分类:技术文章
本文共 7332 字,大约阅读时间需要 24 分钟。
* // Get the length of a string * $length = Str::length('Taylor Otwell'); * * // Get the length of a multi-byte string * $length = Str::length('Τάχιστη') * * * @param string $value * @return int */ public static function length($value) { // mb_strlen-获取字符串长度 return (MB_STRING) ? mb_strlen($value, static::encoding()) : strlen($value); } /** * Convert a string to lowercase. * 将字符串转换为小写。 ** // Convert a string to lowercase * $lower = Str::lower('Taylor Otwell'); * * // Convert a multi-byte string to lowercase * $lower = Str::lower('Τάχιστη'); *
* * @param string $value * @return string */ public static function lower($value) { // mb_strtolower-使字符串小写 return (MB_STRING) ? mb_strtolower($value, static::encoding()) : strtolower($value); } /** * Convert a string to uppercase. * 将字符串转换为大写。 ** // Convert a string to uppercase * $upper = Str::upper('Taylor Otwell'); * * // Convert a multi-byte string to uppercase * $upper = Str::upper('Τάχιστη'); *
* * @param string $value * @return string */ public static function upper($value) { // mb_strtoupper-使字符串大写 return (MB_STRING) ? mb_strtoupper($value, static::encoding()) : strtoupper($value); } /** * Convert a string to title case (ucwords equivalent). * 将字符串转换为标题大小写(ucwords 等效) ** // Convert a string to title case * $title = Str::title('taylor otwell'); * * // Convert a multi-byte string to title case * $title = Str::title('νωθρού κυνός'); *
* * @param string $value * @return string */ public static function title($value) { if (MB_STRING) { // mb_convert_case-对字符串大小写进行转换 return mb_convert_case($value, MB_CASE_TITLE, static::encoding()); } // ucwords-对字符串每个单词的首字母转换为大写 return ucwords(strtolower($value)); } /** * Limit the number of characters in a string. * 限制字符串中的字符数。 ** // Returns "Tay..." * echo Str::limit('Taylor Otwell', 3); * * // Limit the number of characters and append a custom ending * echo Str::limit('Taylor Otwell', 3, '---'); *
* * @param string $value * @param int $limit * @param string $end * @return string */ public static function limit($value, $limit = 100, $end = '...') { if (static::length($value) <= $limit) return $value; if (MB_STRING) { return mb_substr($value, 0, $limit, static::encoding()).$end; } return substr($value, 0, $limit).$end; } /** * Limit the number of words in a string. * 限制字符串中的单词数。 ** // Returns "This is a..." * echo Str::words('This is a sentence.', 3); * * // Limit the number of words and append a custom ending * echo Str::words('This is a sentence.', 3, '---'); *
