
Get ip address from hostname in C using Linux sockets
发布日期:2021-08-31 13:48:47
浏览次数:13
分类:技术文章
本文共 3271 字,大约阅读时间需要 10 分钟。
Here are 2 methods to get the ip address of a hostname :
The first method uses the traditional gethostbyname function to retrieve information about a hostname/domain name.
Code1 | #include<stdio.h> //printf |
2 | #include<string.h> //memset |
3 | #include<stdlib.h> //for exit(0); |
4 | #include<sys/socket.h> |
5 | #include<errno.h> //For errno - the error number |
6 | #include<netdb.h> //hostent |
7 | #include<arpa/inet.h> |
8 |
9 | int hostname_to_ip( char * , char *); |
10 |
11 | int main( int argc , char *argv[]) |
12 | { |
13 | if (argc <2) |
14 | { |
15 | printf ( "Please provide a hostname to resolve" ); |
16 | exit (1); |
17 | } |
18 | |
19 | char *hostname = argv[1]; |
20 | char ip[100]; |
21 | |
22 | hostname_to_ip(hostname , ip); |
23 | printf ( "%s resolved to %s" , hostname , ip); |
24 | |
25 | printf ( "\n" ); |
26 | |
27 | } |
28 | /* |
29 | Get ip from domain name |
30 | */ |
31 |
32 | int hostname_to_ip( char * hostname , char * ip) |
33 | { |
34 | struct hostent *he; |
35 | struct in_addr **addr_list; |
36 | int i; |
37 | |
38 | if ( (he = gethostbyname( hostname ) ) == NULL) |
39 | { |
40 | // get the host info |
41 | herror( "gethostbyname" ); |
42 | return 1; |
43 | } |
44 |
45 | addr_list = ( struct in_addr **) he->h_addr_list; |
46 | |
47 | for (i = 0; addr_list[i] != NULL; i++) |
48 | { |
49 | //Return the first one; |
50 | strcpy (ip , inet_ntoa(*addr_list[i]) ); |
51 | return 0; |
52 | } |
53 | |
54 | return 1; |
55 | } |
Compile and Run
1 | $ gcc hostname_to_ip.c && ./a.out www.google.com |
2 | www.google.com resolved to 74.125.235.16 |
3 |
4 | $ gcc hostname_to_ip.c && ./a.out www.msn.com |
5 | www.msn.com resolved to 207.46.140.34 |
6 |
7 | $ gcc hostname_to_ip.c && ./a.out www.yahoo.com |
8 | www.yahoo.com resolved to 98.137.149.56 |
The second method uses the getaddrinfo function to retrieve information about a hostname/domain name.
Code1 | #include<stdio.h> //printf |
2 | #include<string.h> //memset |
3 | #include<stdlib.h> //for exit(0); |
4 | #include<sys/socket.h> |
5 | #include<errno.h> //For errno - the error number |
6 | #include<netdb.h> //hostent |
7 | #include<arpa/inet.h> |
8 |
9 | int hostname_to_ip( char * , char *); |
10 |
11 | int main( int argc , char *argv[]) |
12 | { |
13 | if (argc <2) |
14 | { |
15 | printf ( "Please provide a hostname to resolve" ); |
16 | exit (1); |
17 | } |
18 | |
19 | char *hostname = argv[1]; |
20 | char ip[100]; |
21 | |
22 | hostname_to_ip(hostname , ip); |
23 | printf ( "%s resolved to %s" , hostname , ip); |
24 | |
25 | printf ( "\n" ); |
26 | |
27 | } |
28 | /* |
29 | Get ip from domain name |
30 | */ |
31 |
32 | int hostname_to_ip( char *hostname , char *ip) |
33 | { |
34 | int sockfd; |
35 | struct addrinfo hints, *servinfo, *p; |
36 | struct sockaddr_in *h; |
37 | int rv; |
38 |
39 | memset (&hints, 0, sizeof hints); |
40 | hints.ai_family = AF_UNSPEC; // use AF_INET6 to force IPv6 |
41 | hints.ai_socktype = SOCK_STREAM; |
42 |
43 | if ( (rv = getaddrinfo( hostname , "http" , &hints , &servinfo)) != 0) |
44 | { |
45 | fprintf (stderr, "getaddrinfo: %s\n" , gai_strerror(rv)); |
46 | return 1; |
47 | } |
48 |
49 | // loop through all the results and connect to the first we can |
50 | for (p = servinfo; p != NULL; p = p->ai_next) |
51 | { |
52 | h = ( struct sockaddr_in *) p->ai_addr; |
53 | strcpy (ip , inet_ntoa( h->sin_addr ) ); |
54 | } |
55 | |
56 | freeaddrinfo(servinfo); // all done with this structure |
57 | return 0; |
58 | } |
Compile and Run
1 | $ gcc hostname_to_ip.c && ./a.out www.google.com |
2 | www.google.com resolved to 74.125.235.19 |
3 |
4 | $ gcc hostname_to_ip.c && ./a.out www.yahoo.com |
5 | www.yahoo.com resolved to 72.30.2.43 |
6 |
7 | $ gcc hostname_to_ip.c && ./a.out www.msn.com |
8 | www.msn.com resolved to 207.46.140.34 |
转载地址:https://blog.csdn.net/weixin_34077371/article/details/90663146 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
能坚持,总会有不一样的收获!
