
LeetCode C++ 98. Validate Binary Search Tree【二叉搜索树/DFS】中等
发布日期:2021-07-01 02:51:55
浏览次数:2
分类:技术文章
本文共 2211 字,大约阅读时间需要 7 分钟。
Given a binary tree, determine if it is a valid binary search tree (BST). Assume a BST is defined as follows:
- The left subtree of a node contains only nodes with keys less than the node’s key.
- The right subtree of a node contains only nodes with keys greater than the node’s key.
- Both the left and right subtrees must also be binary search trees.
Example 1:
2 / \ 1 3Input: [2,1,3]Output: true
Example 2:
5 / \ 1 4 / \ 3 6Input: [5,1,4,null,null,3,6]Output: falseExplanation: The root node's value is 5 but its right child's value is 4.
题意:验证给出的二叉树是否是二叉搜索树。
思路1:递归中序遍历。如果这是一棵二叉搜索树,那么递归中序遍历时,前驱结点的值一定小于后继结点的值。至于如何保持前驱结点,应该在二叉树线索化的时候学习过(有时间也会写一下)。代码如下:
class Solution { public: TreeNode *pre; bool isValidBST(TreeNode* root) { if (root == nullptr) return true; pre = nullptr; return inorder(root); } bool inorder(TreeNode *root) { if (root == nullptr) return true; if (inorder(root->left) == false) return false; if (pre && pre->val >= root->val) return false; pre = root; if (inorder(root->right) == false) return false; return true; }};
或者直接使用原函数:
class Solution { public: TreeNode *pre = nullptr; bool isValidBST(TreeNode* root) { if (root == nullptr) return true; if (isValidBST(root->left) == false) return false; if (pre && pre->val >= root->val) return false; pre = root; if (isValidBST(root->right) == false) return false; return true; } };
