
JS:ES6(ES2015)新特性之常量、箭头函数、解构赋值
发布日期:2021-07-01 06:13:41
浏览次数:4
分类:技术文章
本文共 3577 字,大约阅读时间需要 11 分钟。
几个概念
1、JavaScript VS ECMAScript
ECMAScript 一种标准
JavaScript 一种标准的实现2、ES6 == ES2015
ES6 版本号
ES2015 发布年份3、新特性
常量 Const 箭头函数 Arrow Function 解构赋值 Desctructuring4、准备工具
开发工具 VSCode 插件 Live Server 浏览器 Chromeconst常量
// ES3声明常量,可以被修改var BASE_NAME = "Tom";BASE_NAME = "jack";console.log(BASE_NAME);// jack// ES5声明常量,不可以被修改Object.defineProperty(window, "es", { value: "Tom", writable: false});es = "Jack";console.log(es);// Tom// ES6声明常量,不可以被修改const es = "Tom";es = "Jack"; // Uncaught TypeError: Assignment to constant variable.
const声明常量的特性:
- 如果声明的时候没有赋值,会抛出异常
- 不允许重复声明
- 不属于顶层对象window
- 不存在变量提升
- 必须先定义再使用,暂时性死区
- 存在块级作用域
如果const声明的是引用类型,内部元素可以被改变,
需要使用冻结
,防止内部元素被改变 浅层冻结
const obj = { name: "Tom"};// 浅层冻结Object.freeze(obj);const arr = ['Tom'];// 浅层冻结Object.freeze(arr);obj.name = "Jack";arr[0] = "Jack";console.log(obj);console.log(arr);
深层递归冻结
/*** 深层递归冻结*/function deepFreeze(obj){ Object.freeze(obj); Object.keys(obj).forEach(key=>{ if(typeof obj[key] === 'object'){ deepFreeze(obj[key]); } })}const obj = { name: "Tom", pets: ["dog", "cat"]};// Object.freeze(obj);deepFreeze(obj);obj.pets[0] = "pig";console.log(obj);
声明变量关键字:
优先使用const,如果需要被改变再使用let箭头函数
定义函数
function sum1(x, y){ return x + y;}const sum2 = function(x, y){ return x + y;}// 使用箭头函数const sum3 = (x, y) => { return x + y;}const sum4 = (x, y) => x + y;const sum5 = x => { /*方法体*/};
以下情况不适用箭头函数
场景1: 事件回调函数
场景2:对象内方法
const person = { name: "Tom", showName: function(){ console.log(this.name); }}person.showName();
场景3:函数内使用arguments
function sum(x, y){ console.log(arguments); return x + y;}sum(1, 2);
场景4:构造函数、原型方法
function Person(name, age){ this.name = name; this.age = age;}Person.prototype.showName = function(){ console.log(this.name);}const person = new Person("Tom", 23);person.showName();console.log(person);
解构赋值
等号左右两边结构要完全一致
// 对象解构赋值const obj = { name: "Tom", age: 23,};// const name = obj.name;// const age = obj.age;const { name, age } = obj;console.log(name, age);// 数组解构赋值const arr = [0, 1, 2];// const a = arr[0];// const b = arr[1];// const c = arr[2];const [a, b, c] = arr;console.log(a, b, c);
2、结构赋值取别名
const obj = { name: "Tom", age: 23, pet: { name: "bigo", }, }; // pet.name 别名 petName const { name, age, pet: { name: petName }, } = obj; console.log(name, age, petName);
3、解构赋值应用
(1)作为数组参数
function sum(arr){ let result = 0; for (let i = 0; i < arr.length; i++) { result += arr[i]; } console.log(result);}const arr = [1, 2, 3];sum(arr);// 改写如下function sum([a, b, c]) { console.log(a + b + c);}const arr = [1, 2, 3];sum(arr);
(2)作为对象参数
// pet有默认参数function foo({ name, age, pet = "pig" }) { console.log(name, age, pet);}const obj = { name: "Tom", age: 23,};foo(obj);
(3)作为函数返回值
function foo() { return { name: "Tom", age: 23, };}const { name, age} = foo();console.log(name, age);
(4)交换变量的值
let a = 1;let b = 2;[a, b] = [b, a];console.log(a, b);
(5)解析json时使用
const res = '{"name": "Tom", "age": 23}';const { name, age} = JSON.parse(res);console.log(name, age);
(6)Ajax请求应用
data.json
{ "name": "Tom", "age": 23}
Babel浏览器兼容
ES6 -> Babel -> ES5
环境配置
1、环境 Node.js 2、初始化 npm init -y3、安装依赖
npm install --save-dev babel-preset-env babel-cli# 或者npm i -D babel-preset-env babel-cli
3、创建配置文件
cat .babelrc{ "presets": ["env"]}
4、文件转化
文件:babel src/index.js -o dist/index.js文件夹:babel src -d dist实时监控:babel src -w -d dist
转载地址:https://pengshiyu.blog.csdn.net/article/details/108699408 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
表示我来过!
