
本文共 5935 字,大约阅读时间需要 19 分钟。
本文的内容来自iOS UICollectionView: The Complete Guide, Second Edition。文章对应的源码可以再处下载。
iOS UICollectionView: The Complete Guide摘要
基本
UICollectionViewFlowLayout基本参数设置
//configure our collection view layout UICollectionViewFlowLayout *flowLayout = (UICollectionViewFlowLayout *)self.collectionView.collectionViewLayout; flowLayout.minimumInteritemSpacing = 40.0f; flowLayout.minimumLineSpacing = 40.0f; flowLayout.sectionInset = UIEdgeInsetsMake(10, 10, 10, 10); flowLayout.itemSize = CGSizeMake(200, 200);
批量更新
使用performBatchUpdates:completion:
进行批量的更新,该方法默认有动画:
[self.collectionView performBatchUpdates:^{ //更新模型 NSDate *newDate = [NSDate date]; [datesArray insertObject:newDate atIndex:0]; //更新collection view [self.collectionView insertItemsAtIndexPaths:@[[NSIndexPath indexPathForItem:0 inSection:0]]]; } completion:nil];
prepareForReuse
prepareForReuse
方法重置cell的状态,先调用其super
方法
-(void)prepareForReuse{ [super prepareForReuse]; self.text = @"";}
selected、highlighted的理解
cell有两个重要的bool属性:selected和highlighted。highlighted完全由用户的交互决定,当用户的手指按下一个cell时,它就自动的变成highlighted。如果cell支持selection,当用户抬起他的手指,cell就变成selected。cell会保持selected,直到你写的一些代码使它unselected或者用户再次点击它。
UICollectionViewCell的层级关系如下所示:
- 黑色的为
UICollectionViewCell
本身,最前面的绿色view为contentView
,中间的为selectedBackgroundView与backgroundView - 如果cell被selected,selectedBackgroundView将会被添加到视图层级中
backgroundView如果被设置,就会永久的显示。当cell被selected,selectedBackgroundView就会被添加到view的层级中,当cell变成unselected,它就会被移除。
下面通过例子说明。通过如下代码来允许多选:
self.collectionView.allowsMultipleSelection = YES;
通过设置selectedBackgroundView来设置selected的背景view。重写setHighlighted来设置highlighted的样式。自定义的UICollectionViewCell如下:
@interface AFCollectionViewCell : UICollectionViewCell@property (nonatomic, strong) UIImage *image;@end@implementation AFCollectionViewCell{ UIImageView *imageView;}- (id)initWithFrame:(CGRect)frame{ if (!(self = [super initWithFrame:frame])) return nil; self.backgroundColor = [UIColor whiteColor]; imageView = [[UIImageView alloc] initWithFrame:CGRectInset(self.bounds, 10, 10)]; [self.contentView addSubview:imageView]; UIView *selectedBackgroundView = [[UIView alloc] initWithFrame:CGRectZero]; selectedBackgroundView.backgroundColor = [UIColor colorWithWhite:1.0f alpha:0.8f]; self.selectedBackgroundView = selectedBackgroundView; return self;}#pragma mark - Overriden UICollectionViewCell methods-(void)prepareForReuse{ [super prepareForReuse]; self.backgroundColor = [UIColor whiteColor]; self.image = nil; //also resets imageView’s image}-(void)setHighlighted:(BOOL)highlighted{ [super setHighlighted:highlighted]; if (self.highlighted) { imageView.alpha = 0.8f; } else { imageView.alpha = 1.0f; }}#pragma mark - Overridden Properties-(void)setImage:(UIImage *)image{ _image = image; imageView.image = image;}
运行的效果如下:
在上面的例子中,创建selectedBackgroundView
时,frame为CGRectZero,这样做是OK的,cell会自动拉伸它
collectionView的canCancelContentTouches
、delaysContentTouches
属性:
canCancelContentTouches