* * @param string $value * @param int $words * @param string $end * @return string */ public static function words($value, $words = 100, $end = '...') { preg_match('/^\s*+(?:\S++\s*+){1,'.$words.'}/', $value, $matches); if (static::length($value) == static::length($matches[0])) { $end = ''; } return rtrim($matches[0]).$end; } /** * Get the singular form of the given word. * 获取给定单词的单数形式。 * The word should be defined in the "strings" configuration file. * * @param string $value * @return string */ public static function singular($value) { $inflection = Config::get('strings.inflection'); // array_flip-交换数组中的键和值 $singular = array_get(array_flip($inflection), strtolower($value), $value); // ctype_upper-做大写字母检测 return (ctype_upper($value[0])) ? static::title($singular) : $singular; } /** * Get the plural form of the given word. * 获取给定单词的复数形式。 * The word should be defined in the "strings" configuration file. * 这个词应该在“strings”配置文件中定义。 ** // Returns the plural form of "child" * $plural = Str::plural('child', 10); * * // Returns the singular form of "octocat" since count is one * $plural = Str::plural('octocat', 1); *
* * @param string $value * @param int $count * @return string */ public static function plural($value, $count = 2) { if ((int) $count == 1) return $value; $plural = array_get(Config::get('strings.inflection'), strtolower($value), $value); return (ctype_upper($value[0])) ? static::title($plural) : $plural; } /** * Generate a URL friendly "slug" from a given string. * 从给定的字符串生成一个 URL 友好的“slug”。 ** // Returns "this-is-my-blog-post" * $slug = Str::slug('This is my blog post!'); * * // Returns "this_is_my_blog_post" * $slug = Str::slug('This is my blog post!', '_'); *
* * @param string $title * @param string $separator * @return string */ public static function slug($title, $separator = '-') { $title = static::ascii($title); // Remove all characters that are not the separator, letters, numbers, or whitespace. // 删除除分隔符、字母、数字或空格以外的所有字符。 // preg_quote 转义正则表达式字符 $title = preg_replace('![^'.preg_quote($separator).'\pL\pN\s]+!u', '', static::lower($title)); // Replace all separator characters and whitespace by a single separator // 用单个分隔符替换所有分隔符和空格 $title = preg_replace('!['.preg_quote($separator).'\s]+!u', $separator, $title); return trim($title, $separator); } /** * Convert a string to 7-bit ASCII. * 将字符串转换为 7 位 ASCII。 * This is helpful for converting UTF-8 strings for usage in URLs, etc. * 这有助于转换 UTF-8 字符串以用于 URL 等。 * @param string $value * @return string */ public static function ascii($value) { $foreign = Config::get('strings.ascii'); // array_keys-数组中所有的键 array_values-数组中所有的值 $value = preg_replace(array_keys($foreign), array_values($foreign), $value); return preg_replace('/[^\x09\x0A\x0D\x20-\x7E]/', '', $value); } /** * Convert a string to an underscored, camel-cased class name. * 将字符串转换为带下划线的驼峰式类名。 * This method is primarily used to format task and controller names. * 此方法主要用于格式化任务和控制器名称。 ** // Returns "Task_Name" * $class = Str::classify('task_name'); * * // Returns "Taylor_Otwell" * $class = Str::classify('taylor otwell') *
