
本文共 7318 字,大约阅读时间需要 24 分钟。
spring2+struts2+hibernate3实现连接两个数据库
jdbc.properties文件,写数据库的连接信息
jdbc.driverClassName=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://127.0.0.1:3306/destoon?useUnicode=true&characterEncoding=utf-8
jdbc.username=root
jdbc.password=123456
sql.driverClassName=net.sourceforge.jtds.jdbc.Driver
sql.url=jdbc:jtds:sqlserver://127.0.0.1:1433/business
sql.username=sa
sql.password=sa
cpool.minPoolSize=1
cpool.maxPoolSize=5
cpool.maxIdleTime=7200
cpool.maxIdleTimeExcessConnections=18000
cpool.acquireIncrement=1
applicationContext.xml文件配置两个数据源,两个SessionFactory分别对应相应的数据源
Spring公共配置
classpath*:/jdbc.properties
classpath*:/com/search/bean/*.hbm.xml
hibernate.dialect=org.hibernate.dialect.SQLServerDialect
hibernate.show_sql=true
hibernate.format_sql=false
hibernate.query.substitutions=true 1, false 0
hibernate.jdbc.batch_size=20
hibernate.cache.provider_class=org.hibernate.cache.EhCacheProvider
hibernate.cache.provider_configuration_file_resource_path=/ehcache-hibernate.xml
classpath*:/com/search/bean/*.hbm.xml
hibernate.dialect=org.hibernate.dialect.MySQLInnoDBDialect
hibernate.show_sql=true
hibernate.format_sql=false
hibernate.query.substitutions=true 1, false 0
hibernate.jdbc.batch_size=20
hibernate.cache.provider_class=org.hibernate.cache.EhCacheProvider
hibernate.cache.provider_configuration_file_resource_path=/ehcache-hibernate.xml
DAO类,提供两个sessionFactory,根据数据库关键字而连接不同的数据库
@Repository
public abstract class BaseDaoImpl implements BaseDao {
protected Logger log = LoggerFactory.getLogger(getClass());
public static final String SQLSERVER="sqlserver";
public static final String MYSQL="mysql";
protected static SessionFactory sessionFactory;
@Autowired
@Resource(name="sessionFactory")
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public Session getSession() {
return sessionFactory.getCurrentSession();
}
protected static SessionFactory sqlSessionFactory;
@Autowired
@Resource(name="sqlSessionFactory")
public void setSqlSessionFactory(SessionFactory sqlSessionFactory) {
this.sqlSessionFactory = sqlSessionFactory;
}
public Session getSqlSession(String database) {
if (database.equals(SQLSERVER)) {
return sqlSessionFactory.getCurrentSession();
}else if (database.equals(MYSQL)) {
return sessionFactory.getCurrentSession();
}
return null;
}
@SuppressWarnings("unchecked")
public List searchListBySQL(String sql,String database) {