[***.219.124.196]2024年04月27日 14时27分34秒
关于作者
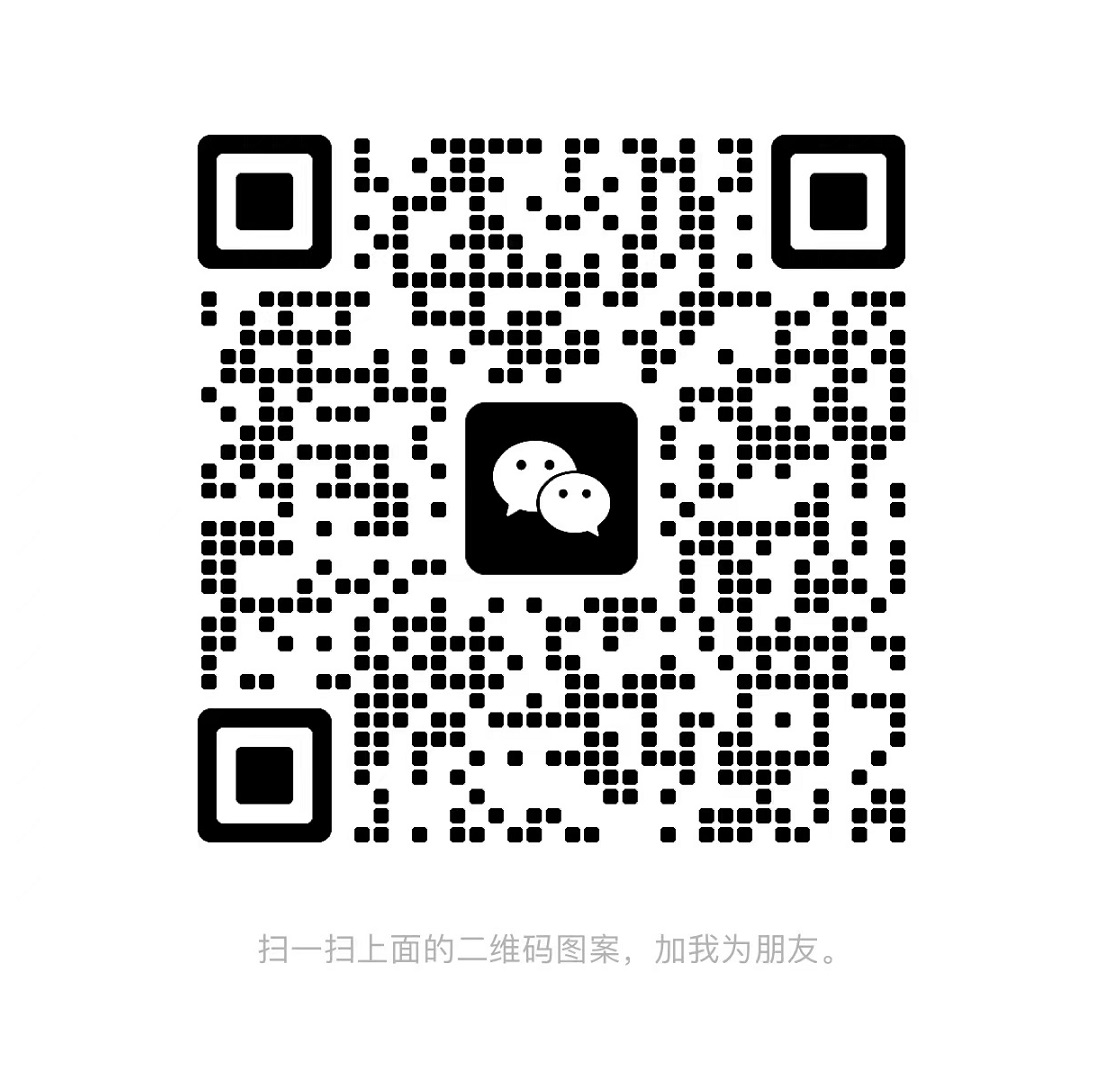
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!
推荐文章
树莓派网线直连
2019-04-29
复合材料培训(I第七期)
2019-04-29
复合材料生活中的应用
2019-04-29
ABAQUS复合材料(适合小白)
2019-04-29
ABAQUS高级案例解析
2019-04-29
人工智能药物研发
2019-04-29
【超级干货+福利】AIDD最全面的学习教程
2019-04-29
最新通知:AIDD与网络药理学资料大全
2019-04-29
Lammps分子动力学与第一性原理材料模拟及催化
2019-04-29
实习生小白的日常
2019-04-29
实习小白的日常(3)
2019-04-29
实习小白的日常(4)
2019-04-29
APP页面布局参考
2019-04-29
linux 的 Socket IO 模型
2019-04-29
APP调用服务器API设计
2019-04-29
Opencv+Zbar二维码识别(标准条形码/二维码识别)
2019-04-29
zbar优化
2019-04-29
微信扫码登录验证PHP代码(不用开放平台)
2019-04-29
CH554E USB单片机 10引脚小封装低成本USB方案
2019-04-29