[***.240.166.169]2024年05月06日 13时26分19秒
关于作者
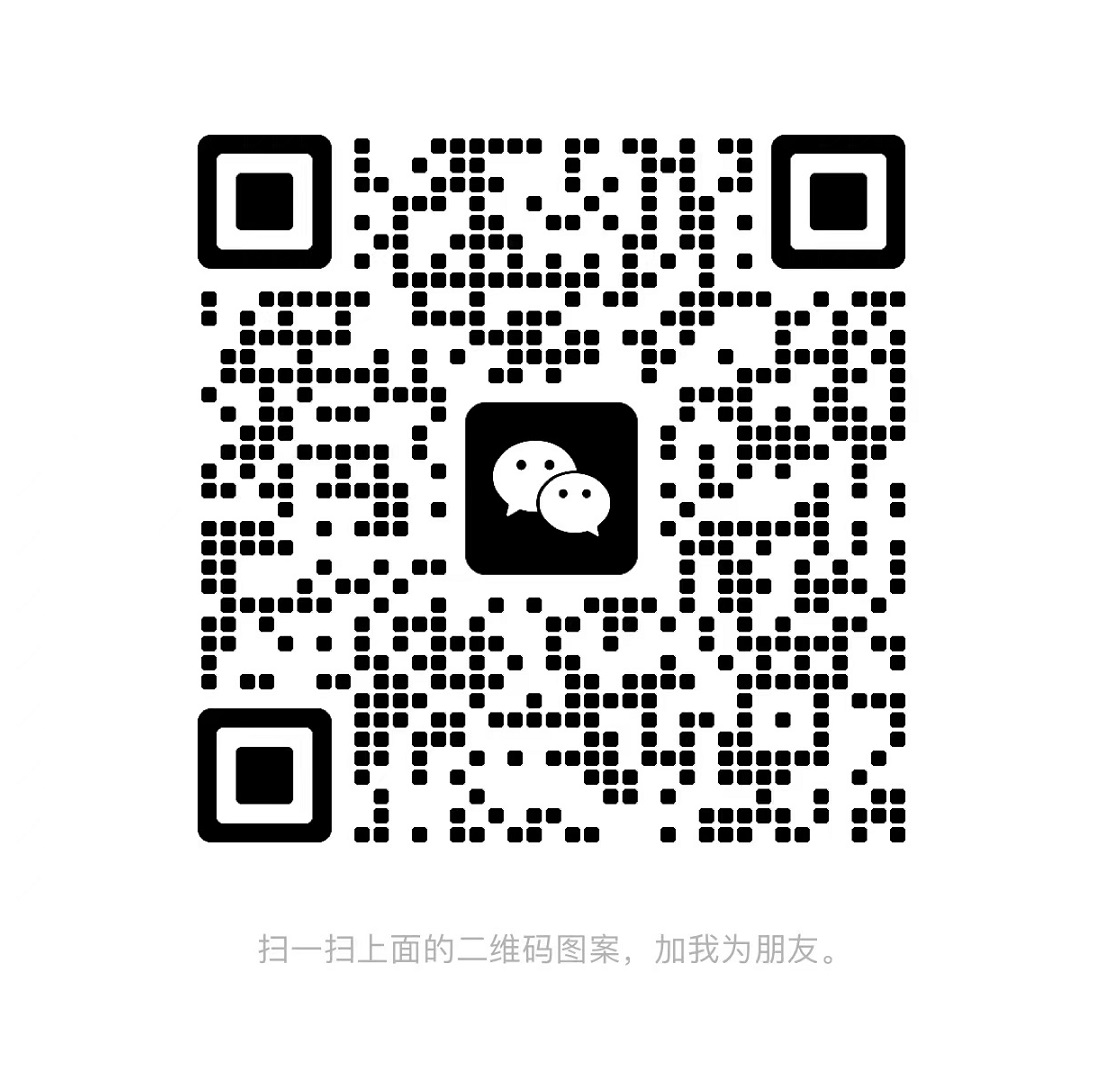
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
[12] JMeter-结果分析之图形图表
2019-05-01
使用aspose.words 18.6实现pdf文档转换
2019-05-01
Java数组详解
2019-05-01
vs中动态DLL与静态LIB工程中加入版本信息的方法
2019-05-01
大数据分析技术与应用一站式学习(值得收藏)_v20200418
2019-05-01
自定义Starter
2019-05-01
映 射 ALT 键
2019-05-01
vim使用快捷键F4生成文件头注释、F5生成main函数模板、F6生成.h文件框架模板
2019-05-01
OV5620的视频驱动
2019-05-01
C++中两个类交叉定义或递归定义的解决办法
2019-05-01
记一次Hive 行转列 引起的GC overhead limit exceeded
2019-05-01
OpenGL ES八 - 交叉存取顶点数据
2019-05-01
crontab定时任务写法
2019-05-01
nginx: [emerg] unknown directive "if($remote_addr" in /usr/local/tools/nginx/conf/nginx.conf:57
2019-05-01
module pip has no attribute main问题解决
2019-05-01
LeetCode 134.Gas Station (加油站)
2019-05-01
Python之命名元组 (namedtuple)
2019-05-01
使用libpcap过滤arp
2019-05-01
[转帖]Robots.txt指南
2019-05-01