
本文共 4282 字,大约阅读时间需要 14 分钟。
itoa函数及atoi函数 2007-05-11 13:52 C语言提供了几个标准库函数,可以将任意类型(整型、长整型、浮点型等)的数字转换为字符串。以下是用itoa()函数将整数转 换为字符串的一个例子: # include <stdio.h> # include <stdlib.h> void main (void) { int num = 100; char str[25]; itoa(num, str, 10); printf("The number 'num' is %d and the string 'str' is %s. /n" , num, str); } itoa()函数有3个参数:第一个参数是要转换的数字,第二个参数是要写入转换结果的目标字符串,第三个参数是转移数字时所用 的基数。在上例中,转换基数为10。10:十进制;2:二进制... itoa并不是一个标准的C函数,它是Windows特有的,如果要写跨平台的程序,请用sprintf。 是Windows平台下扩展的,标准库中有sprintf,功能比这个更强,用法跟printf类似: char str[255]; sprintf(str, "%x", 100); //将100转为16进制表示的字符串。 下列函数可以将整数转换为字符串: ---------------------------------------------------------- 函数名 作 用 ---------------------------------------------------------- itoa() 将整型值转换为字符串 itoa() 将长整型值转换为字符串 ultoa() 将无符号长整型值转换为字符串 一 atoi 把字符串转换成整型数 例程序: #include <ctype.h> #include <stdio.h> int atoi (char s[]); int main(void ) { char s[100]; gets(s); printf("integer=%d/n",atoi(s)); return 0; } int atoi (char s[]) { int i,n,sign; for(i=0;isspace(s[i]);i++)//跳过空白符 ; sign=(s[i]=='-')?-1:1; if(s[i]=='+'||s[i]==' -')//跳过符号 i++; for(n=0;isdigit(s[i]);i++) n=10*n+(s[i]-'0');//将数字字符转换成整形数字 return sign *n; } 二 itoa 把一整数转换为字符串 例程序: #include <ctype.h> #include <stdio.h> void itoa (int n,char s[]); //atoi 函数:将s转换为整形数 int main(void ) { int n; char s[100]; printf("Input n:/n"); scanf("%d",&n); printf("the string : /n"); itoa (n,s); return 0; } void itoa (int n,char s[]) { int i,j,sign; if((sign=n)<0)//记录符号 n=-n;//使n成为正数 i=0; do{ s[i++]=n%10+'0';//取下一个数字 }while ((n/=10)>0);//删除该数字 if(sign<0) s[i++]='-'; s[i]='/0'; for(j=i;j>=0;j--)//生成的数字是逆序的,所以要逆序输出 printf("%c",s[j]); } 是int 转string类型的一个函数 msdn上是这么写的 _itoa, _i64toa, _ui64toa, _itow, _i64tow, _ui64tow Convert an integer to a string. char *_itoa( int value, char *string, int radix ); char *_i64toa( __int64 value, char *string, int radix ); char * _ui64toa( unsigned _int64 value, char *string, int radix ); wchar_t * _itow( int value, wchar_t *string, int radix ); wchar_t * _i64tow( __int64 value, wchar_t *string, int radix ); wchar_t * _ui64tow( unsigned __int64 value, wchar_t *string, int radix ); Routine Required Header Compatibility _itoa <stdlib.h> Win 95, Win NT _i64toa <stdlib.h> Win 95, Win NT _ui64toa <stdlib.h> Win 95, Win NT _itow <stdlib.h> Win 95, Win NT _i64tow <stdlib.h> Win 95, Win NT _ui64tow <stdlib.h> Win 95, Win NT For additional compatibility information, see Compatibility in the Introduction. Libraries LIBC.LIB Single thread static library, retail version LIBCMT.LIB Multithread static library, retail version MSVCRT.LIB Import library for MSVCRT.DLL, retail version Return Value Each of these functions returns a pointer to string. There is no error return. Parameters value Number to be converted string String result radix Base of value; must be in the range 2 – 36 Remarks The _itoa, _i64toa, and _ui64toa function convert the digits of the given value argument to a null-terminated character string and stores the result (up to 33 bytes) in string. If radix equals 10 and value is negative, the first character of the stored string is the minus sign ( – ). _itow, _i64tow, and _ui64tow are wide-character versions of _itoa, _i64toa, and _ui64toa respectively. Generic-Text Routine Mappings TCHAR.H Routine _UNICODE & _MBCS Not Defined _MBCS Defined _UNICODE Defined _itot _itoa _itoa _itow Example /* ITOA.C: This program converts integers of various * sizes to strings in various radixes. */ #include <stdlib.h> #include <stdio.h> void main( void ) { char buffer[20]; int i = 3445; long l = -344115L; unsigned long ul = 1234567890UL; _itoa( i, buffer, 10 ); printf( "String of integer %d (radix 10): %s/n", i, buffer ); _itoa( i, buffer, 16 ); printf( "String of integer %d (radix 16): 0x%s/n", i, buffer ); _itoa( i, buffer, 2 ); printf( "String of integer %d (radix 2): %s/n", i, buffer ); _ltoa( l, buffer, 16 ); printf( "String of long int %ld (radix 16): 0x%s/n", l, buffer ); _ultoa( ul, buffer, 16 ); printf( "String of unsigned long %lu (radix 16): 0x%s/n", ul, buffer ); } Output String of integer 3445 (radix 10): 3445 String of integer 3445 (radix 16): 0xd75 String of integer 3445 (radix 2): 110101110101 String of long int -344115 (radix 16): 0xfffabfcd String of unsigned long 1234567890 (radix 16): 0x499602d2 Data Conversion Routines See Also _ltoa, _ultoa
转载地址:https://blog.csdn.net/sayigood/article/details/5119884 如侵犯您的版权,请留言回复原文章的地址,我们会给您删除此文章,给您带来不便请您谅解!