- 默认为YES,如果设置为NO的话,当开始tracking时,如果touch移动了,并不会来drag视图delaysContentTouches
- 默认为YES,如果设置为NO,会立即的调用touchesShouldBegin:withEvent:inContentView:
方法
Supplementary Views
Supplementary views 是指和cell一起滚动并显示某些信息的view
Supplementary views的显示的内容由数据源提供,由UICollectionViewLayout
对象布局
内置的UICollectionViewFlowLayout
提供了两种类型的Supplementary views:header和footer。
Class
或者UINib
,Supplementary views会被复用 如下,注册一个header
:
[surveyCollectionView registerClass:[AFCollectionHeaderView class] forSupplementaryViewOfKind:UICollectionElementKindSectionHeader withReuseIdentifier:HeaderIdentifier];
UICollectionElementKindSectionHeader
为内置的header类型,另一种为UICollectionElementKindSectionFooter
除此之外,还需要给Supplementary views指定一个大小
如下,通过flow layout的headerReferenceSize
指定header大小: surveyFlowLayout.headerReferenceSize = CGSizeMake(60, 50);
需要注意的是:当水平滚动时,使用的是CGSize
的width
,而header的垂直方向会被拉伸,填满整个空间。当垂直滚动时,使用的是CGSize
的height
,header水平方向填满整个区域,示意如下:
之后,实现UICollectionViewDelegate
的collectionView:viewForSupplementaryElementOfKind: atIndexPath:
方法,即可显示Supplementary views ,如下:
-(UICollectionReusableView *)collectionView:(UICollectionView *)collectionView viewForSupplementaryElementOfKind:(NSString *)kind atIndexPath:(NSIndexPath *)indexPath{ //Provides a view for the headers in the collection view AFCollectionHeaderView *headerView = (AFCollectionHeaderView *)[collectionView dequeueReusableSupplementaryViewOfKind:kind withReuseIdentifier:HeaderIdentifier forIndexPath:indexPath]; ......}
用户交互
一些常用的代理方法:
选择cell
-(void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath
修改collection view的内容
insertSections:deleteSections:reloadSections:moveSection:toSection:insertItemsAtIndexPaths:deleteItemsAtIndexPaths:reloadItemsAtIndexPaths:moveItemAtIndexPath:toIndexPath:
自定义selection行为
collectionView:shouldHighlightItemAtIndexPath:collectionView:shouldSelectItemAtIndexPath:collectionView:shouldDeselectItemAtIndexPath:
支持Cut/Copy/Paste
如下的例子,使collection view
的cell支持copy
1.使collection view
支持menu -(BOOL)collectionView:(UICollectionView *)collectionView shouldShowMenuForItemAtIndexPath:(NSIndexPath *)indexPath{ return YES;}
2.collection view
会询问代理对象,支持的action的类型。目前有cut:
copy:
和 paste:
-(BOOL)collectionView:(UICollectionView *)collectionView canPerformAction:(SEL)action forItemAtIndexPath:(NSIndexPath *)indexPath withSender:(id)sender{ if ([NSStringFromSelector(action) isEqualToString:@"copy:"]) { return YES; } return NO;}
3.执行action对应的操作
如下,执行copy操作-(void)collectionView:(UICollectionView *)collectionView performAction:(SEL)action forItemAtIndexPath:(NSIndexPath *)indexPath withSender:(id)sender{ if ([NSStringFromSelector(action) isEqualToString:@"copy:"]) { UIPasteboard *pasteboard = [UIPasteboard generalPasteboard]; [pasteboard setString:[[self photoModelForIndexPath:indexPath] name]]; }}
转载地址:https://windzen.blog.csdn.net/article/details/48621759 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关于作者
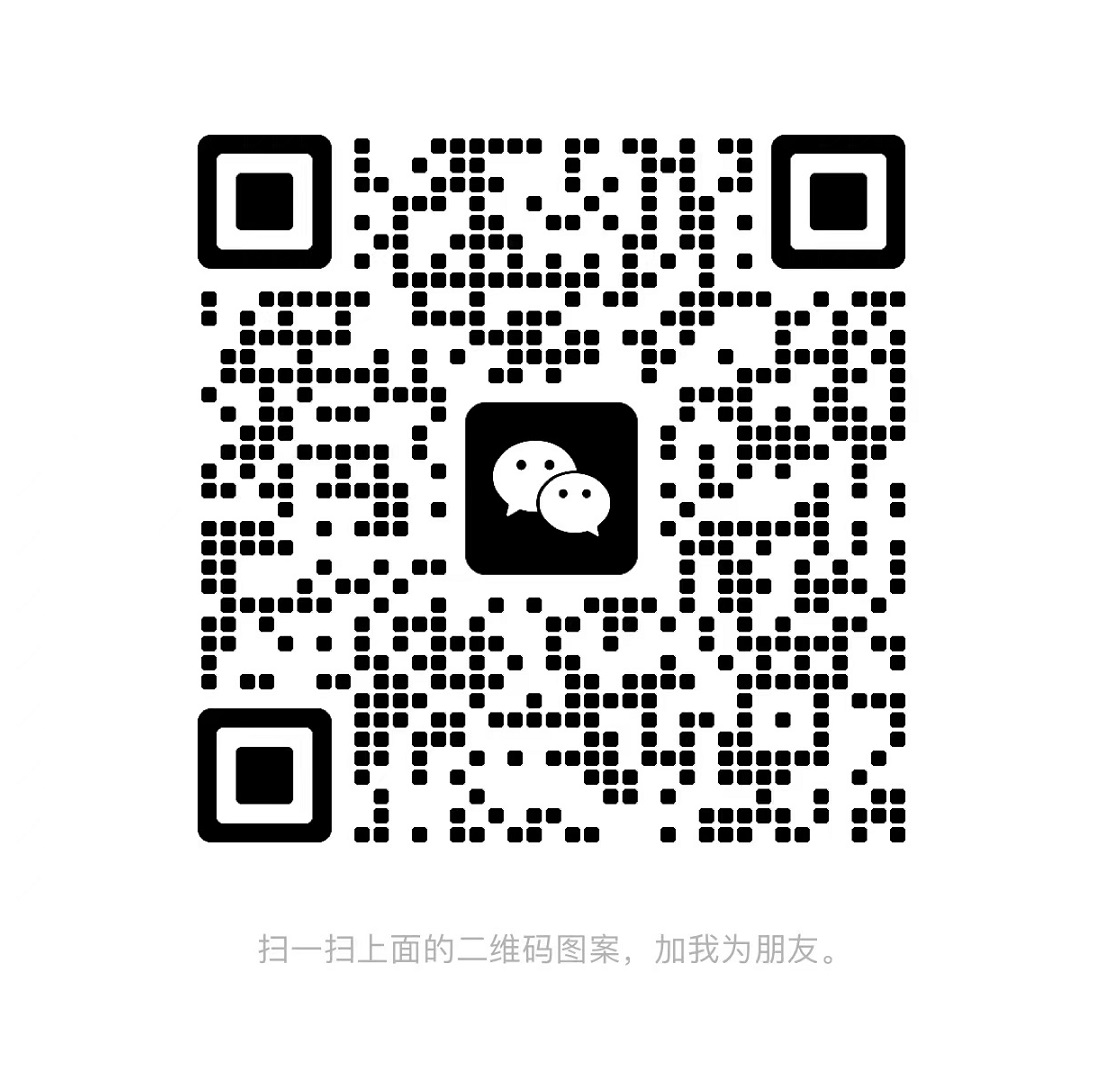