* * @param string $value * @return string */ public static function classify($value) { $search = array('_', '-', '.'); return str_replace(' ', '_', static::title(str_replace($search, ' ', $value))); } /** * Return the "URI" style segments in a given string. * 返回给定字符串中的“URI”样式段。 * @param string $value * @return array */ public static function segments($value) { return array_diff(explode('/', trim($value, '/')), array('')); } /** * Generate a random alpha or alpha-numeric string. * 生成随机的字母或字母数字字符串。 ** // Generate a 40 character random alpha-numeric string * echo Str::random(40); * * // Generate a 16 character random alphabetic string * echo Str::random(16, 'alpha'); *
* * @param int $length * @param string $type * @return string */ public static function random($length, $type = 'alnum') { // str_shuffle 随机打乱一个字符串 str_repeat-重复一个字符串 substr-返回字符串的子串 return substr(str_shuffle(str_repeat(static::pool($type), 5)), 0, $length); } /** * Get the character pool for a given type of random string. * 获取给定类型随机字符串的字符池。 * @param string $type * @return string */ protected static function pool($type) { switch ($type) { case 'alpha': return 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; case 'alnum': return '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; default: throw new \Exception("Invalid random string type [$type]."); } }}
github地址:
转载地址:https://liushilong.blog.csdn.net/article/details/117397934 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
网站不错 人气很旺了 加油
[***.192.178.218]2024年04月06日 06时58分43秒
关于作者
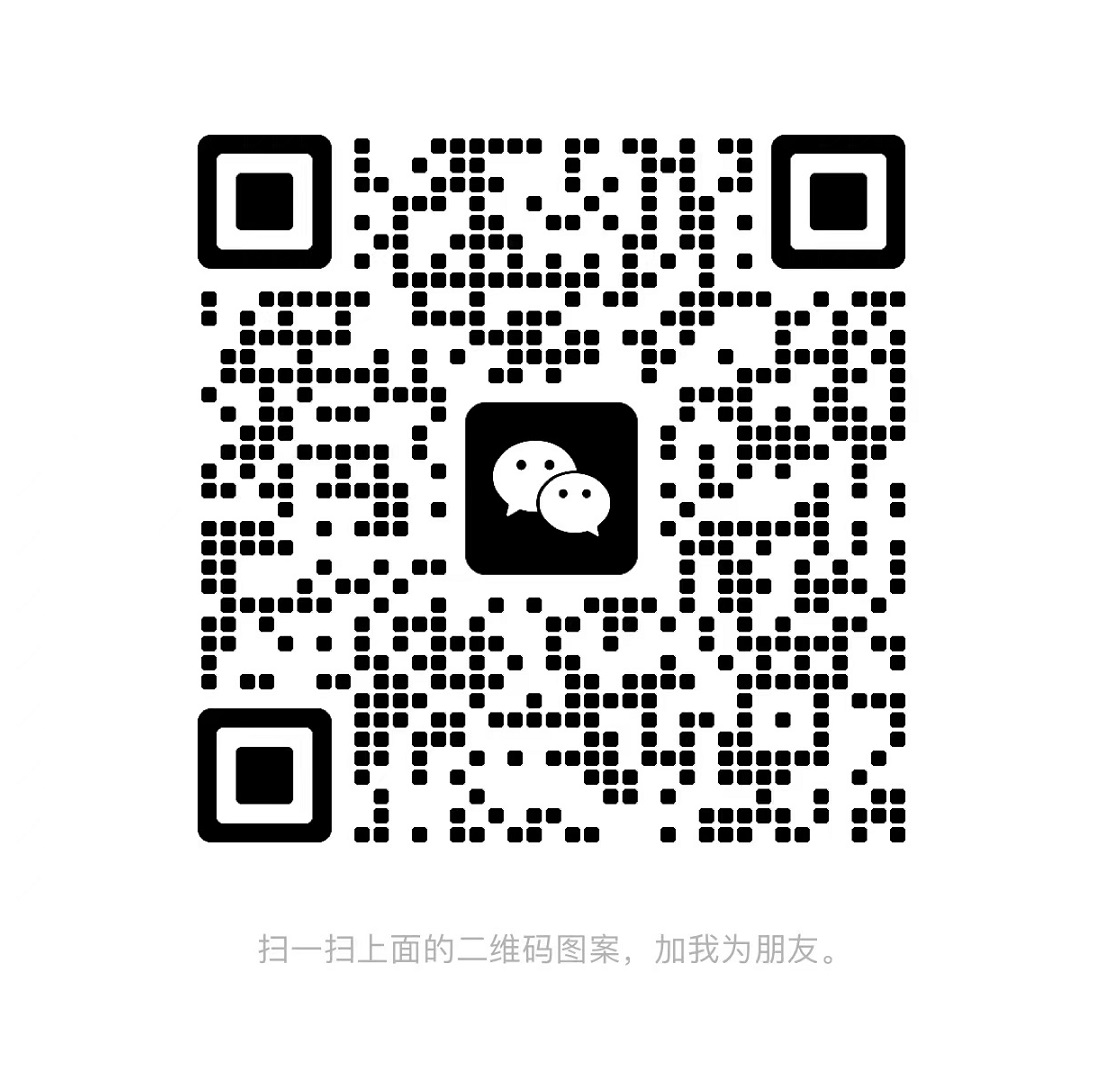
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
YAPF —— Python代码格式化工具
2019-04-30
ranger
2019-04-30
slurm
2019-04-30
Python计算文本BLEU分数
2019-04-30
torch.distributed 分布式
2019-04-30
MATLAB与CUDA
2019-04-30
Linux png转jpg (convert命令)
2019-04-30
NAS (Network Attached Storage 网络附属存储)
2019-04-30
Ubuntu更新后终端中字体的颜色全是白色
2019-04-30
vscode git
2019-04-30
基于MATLAB的二进制数字调制与解调信号的仿真——2PSK
2019-04-30
基于MATLAB的模拟调制信号与解调的仿真——DSB
2019-04-30
基于MATLAB的模拟调制信号与解调的仿真——SSB
2019-04-30
HDU - 1166 敌兵布阵 (树状数组模板题/线段树模板题)
2019-04-30
CodeForces - 761C Dasha and Password (思维 暴力)
2019-04-30
POJ - 2481 Cows (树状数组 入门题)
2019-04-30
CodeForces - 987C Three displays (暴力/dp)
2019-04-30
计蒜客 NAIPC 2016 F. Mountain Scenes(dp)
2019-04-30
牛客国庆集训派对Day4——I 连通块计数(思维)
2019-04-30