
Token插件:Auth0和jjwt对比
发布日期:2021-06-30 21:30:36
浏览次数:2
分类:技术文章
本文共 8971 字,大约阅读时间需要 29 分钟。
依赖包
com.auth0 java-jwt 3.10.0 io.jsonwebtoken jjwt-api 0.11.2 io.jsonwebtoken jjwt-impl 0.11.2 runtime io.jsonwebtoken jjwt-jackson 0.11.2 runtime
Auto0工具类
import com.auth0.jwt.JWT;import com.auth0.jwt.JWTCreator;import com.auth0.jwt.algorithms.Algorithm;import com.auth0.jwt.exceptions.JWTVerificationException;import com.auth0.jwt.interfaces.DecodedJWT;import com.auth0.jwt.interfaces.JWTVerifier;import java.util.Date;import java.util.HashMap;import java.util.Map;/** * @author: lizz * @date: 2020/1/20 09:58 */public class Auth0Util { /** * 引入apollo的配置 */ private static String TOKEN_KEY = "54f12048-1f56-45c1-a3f1-2c546bc2bb42"; /** * jwt有效时间 */ private static long TOKEN_TIMEOUT = 10* 60 * 1000; /** * jwt生成方 */ private final static String JWT_ISSUER = "lb-fw-gw"; /** * 生成jwt * * @param header * @return */ public static String create(Mapheader) { return create(header, new HashMap<>(0), JWT_ISSUER, TOKEN_TIMEOUT); } public static String create(Map header, Map claims) { return create(header, claims, JWT_ISSUER, TOKEN_TIMEOUT); } public static String create(Map header, long timeout) { return create(header, new HashMap<>(0), JWT_ISSUER, timeout); } /** * 生成jwt * * @param header * @param issuer * @return */ public static String create(Map header, Map claims, String issuer, long timeout) { String token; try { Algorithm algorithm = Algorithm.HMAC256(TOKEN_KEY); Date date = new Date(System.currentTimeMillis() + timeout); JWTCreator.Builder builder = JWT.create() .withHeader(header) .withIssuer(issuer) .withExpiresAt(date); for (String key : claims.keySet()) { builder.withClaim(key, claims.get(key)); } token = builder.sign(algorithm); } catch (JWTVerificationException exception) { System.out.println(exception.getMessage()); return null; } return token; } /** * 验证jwt * * @param token * @return */ public static DecodedJWT decode(String token) { DecodedJWT jwt; try { Algorithm algorithm = Algorithm.HMAC256(TOKEN_KEY); JWTVerifier verifier = JWT.require(algorithm)// .withIssuer(JWT_ISSUER)// .acceptLeeway(1)// .acceptExpiresAt(1)// .acceptIssuedAt(1)// .acceptNotBefore(1) .build(); jwt = verifier.verify(token); } catch (JWTVerificationException exception) { System.out.println(exception.getMessage()); return null; } return jwt; }}
jjwt工具类
import io.jsonwebtoken.*;import io.jsonwebtoken.security.Keys;import javax.crypto.SecretKey;import javax.crypto.spec.SecretKeySpec;import java.security.Key;import java.util.Date;import java.util.HashMap;import java.util.Map;/** * @description: jsonwebtoken工具类 * @author: lizz * @date: 2020/2/25 4:18 下午 */public class JsonWebTokenUtil { /** * jwt密钥 必须大于32个字符 */ private static String TOKEN_KEY = "54f12048-1f56-45c1-a3f1-2c546bc2bb42"; /** * jwt有效时间 */ private static long TOKEN_TIMEOUT = 60 * 30 * 1000; /** * jwt生成方 */ private final static String JWT_ISSUER = "lb-fw-gw"; /** * 生成jwt * * @param header * @return */ public static String create(Mapheader) { return create(header, new HashMap<>(2), JWT_ISSUER, TOKEN_TIMEOUT); } public static String create(Map header, Map claims) { return create(header, claims, JWT_ISSUER, TOKEN_TIMEOUT); } public static String create(Map header, long timeout) { return create(header, new HashMap<>(2), JWT_ISSUER, timeout); } public static String create(Map header, Map claims, long timeout) { return create(header, claims, JWT_ISSUER, timeout); } /** * 生成jwt * * @param header * @return */ public static String create(Map header, Map claims, String issuer, long timeout) { String token; try { Date date = new Date(System.currentTimeMillis() + timeout); SecretKey key = Keys.hmacShaKeyFor(TOKEN_KEY.getBytes()); token = Jwts.builder() .setHeader(header) .setClaims(claims) .setIssuer(issuer) .setExpiration(date) .signWith(key, SignatureAlgorithm.HS256) .compact(); } catch (JwtException exception) { System.out.println(exception.getMessage()); return null; } return token; } /** * 验证jwt * * @param token * @return */ public static Jws decode(String token) { Jws claimsJws; try { Key key = new SecretKeySpec(TOKEN_KEY.getBytes(), SignatureAlgorithm.HS256.getJcaName()); claimsJws = Jwts.parserBuilder().setSigningKey(key).build().parseClaimsJws(token); } catch (JwtException exception) { System.out.println(exception.getMessage()); return null; } return claimsJws; }}