return getSqlSession(database).createSQLQuery(sql).list();
}
}
DAO实现类
@Repository
public class ProductDaoImpl extends JeeCoreDaoImpl implements ProductDao {
public List getAllProducts(){
String hql="select * from table;
return searchListBySQL(hql,"mysql");
}
}
第二种方法
一、(在src下)写两个Hibernate.cfg.xml文件:
hbn-mysql.cfg.xml和hbn-sqlserver.cfg.xml
二、分别解析上面的两个.cfg.xml文件建两个sessionFactory,
三、使用session时哪个sessionFactory打开的session就能访问哪个数据库。
详细步骤:-----------------------------------------
一、(在src下)建两个Hibernate.cfg.xml文件
(1.)hbn-mysql.cfg.xml的内容:
Java代码
hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-2.0.dtd">
mysql
jdbc:mysql://localhost:3306/dataSource?characterEncoding=utf-8
com.mysql.jdbc.Driverroot
password
org.hibernate.dialect.MySQLDialect
resource="com/fourstar/starTransport/daomain/orderIdStatus.hbm.xml" />
resource="com/fourstar/starTransport/daomain/freightCompany.hbm.xml" />
(2.)hbn-sqlserver.cfg.xml的内容:
Java代码
hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
true
com.microsoft.sqlserver.jdbc.SQLServerDriver
jdbc:sqlserver://192.168.2.16:1433; DatabaseName=DB
root
password
2
org.hibernate.dialect.SQLServerDialect
resource="com/fourstar/starTransport/daomain/transactions.hbm.xml" />
resource="com/fourstar/starTransport/daomain/transactionDetail.hbm.xml" />
二、建一个类(HbnUtil)用于连接数据库(用于创建sessionFactory和openSession()静态方法)
Java代码
package com.fourstar.starTransport.dao.util;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HbnUtil {// 根据hibernate.cfg.xml创建一个静态sessionFactory
private static SessionFactory sf;
private static SessionFactory MSsf;
static {
sf = new Configuration().configure("/hbn-sqlserver.cfg.xml")
.buildSessionFactory();
MSsf = new Configuration().configure("/hbn-mysql.cfg.xml")
.buildSessionFactory();
}
/**根据DBName判断调用哪个sessionFactory的openSession()方法*/
public static Session getSessionByDB(String DBName) {
Session s = null;
if (DBName == "mysql") {
if (!MSsf.isClosed())
s = MSsf.openSession();
} else if (DBName == "sqlserver") {
if (!sf.isClosed())
s = sf.openSession();
} else {
System.out.println("错误的 DBName!");
}
return s;
}
/**根据DBName判断调用哪个sessionFactory的close()方法*/
public static void closeSessionFactoryByDB(String DBName) {
if (DBName == "mysql") {
if (!MSsf.isClosed()) {
MSsf.close();
}
} else if (DBName == "sqlserver") {
if (!sf.isClosed()) {
sf.close();
}
} else {
System.out.println("错误的 DBName!");
}
}
}
三、测试:使用getSessionByDB(String DBName)获得不同数据库的session
Java代码