发表评论
最新留言
关于作者
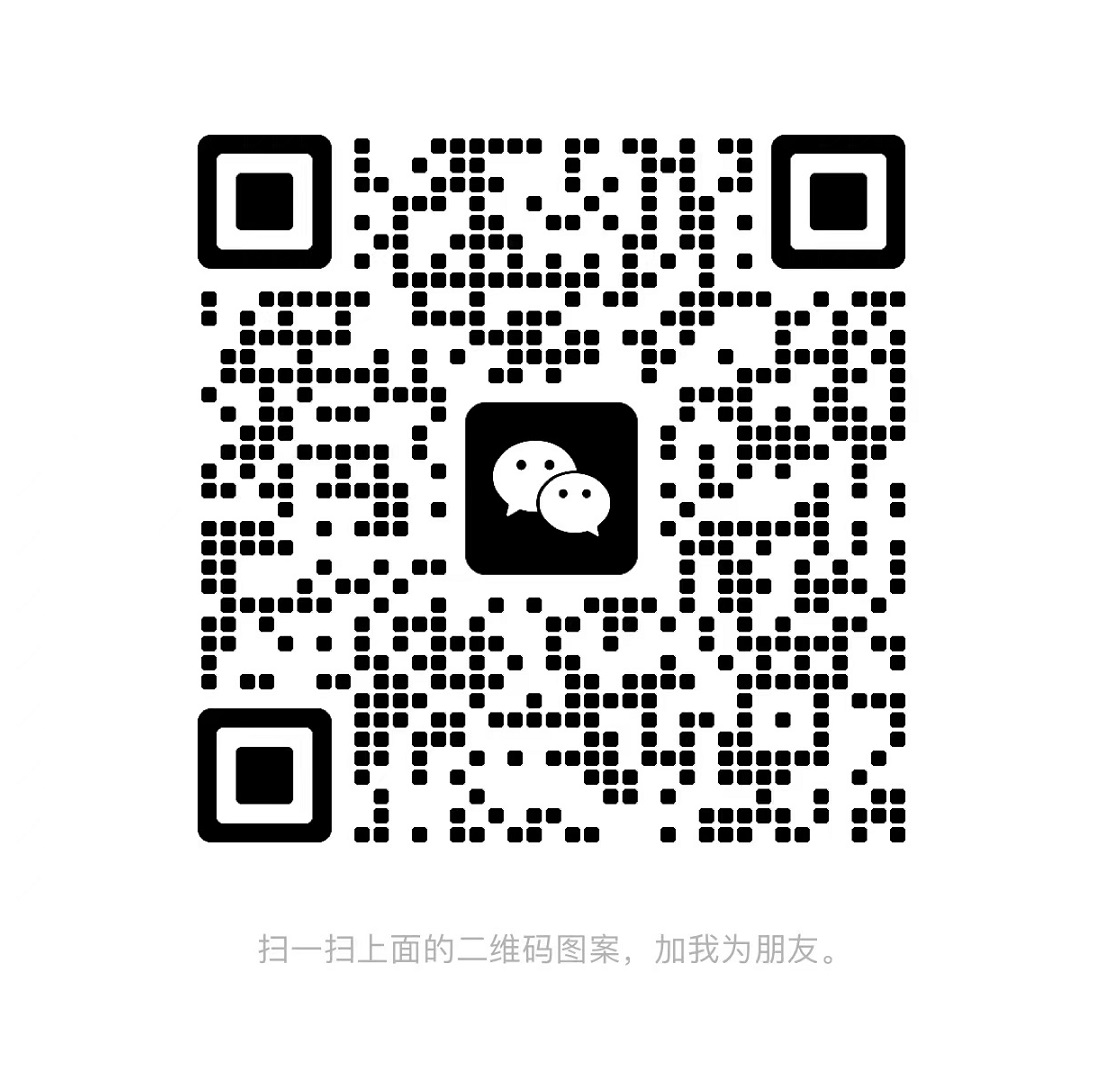