单测
package com.lizz.gateway;import com.lizz.gateway.util.Auth0Util;import com.lizz.gateway.util.JsonWebTokenUtil;import org.junit.jupiter.api.Test;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import java.util.HashMap;import java.util.Map;/** * @description: jwt插件对比 auth0 and jjwt * @author: lizz * @date: 2020/10/28 17:47 */public class OnceTester { private Logger logger = LoggerFactory.getLogger(OnceTester.class); private static Mapheader = new HashMap () { { put("auth", "level1"); }}; private static Map claims = new HashMap () { { put("user", "xxxs"); }}; private static String token; @Test public void jwtTest() { //需要根据当前时间生成jwt,否则解析会失效 token = JsonWebTokenUtil.create(header, claims); auth0Create(10000); jjwtCreate(10000); auth0Create(100000); jjwtCreate(100000); auth0Create(1000000); jjwtCreate(1000000); auth0Decode(10000); jjwtDecode(10000); auth0Decode(100000); jjwtDecode(100000); auth0Decode(1000000); jjwtDecode(1000000); } /** * 生成jwt * * @param times 次数 */ private void auth0Create(int times) { long btime = System.currentTimeMillis(); for (int i = 1; i <= times; i++) { Auth0Util.create(header, claims); } logger.info("auth0 Create times:{},time:{}", times, System.currentTimeMillis() - btime); } /** * 生成jwt * * @param times 次数 */ private void jjwtCreate(int times) { long btime = System.currentTimeMillis(); for (int i = 1; i <= times; i++) { JsonWebTokenUtil.create(header, claims); } logger.info("jjwt Create times:{},time:{}", times, System.currentTimeMillis() - btime); } /** * 生成jwt * * @param times 次数 */ private void auth0Decode(int times) { long btime = System.currentTimeMillis(); for (int i = 1; i <= times; i++) { Auth0Util.decode(token); } logger.info("auth0 decode times:{},time:{}", times, System.currentTimeMillis() - btime); } /** * 生成jwt * * @param times 次数 */ private void jjwtDecode(int times) { long btime = System.currentTimeMillis(); for (int i = 1; i <= times; i++) { JsonWebTokenUtil.decode(token); } logger.info("jjwt decode times:{},time:{}", times, System.currentTimeMillis() - btime); }}
18:29:52.217 [main] INFO com.lizz.gateway.OnceTester - auth0 Create times:10000,time:156018:29:55.294 [main] INFO com.lizz.gateway.OnceTester - jjwt Create times:10000,time:306818:29:58.062 [main] INFO com.lizz.gateway.OnceTester - auth0 Create times:100000,time:276818:30:11.734 [main] INFO com.lizz.gateway.OnceTester - jjwt Create times:100000,time:1367218:30:27.485 [main] INFO com.lizz.gateway.OnceTester - auth0 Create times:1000000,time:1575118:32:38.006 [main] INFO com.lizz.gateway.OnceTester - jjwt Create times:1000000,time:13052018:32:39.181 [main] INFO com.lizz.gateway.OnceTester - auth0 decode times:10000,time:117518:32:43.956 [main] INFO com.lizz.gateway.OnceTester - jjwt decode times:10000,time:477518:32:45.932 [main] INFO com.lizz.gateway.OnceTester - auth0 decode times:100000,time:197618:33:23.977 [main] INFO com.lizz.gateway.OnceTester - jjwt decode times:100000,time:3804518:33:38.295 [main] INFO com.lizz.gateway.OnceTester - auth0 decode times:1000000,time:1431818:40:11.813 [main] INFO com.lizz.gateway.OnceTester - jjwt decode times:1000000,time:393518
测试数据
取3次中位数。
操作 | decode | create | ||
---|---|---|---|---|
次数 | auth0 | jsonweboken | auth0 | jsonwebtoken |
1,000 | 1665 ms | 2842 ms | 2306 | 2398 ms |
10,000 | 2951 ms | 9755 ms | 3952 | 5246 ms |
100,000 | 5786ms | 38859 ms | 11784 | 16191 ms |
结论
- 性能方面auth0更优。
- 功能方面jsonwebtoken支持更高级的应用,如高级加密算法等。
转载地址:https://lizz6.blog.csdn.net/article/details/104614942 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
表示我来过!