递归函数的时间效率较低:
执行用时:20 ms, 在所有 C++ 提交中击败了36.41% 的用户内存消耗:17.9 MB, 在所有 C++ 提交中击败了35.56% 的用户
思路2:非递归中序遍历。代码如下:
class Solution { public: bool isValidBST(TreeNode* root) { if (root == nullptr) return true; TreeNode *pre = nullptr, *cur = root; stackst; while (cur || !st.empty()) { while (cur) { st.push(cur); cur = cur->left; } cur = st.top(); st.pop(); if (pre && pre->val >= cur->val) return false; pre = cur; cur = cur->right; } return true; } };
时间效率更高:
执行用时:12 ms, 在所有 C++ 提交中击败了92.82% 的用户内存消耗:18.1 MB, 在所有 C++ 提交中击败了16.36% 的用户
转载地址:https://memcpy0.blog.csdn.net/article/details/108733071 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
路过,博主的博客真漂亮。。
[***.116.15.85]2024年05月04日 13时04分22秒
关于作者
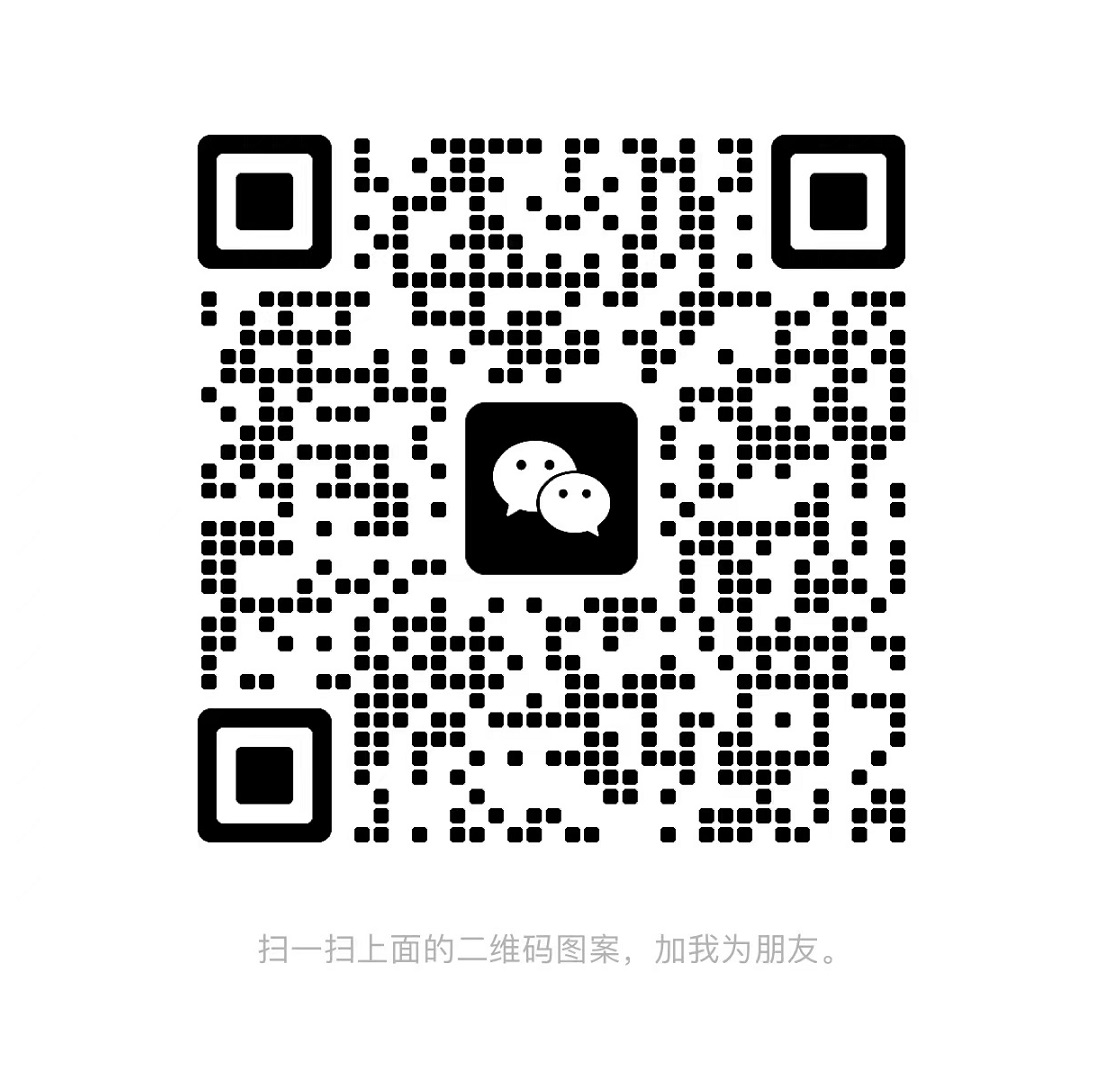
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
《unix环境高级编程》信号——sigaction 函数
2019-05-02
《unix环境高级编程》信号——sigsetjmp 函数和 siglongjmp 函数
2019-05-02
《unix高级环境编程》信号——sigsuspend 函数
2019-05-02
《unix高级环境编程》高级 I/O——记录锁
2019-05-02
《unix高级环境编程》高级 I/O—— I/O 多路复用
2019-05-02
《unix高级环境编程》高级 I/O—— readv 和 writev 函数
2019-05-02
《unix高级环境编程》高级 I/O—— 存储映射 I/O
2019-05-02
《unix高级环境编程》进程间通信——管道和FIFO
2019-05-02
《网络协议》IP 分片与 TCP 分段
2019-05-02
《网络协议》UDP 协议
2019-05-02
《网络协议》TCP 协议
2019-05-02
《网络协议》图解 TCP 连接建立与释放
2019-05-02
《网络协议》TCP 的交互数据流
2019-05-02
《网络协议》TCP 的成块数据流
2019-05-02
《网络协议》TCP 四种定时器
2019-05-02
《网络协议》TCP 拥塞控制
2019-05-02
《网络编程》关于 UNIX网络编程 卷1 的 unp.h 和源码编译问题
2019-05-02
《网络编程》套接字编程简介
2019-05-02
《网络编程》基本 TCP 套接字编程
2019-05-02
《网络编程》基于 TCP 套接字编程的分析
2019-05-02