package com.fourstar.starTransport.dao.util;
import org.hibernate.HibernateException;
import org.hibernate.Query;
import org.hibernate.Session;
public class Test1{
public static void main(String[] args) {
String DBName = "mysql";
// String DBName = "sqlservrer";
Session s = HbnUtil.getSessionByDB(DBName);
try {
String sql = " from freightCompany";
Query query = s.createQuery(sql);
} catch (HibernateException e) {
e.printStackTrace();
}finally{
s.close();
HbnUtil.closeSessionFactoryByDB(DBName);
}
}
}
————————————————————————————————————————
————————————————————————————————————————
做完这些之后我再介绍下hibernate连接两个数据库的原理:
——————————————————————————————————————
一、Hibernate访问数据库时加载的过程
对于大多数使用Hibernate的朋友来说,通常使用一下方式来获得Configuration实例: Configuration
configure = new Configuration().configure();
在Hibernate中,Configuration是hibernate的入口。在实例化一个Configuration的时候,Hibernate会自动在环境变量(classpath)里面查找Hibernate配置文件hibernate.properties。如果该文件存在,则将该文件的内容加载到一个Properties的实例GLOBAL_PROPERTIES里面,如果不存在,将打印信息
hibernate.properties not found;
接下来Hibernate将所有系统环境变量(System.getProperties())也添加到GLOBAL_PROPERTIES里面。如果配置文件hibernate.properties存在,系统还会进一步验证这个文件配置的有效性,对于一些已经不支持的配置参数,系统将打印出警告信息。
默认状态下configure()方法会自动在环境变量(classpath)下面寻找Hibernate配置文件hibernate.cfg.xml,如果该文件不存在,系统会打印如下信息并抛出HibernateException异常:
hibernate.cfg.xml not
found;如果该文件存在,configure()方法会首先访问<session-factory>,并获取该元素name的属性,如果name的属性非空,将用这个配置的值来覆盖hibernate.properties的hibernate.session_factory_name的配置的值,从这里我们可以看出,hibernate.cfg.xml里面的配置信息可以覆盖hibernate.properties的配置信息。
接下来configure()方法访问<session-factory>的子元素,首先将使用所有的<property>元素配置的信息来覆盖hibernate.properties里面对应的配置信息。
然后configure()会依次访问以下几个元素的内容
<mapping><jcs-class-cache><jcs-collection-cache><collection-cache>
其中<mapping>是必不可少的,必须通过配置<mapping>,configure()才能访问到我们定义的java对象和关系数据库表的映射文件(hbm.xml),例如:
<mapping resource="Cat.hbm.xml"/>
这样configure()方法利用各种资源就创建了一个Configuration实例。对于整个项目来说,如果用一个本地线程来存放这个Configuration实例,那么整个项目只需要实例化一次Configuration对象(注:Configuration实例很花费时间),也就提高了项目的效率。
以上了解了解析hibernate.cfg.xml映射文件使得configuration对象的实例化的方法new Configuration().configure()。如果我们需要连接两个数据库就需要解析两个hibernate映射文件去实例化两个configuration对象,我们使用方法new Configuration().configure(“hibernate文件路径”)。然后使用configuration对象的 buildSessionFactory()方法去创建session工厂。有了session工厂就可以生产数据库操作对象session了。
转载地址:https://blog.csdn.net/weixin_32050033/article/details/113424107 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关于作者
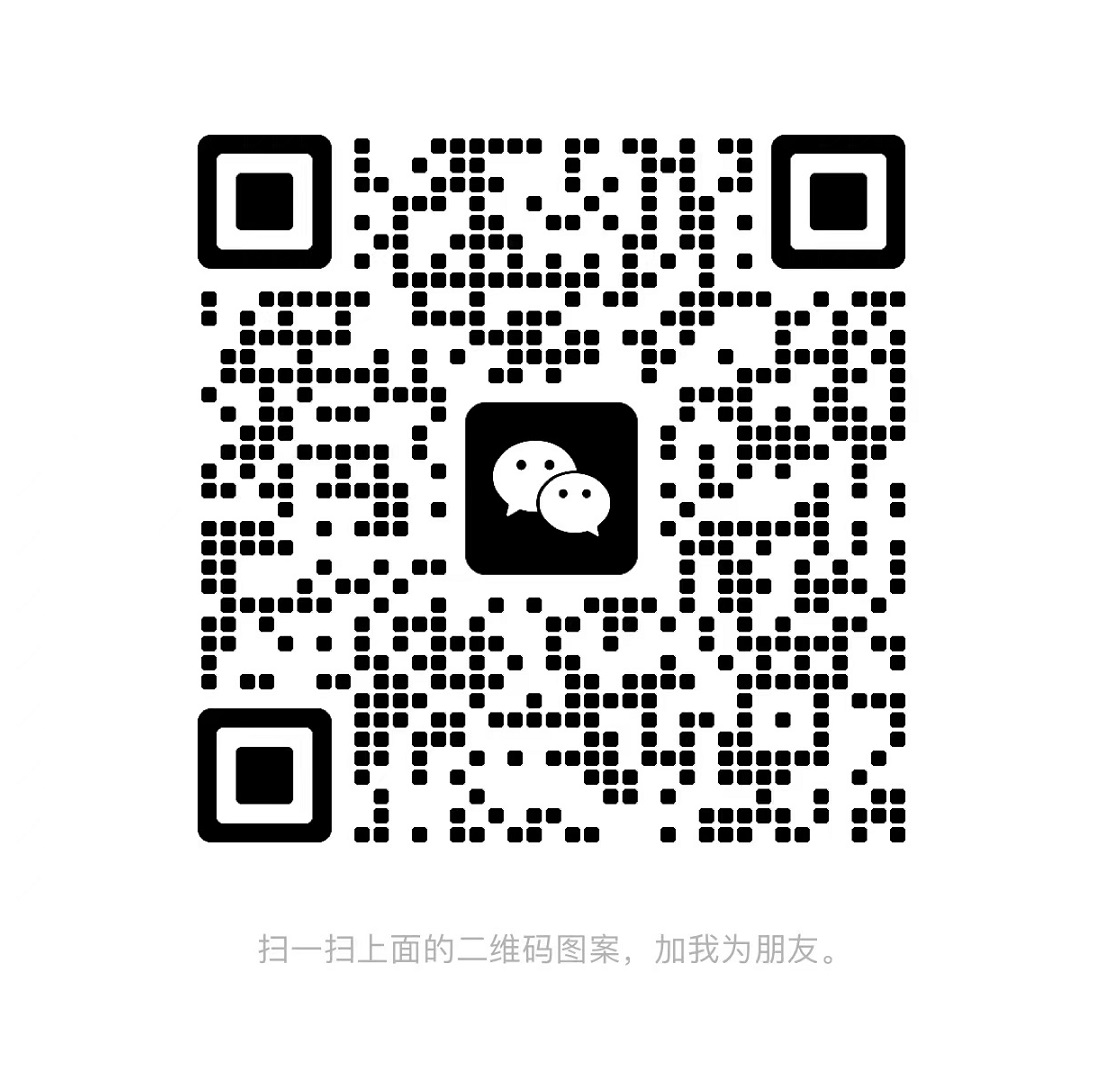