[***.240.166.169]2024年04月13日 22时13分21秒
关于作者
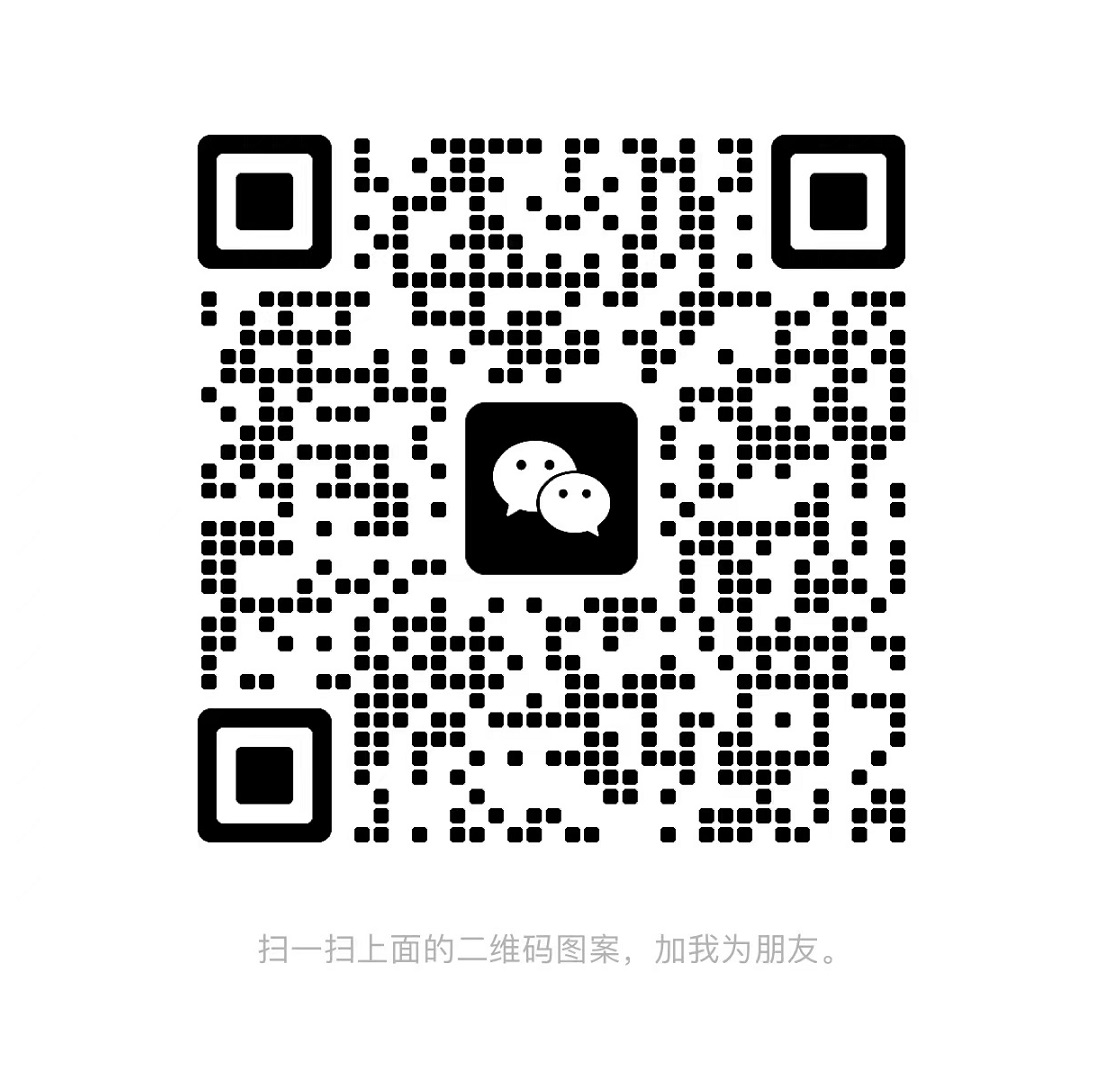
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
简析 __init__、__new__、__call__ 方法
2019-05-01
程序员如何优雅地写公众号
2019-05-01
@classmethod与@staticmethod的区别
2019-05-01
只有1%的程序员搞懂过浮点数陷阱
2019-05-01
Google 为什么把几十亿行代码放在一个库
2019-05-01
Erlang 之父 Joe Armstrong 去世
2019-05-01
来不及了,世界读书日,送10本书
2019-05-01
速来,上期中奖名单
2019-05-01
一名 Google 工程师的大数据处理经验
2019-05-01
30分钟学会pyecharts数据可视化
2019-05-01
从一个骗子身上学到的
2019-05-01
关于Python爬虫,这里有一条高效的学习路径
2019-05-01
Python学习指南,看这篇清晰多了!
2019-05-01
Oracle裁员,3点建议
2019-05-01
「忙」只是借口
2019-05-01
如果只有1小时学Python,看这篇就够了
2019-05-01
命名难,难于上青天
2019-05-01
记一件小事
2019-05-01
一个牛逼的数据库操作命令行工具:mycli
2019-05-01
掌握 Python 爬虫的所有技巧,都在这里!
2019-05-01